How to Get Class Name in C++
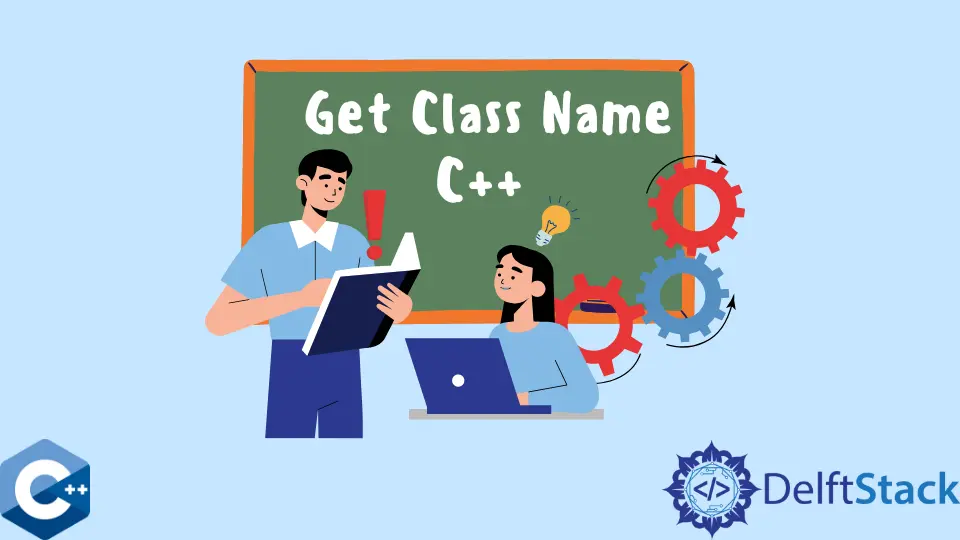
In this article, we shall learn how to get the class name using the C++ programming language.
Overview of Class in C++
In C++, everything is connected to classes and objects, each with its characteristics and procedures.
A class is a user-defined data type, which means you can create your types. It serves as an object constructor, sometimes known as a guideline,
to generate new objects.
Get Class Name in C++
Let’s begin by importing the libraries so we can access all the methods necessary for our application.
#include <iostream>
We’ll create three classes and give them the names getClassNameSaad
, getClassName,
and className
. In the following steps, we will get access to the names of these classes.
class getClassNameSaad {};
class getClassName {};
class className {};
Inside the main()
function, we need to make an instance of the classes we have just created.
int main() {
getClassNameSaad a_variable;
getClassName b_variable;
className c_variable;
}
Now that we are through with the previous step, we need to output the names of the classes. We will utilize the typeid()
method, pass it the parameters a_variable,
b_variable,
and c_variable,
and then access the name()
function of the class.
std::cout << typeid(a_variable).name() << "\n";
std::cout << typeid(b_variable).name() << "\n";
std::cout << typeid(c_variable).name() << "\n";
Complete Source Code:
#include <iostream>
class getClassNameSaad {};
class getClassName {};
class className {};
int main() {
getClassNameSaad a_variable;
getClassName b_variable;
className c_variable;
std::cout << typeid(a_variable).name() << "\n";
std::cout << typeid(b_variable).name() << "\n";
std::cout << typeid(c_variable).name() << "\n";
return 0;
}
Output:
16getClassNameSaad
12getClassName
9className
The integer numbers in the output before each class’s name represent the total number of letters included in that class’s name.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn