How to Get Mouse Position in C++
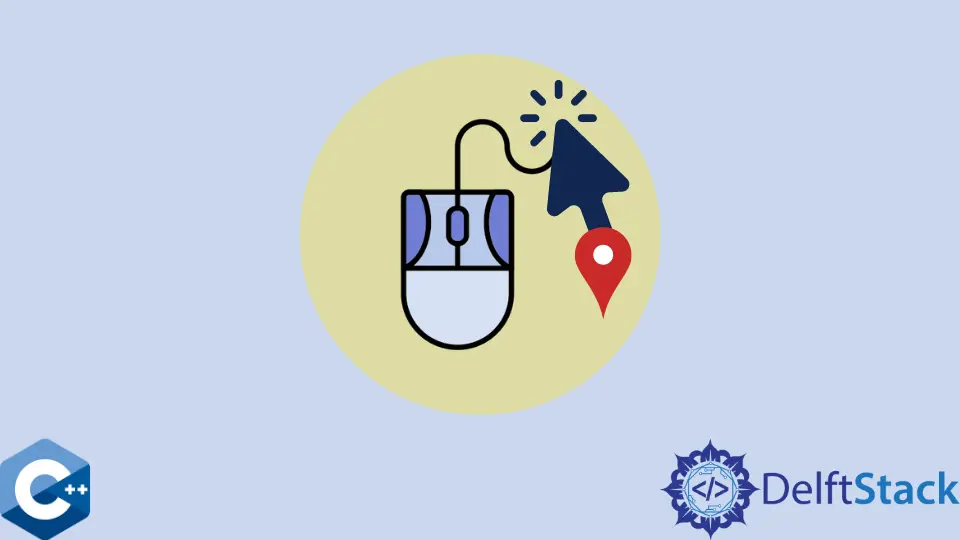
This tutorial teaches how to get the mouse position in C++.
Get Mouse Position in C++
C++ provides a method, GetCursorPos
, to get the x and y position of the mouse cursor. The method is very easy to use; we have to declare a Point
and then pass it to the method, and the method will return the x and y points of the mouse position.
Let’s try an example using the GetCursorPos()
method to get the mouse position in C++.
#include <windows.h>
#include <iostream>
using namespace std;
int main() {
POINT MousePoint;
if (GetCursorPos(&MousePoint)) {
cout << MousePoint.x << "," << MousePoint.y << "\n";
}
return 0;
}
The above code will try to get the x and y points of the mouse cursor by analyzing the whole screen. See the output:
424,266
Getting the position of the mouse is usually required when we are working on developing a game or application, and the GameMaker:Studio
will not allow us to access the mouse coordinates outside the given screen; in this case, we can use the code below to get the coordinates of the mouse.
#include <WinUser.h>
#include <Windows.h>
#define EXPORT extern "C" _declspec(dllexport)
EXPORT double GetMouse(double mode) {
POINT MousePoint;
GetCursorPos(&MousePoint);
if (mode == 0) {
double Mouse_X = MousePoint.x;
return Mouse_X;
} else {
double Mouse_Y = MousePoint.y;
return Mouse_Y;
}
}
The above code uses WinUser.h
, a child header of Windows, and it helps us get the mouse coordinates inside and outside the screen. See the output:
630,368
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook