How to Handle Errors in C++
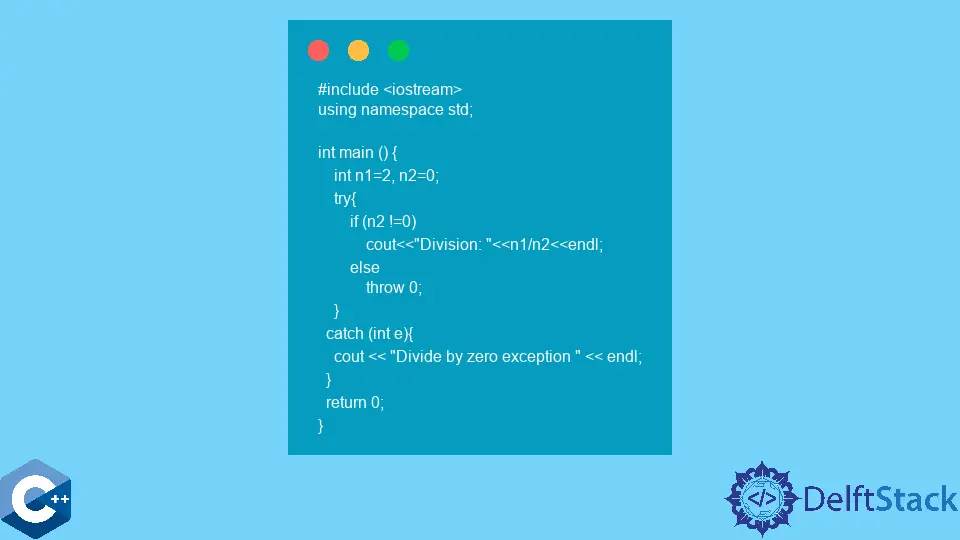
This trivial guide discusses error and exception handling in C++.
Error Handling in C++
There can be multiple types of errors in your program in C++. Some errors require an intimation to the user.
You must give the user a proper error message and exit the program.
For handling errors, there can be several different ways that can be adopted. Some of these are:
- Giving some hard-coded error messages to the user.
- Saving some expected error messages in some variables and displaying those variables.
- Doing exception handling.
The first method of giving some hard-coded error messages is very easy and simple. Wherever there is a chance of error in the code, display some error message and exit the code.
For example, in case of invalid login or password entry, you can display a message like this:
cout << "You have entered an incorrect username or password" << endl;
cout << "Try again!" << endl;
The second method is to make some strings for the possible error message and display those variables wherever required.
const string INVALID_LOGIN_ERROR_MESSAGE = "Invalid Username or Password";
// later in the code
cout << INVALID_LOGIN_ERROR_MESSAGE << endl;
Such types of strings can be declared in a separate header file like ErrorMessages.h
and include that header file in your main file. The advantage of such string literals is to prevent the duplication of code.
Anytime you want to change the error message, you need to change it in one place (i.e., the declaration part).
Exception Handling in C++
Some runtime errors can’t be prevented even with expert programming skills. These are exceptions, and we do exception handling for such types of errors.
For example, we may try accessing some memory from the machine which is not available, or an API user may pass illegal arguments to the function. Such types of errors are handled using exception handling.
To use exception handling in your program, we enclose the block of code that expects some error in a try
block. So whenever there is an exception, control is automatically shifted to the associated catch
block that shows some meaningful error message before terminating the program.
Consider the example below.
#include <iostream>
using namespace std;
int main() {
int n1 = 2, n2 = 0;
try {
if (n2 != 0)
cout << "Division: " << n1 / n2 << endl;
else
throw 0;
} catch (int e) {
cout << "Divide by zero exception " << endl;
}
return 0;
}
The output of the above code will be: