Functors in C++
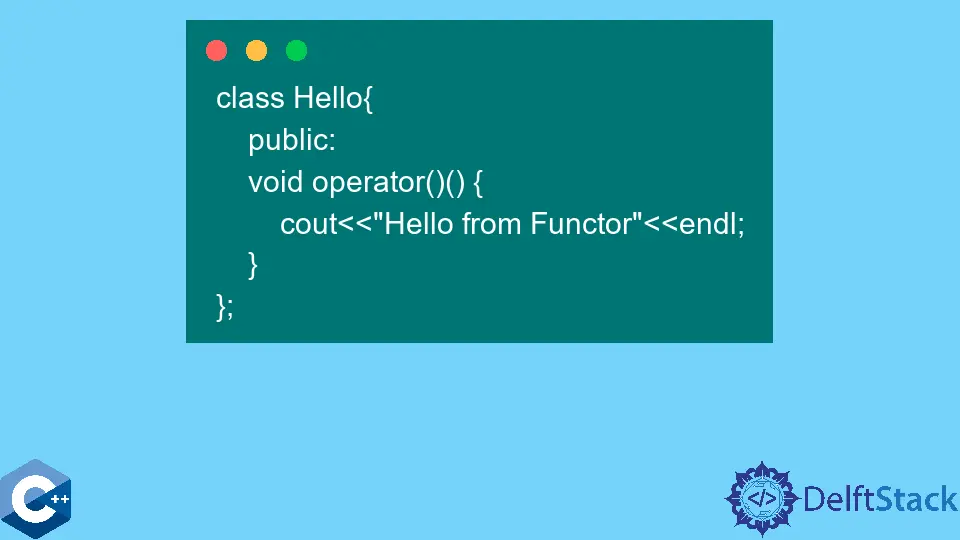
This trivial tutorial introduces functors and their use in the C++ programming language. First, this article will briefly introduce functors and then move toward its syntax and usability.
Introduction to Functors in C++
Functors are the class objects that can behave like functions. There are numerous functors available in the C++ Standard Template Library (STL), and you can make your own also.
The main advantage of functors is that although they are not functions, they can still save the state as they are class objects.
Functors are made by overloading the operator ()
in any class so that the object of that class can be called, as we call a simple function.
Let’s look at the example below to have a clear understanding.
class Hello {
public:
void operator()() { cout << "Hello from Functor" << endl; }
};
Now to use this, we will create an object of the Hello
class and call it like this:
int main() {
Hello hello;
hello();
return 0;
}
Output:
Hello from Functor
We can see from the output that the function call operator ()
is called when we use the object with the operator.
Functors with Parameters in C++
Functors can also be defined as having some parameters and return-type, just like functions. We can amend the previous functor to include the parameter in it.
class Hello {
public:
void operator()(string name) {
cout << "Hello " << name << " from Functor" << endl;
}
};
To call this, we need to pass the parameter as well.
int main() {
Hello hello;
hello("david");
return 0;
}
Output:
Hello david from Functor
Pre-defined Functors in C++
Numerous functors are included in functional
header files which are packaged in the C++ Standard Template Library. Some of them are discussed here.
Functor name | Description |
---|---|
plus |
Calculates the sum of two numbers passed as parameters. |
minus |
Calculates the difference between two numbers passed as parameters. |
multiplies |
Calculates the product of two numbers passed as parameters. |
divides |
Calculates the division result of two numbers passed as parameters. |
equal_to |
Checks if the two parameters passed are equal or not. |
not_equal_to |
Checks if the two parameters passed are not equal. |
greater |
Checks if the first parameter is greater than the second. |
less |
Checks if the first parameter is less than the second. |
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn