C++ Helper Function in Class
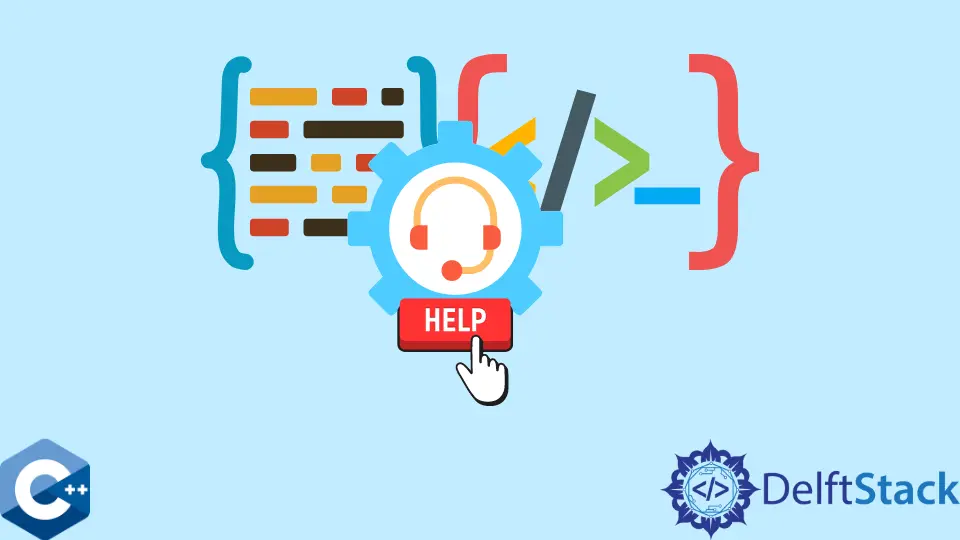
This tutorial demonstrates how to implement a helper function in a class in C++.
C++ Helper Function in Class
A helper function is a function that’s not instantiated by the end-users but provides a useful functionality used within another class internally. If the function isn’t a member of the class but some other class, the C++ program will create a helper function to print or use the data for other purposes.
A helper function is usually supplied by the writer of the class, which doesn’t need to directly access the representation of the class. Let’s try an example in C++ which implements the helper function.
#include <iostream>
#include <vector>
template <typename Delftstack>
void print(const std::vector<Delftstack>& DemoVec) {
typename std::vector<Delftstack>::const_iterator x;
for (x = DemoVec.begin(); x != DemoVec.end(); x++) std::cout << *x << " ";
std::cout << std::endl;
}
int main() {
std::vector<int> vector1, vector2;
vector1.assign(7, 3);
vector2.assign(7, 6);
std::cout << "Vector 1 : ";
print(vector1);
std::cout << "Vector 2 : ";
print(vector2);
if (vector1 == vector2)
std::cout << "vector 1 and Vector 2 are lexicographically equal!"
<< std::endl;
else
std::cout << "vector 1 and vector 2 are lexicographically not equal!"
<< std::endl;
if (vector1 > vector2)
std::cout << "vector 1 is lexicographically greater than vector 2!"
<< std::endl;
else
std::cout << "vector 2 is lexicographically greater than vector 1!"
<< std::endl;
}
The code above uses the helper function in class to compare two vectors. The output for this code when the two vectors are not equal is below.
Vector 1 : 3 3 3 3 3 3 3
Vector 2 : 6 6 6 6 6 6 6
vector 1 and vector 2 are lexicographically not equal !
vector 2 is lexicographically greater than vector 1 !
And when the vectors are equal which means we change the values of vectors from:
vector1.assign(7, 3);
vector2.assign(7, 6);
To:
vector1.assign(7, 3);
vector2.assign(7, 3);
In this case, the output will be:
Vector 1 : 3 3 3 3 3 3 3
Vector 2 : 3 3 3 3 3 3 3
vector 1 and Vector 2 are lexicographically equal!
vector 2 is lexicographically greater than vector 1!
We can ignore the last line because it comes from the else
condition; it is set for the nonequality scenario. For example, if the values are:
vector1.assign(7, 6);
vector2.assign(7, 3);
The output for these values will be:
Vector 1 : 6 6 6 6 6 6 6
Vector 2 : 3 3 3 3 3 3 3
vector 1 and vector 2 are lexicographically not equal!
vector 1 is lexicographically greater than vector 2!
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook