C++ Escape Characters
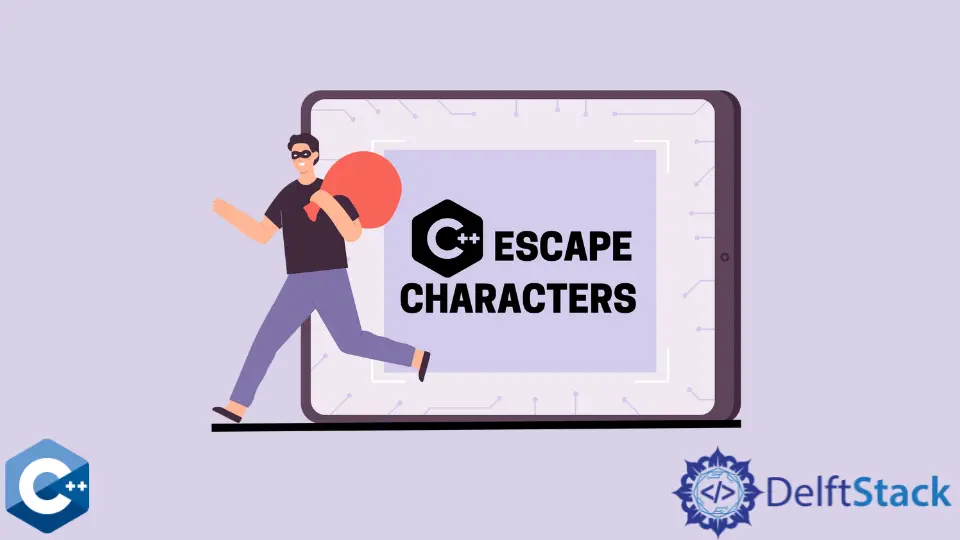
This tutorial will first discuss the escape characters in C and C++. The only caveat here is that using hexadecimal codes assumes having an ASCII-compatible character encoding.
Control Characters in C++
\a |
\x07 |
alert (bell), which produces the bell sound on most computers |
\b |
\x08 |
backspace |
\t |
\x09 |
horizontal tab |
\n |
\x0A |
newline |
\v |
\x0B |
vertical tab |
\f |
\x0C |
form feed |
\r |
\x0D |
carriage return (the Enter key on most computers) |
\e |
\x1B |
Escape (This is non-standard. You might not always find this) |
Punctuation Characters in C++
\"
is the quotation mark (double apostrophe). Also, note that the backslash will not be needed if the character is written as '"'
.
\'
= apostrophe. Again, the backslash will not be required in a specific case, and this time it is "'"
.
\?
= Question mark (However, it is only used in specific cases, such as avoiding trigraphs. In C, Trigraphs are groups of 3 characters that have a specific meaning when used together.
For example, the ??=
trigraph is equivalent to the #
punctuation mark. More information can be found in this documentation.
\\
= Backslash. For when you need to write a backslash.
Numeric Character References in C++
\ +
(plus sign) any 3 octal digits.
\x +
plus any number of hexadecimal digits. Note that the compiler reads the hexadecimal digits until they encounter the first non-hex digit, and after encountering the first non-hex digit, it stops reading digits for the current character.
\u +
plus 4 hex digits (Unicode BMP, added in C++11).
\U +
plus 8 hex digits (Unicode astral planes, added in C++11).
\0
= \00
= \000
= Null character.
Another special case is \e
, which is not standard in C or C++ but would be used by some compilers to represent \x1B
.
If you wish to have something non-standard, for example, to construct a string with a character 0
, followed by a null character \0
, followed by the character 0
once again, then you have a couple of options.
String Concatenation Syntax:
std::string(
"0\0"
"0",
3);
Initializer List Syntax:
std::string{'0', 0, '0'};
Using the latter option over the first option is generally preferred, as you avoid specifying sizes and can also avoid using the escape characters altogether.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn