Dangling Pointers in C++
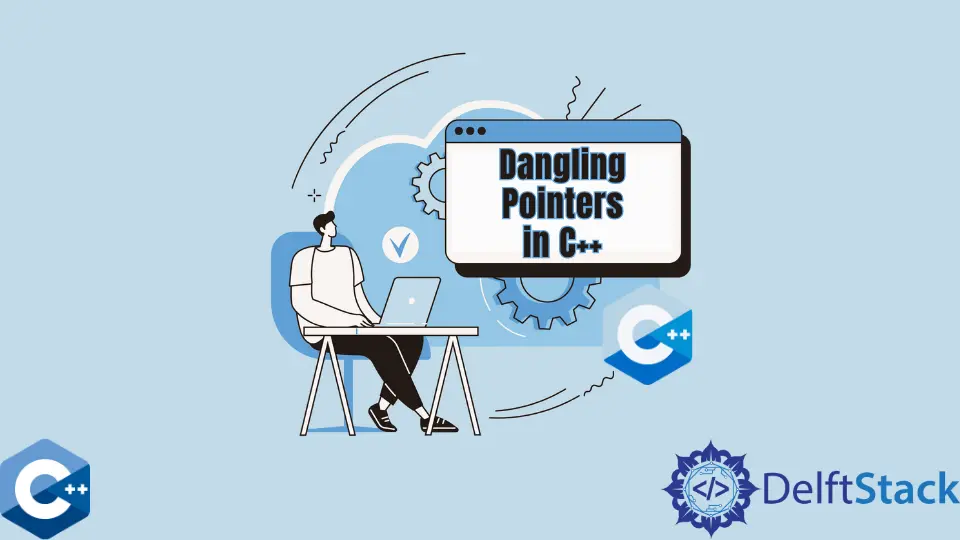
This article will discuss what a dangling pointer is.
Dangling Pointers in C++
A dangling pointer is a pointer (reference) to an object that no longer exists. When the object gets deleted, the pointer points to the memory address where it used to be.
The dangling pointer does not point to valid data and is useless.
A dangling pointer can occur when a programmer forgets to delete a memory space that was previously allocated for an object. This can happen when a programmer has allocated too much memory and doesn’t have enough space left over for all the objects they need, so they allocate more space and forget about the first allocation.
This error can cause a program to crash when two pointers are pointing to the same memory address, and one of them tries to access it.
The dangling pointers are also called memory leaks because they leak memory from the heap. A heap is a memory region where dynamic data resides and can be allocated or deallocated on demand by the programmer.
Simple Example of Dangling Pointers in C++
Dangling pointers are dangerous because they can cause all sorts of unpredictable behavior.
A typical example will be if the dangling pointer points to an area of memory where another piece of data has been placed over time. An example is a value in a list that changed after the dangling pointer was created.
If this happens and the dangling pointer is dereferenced, it will access this new value instead and cause unpredictable behavior.
The dangling pointer problem can often be avoided using smart pointers instead of raw pointers. A smart pointer is a class that provides the same interface as a raw pointer but also automatically deletes the pointed-to object when the smart pointer goes out of scope and releases any resources it uses.
Let’s discuss an example code of dangling pointers.
#include <iostream>
using namespace std;
int main() {
int *pointer = new int;
*pointer = 90;
delete pointer;
cout << pointer << endl;
}
As you have seen code above, we allocated the dynamic memory location to the pointer variable and stored 90
in it.
In the next line, we deleted the dynamic memory using the delete
operator, but the pointer
variable still points to the dynamic memory. This is what a Dangling Pointer is.
Click here to check the working of the above code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook