How to Empty Constructors in C++
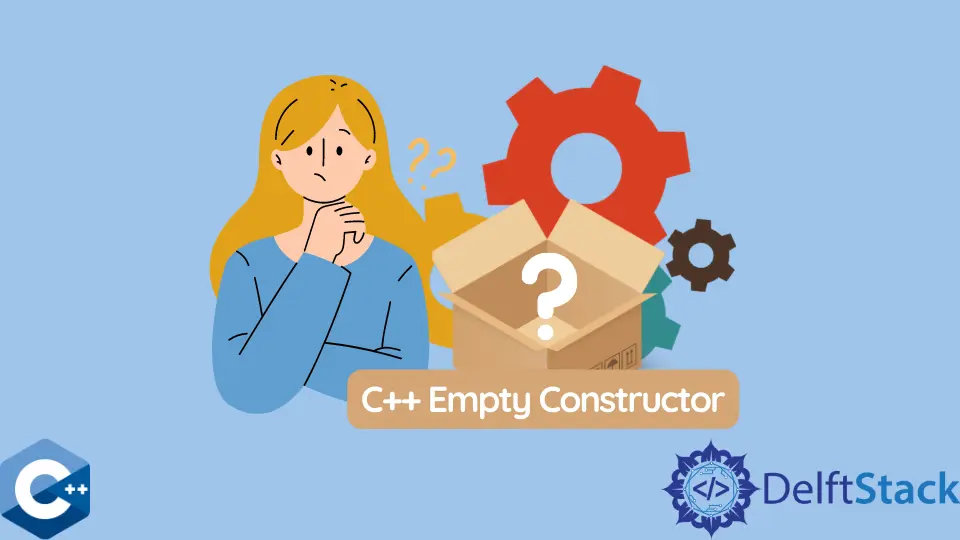
Empty constructors in C++ are a special type of constructor that does nothing. The compiler knows that there is no code to execute, so it will not generate any executable code for the constructor.
Empty Constructors in C++
The primary use of an empty constructor is when a class needs to have a default initialization. This is typically done by assigning values to all the class fields, but this can be tedious and error-prone if there are many fields or if the fields have complicated types.
It can also be difficult for programmers new to C++ because they might not know what initializing every field does or how to do it correctly. Using an empty constructor can avoid these problems and allow for some more complex initialization logic without writing out every step explicitly.
They are also called default constructors because they are the default constructor for a class if no other constructors are specified.
In some cases, empty constructors can be used to implement copy-constructor or move-constructor, but this is not always possible.
Use Empty Constructors in C++
Empty constructors are used when a class has only one constructor, and the default constructor is insufficient to initialize all member variables. Empty constructors can also be used to simplify the initialization of member variables.
We use the following steps to use the empty constructors in C++.
- Define an empty constructor that takes no parameters, initializing all member variables with default values.
- Add this empty constructor before the other constructors in the class definition to become the default one.
- Remove any code from other constructors if they are not needed for initialization.
Let’s discuss an example of the empty constructor.
#include <iostream>
using namespace std;
class Name {
private:
double Number;
public:
// Empty Constructor
Name() {
Number = 956;
cout << "Muhammad Adil" << endl;
cout << "Obtained Marks = " << Number << endl;
}
};
int main() {
Name name1;
return 0;
}
Here, the name
is the empty constructor.
Click here to check the working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook