How to Initialize Static Variables in C++ Class
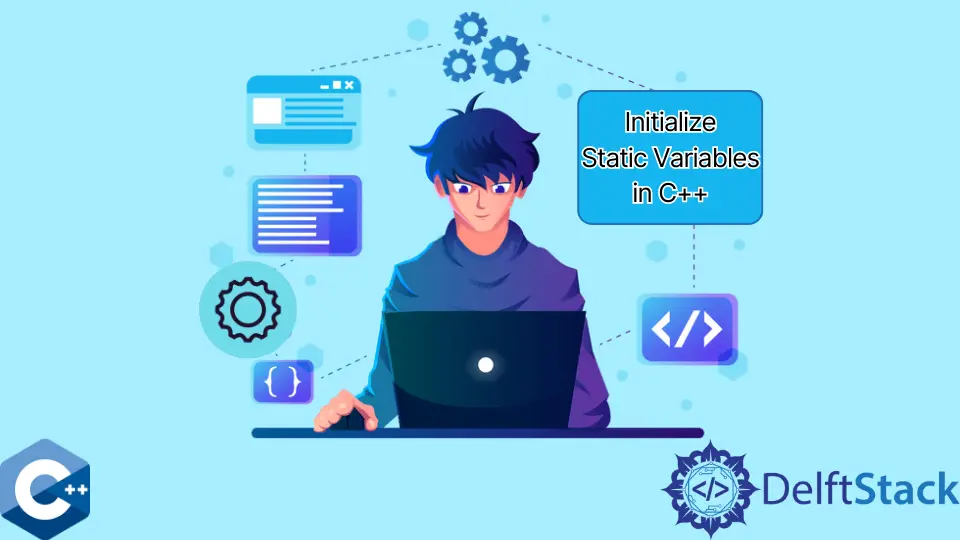
We will learn in this short article how to initialize static variables in C++.
Initialize Static Variables in C++
The initialization of static variables in a C++ class is the process of assigning values to the static variables.
All class objects have access to a static member. If no other initialization is present, all static data is initialized to zero when the first object is created.
Although we cannot include it in the class definition, we can initialize it outside the class by redeclaring the static variable and determining its class affiliation using the scope resolution
operator. Usually, The initialization can be done in two ways.
- Implicitly, by defining them as constants.
- Explicitly by using the “static” keyword and assigning values.
We should use implicit initialization only when we are sure that all static variables have been initialized beforehand. Otherwise, we should use explicit initialization with the static
keyword.
Code Example:
#include <iostream>
using namespace std;
class Sam {
public:
static int demo;
Sam(int x = 1, int y = 2, int z = 3) {
X = x;
Y = y;
Z = z;
}
private:
double X;
double Y;
double Z;
};
int Sam::demo = 7;
int main(void) {
Sam sam1(6, 7, 8);
cout << "Demo: " << Sam::demo << endl;
return 0;
}
Output:
Demo: 7
Difference Between Constant and Static Variables
In C++, a variable is a name given to a storage location in the computer’s memory that can store different data types, such as numbers and text. These names are called identifiers
.
The data stored in the variable may change over time. This type of variable is called dynamic
or dynamic-typed
.
The other variable type is called constant
or constant-typed
. The data stored in this kind of variable never changes over time, and constants are typically used to define an object’s properties and parameters at the beginning of a program or function.
Constants are declared with the const
keyword and can only be initialized at declaration time or inside a function. The static
keyword creates the variable only once, and all functions within the same file can access the variable.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook