Concept of C++ Include Path
- C++ Include Path Directory in Visual Studio IDE
- Ways to Include Paths in C++
- How Preprocessor Searches the Paths
-
Difference Between
#include ""
and#include <>
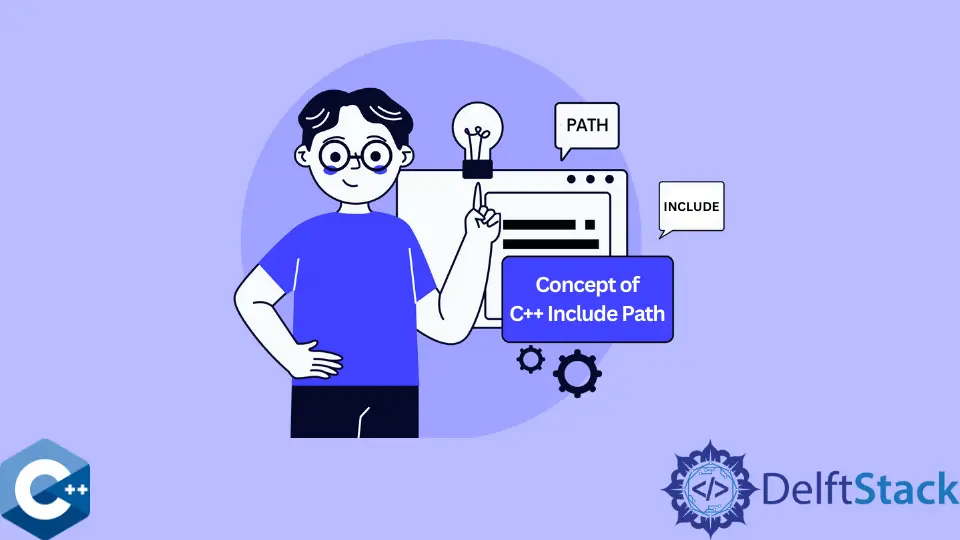
Include paths are used to tell the compiler where to look for header files. The compiler will search in the directories specified by these paths until it finds a header file with a matching name.
C++ Include Path Directory in Visual Studio IDE
The include path is specified as an argument to the compiler, usually on the command line. Usually, the include path directory is located at the following location:
C:\Program Files\Microsoft Visual Studio 14.0\VC
To add this directory, you need to follow the below-mentioned steps.
-
Open up the Visual Studio IDE.
-
On the Build tab, select Configuration Manager.
-
Select your compiler and click on Open.
-
Click on VC++ Directories.
-
Click on Include Files and click Add New Path.
-
Type in the path of your desired folder or click on the button to browse for it.
-
Once you have selected this directory, click Add. This new location will be added to the list of directories under the Include Directories tab.
Ways to Include Paths in C++
There are several ways to include the paths in C++. The most popular way is to use the preprocessor directive.
The preprocessor directive is a keyword that tells the compiler to run some code before compiling the actual program. It can be used to define constants and macros or include other files in your program.
Another way to include paths in C++ is by using a macro. Macros are another type of function that can be used in place of an identifier or any other sequence of characters you want to represent as a single token.
These functions are typically used for simple text replacements, such as when you have a long line and want to replace it with something shorter, like hello world
instead of Hello World
.
How Preprocessor Searches the Paths
The preprocessor searches the C++ include paths directory for the header files, then includes them in the compilation process. The search order is from left to right, top to bottom.
Header files are included by default if found in any of the directories listed in the include paths directory. If a header file isn’t found in any of those directories, it will be searched in the current directory and then in all parent directories until it is found or there are no more parent directories to search through.
An error message will be given if a header file cannot be found, and compilation will fail unless you specify it.
When you use double quotation marks to enclose a complete, unequivocal path specification for the included file, the preprocessor searches only that path specification and neglects the usual directories.
Difference Between #include ""
and #include <>
#include ""
is for header files defined by the programmer. If a programmer has created their own header file, the name of the header file should be enclosed in quotation marks.
On the other hand, #include <>
is used to include predefined header files. If the header file is predefined, put the name of the header file in angular parentheses.
Let’s discuss some of the examples.
#include <stdio.h>
#include <iostream>
#include "adil.h"
These are some examples to include path files in C++.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook