How to Create Directory in C++
-
Use the
mkdir()
Function to Create Directories in C++ -
Use the
std::filesystem::create_directory
Function to Create a Directory in C++ -
Use the
std::filesystem::create_directories
Function to Create a Directory in C++ -
Use
mkdir
System Calls in Linux/Unix on the GCC/G++ Compiler - Conclusion
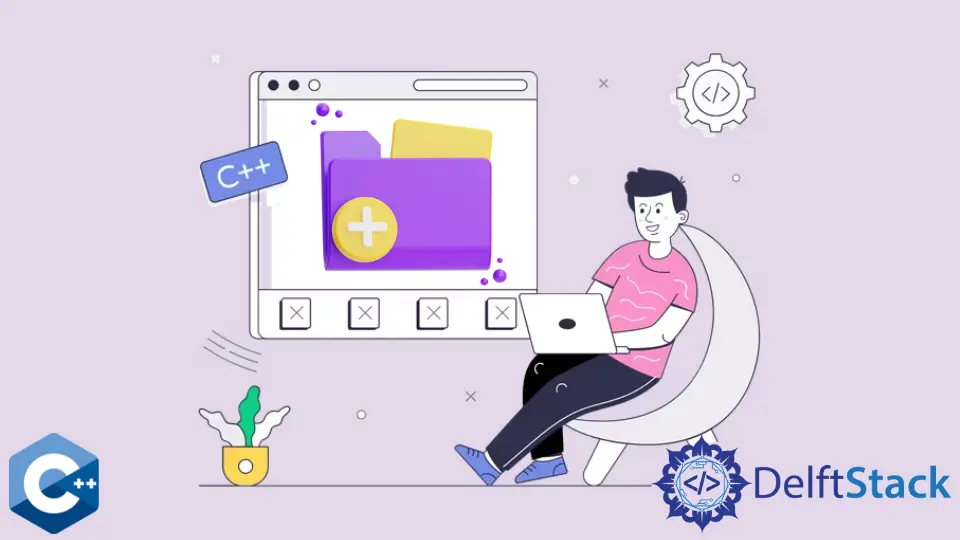
In the world of C++ programming, managing files and directories is a common task.
Creating directories, in particular, is essential for organizing and storing data efficiently. Whether you are developing a file management system, a data storage application, or simply need to organize your project files, knowing how to create directories in C++ is a fundamental skill.
In this article, we will explore various methods for creating directories in C++. We will cover the standard library functions, platform-specific methods, and third-party libraries.
By the end of this article, you will have a comprehensive understanding of the available options and be able to choose the best method for your specific needs.
Use the mkdir()
Function to Create Directories in C++
The mkdir()
function is utilized to create directories in C/C++. We can specify the filename
as it serves as an argument to this function.
Syntax:
#include <sys/stat.h>
int mkdir(const char *path, mode_t mode);
Parameters:
path
: A new directory with thepath
name is specified with the help of this parameter.mode
: It dictates the permissions that are to be given to the specified directory.
A shell command by the same name as this function also exists, which can help in creating a directory in Unix systems. However, our focus here will be on the systems powered by the Windows operating system for this particular method.
For Linux/Unix, we will discuss another method in the last part of the article.
Consider the following code:
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
#include <cstdio>
#include <cstdlib>
#include <iostream>
int main() {
int check;
const char* dirname = "delftstack";
check = mkdir(dirname, 0777);
if (!check) {
std::cout << "Directory created.\n";
} else {
std::cerr << "Unable to create directory.\n";
return 1;
}
system("ls");
return 0;
}
The above code makes use of the mkdir()
function to create a directory with the name "delftstack"
and displays all the directories.
Output:
As we see in the output above, the process of directory creation is a success, and therefore, the words "Directory created."
are displayed on the screen, along with a list of all the directories in the given path.
Use the std::filesystem::create_directory
Function to Create a Directory in C++
Since the C++17 version, the standard library provides the filesystem manipulation interface originally implemented as part of the Boost library. Note that all filesystem facilities are provided under the std::filesystem
namespace, which we are aliasing as fs
in the following examples.
Syntax:
bool create_directory(const std::filesystem::path& p);
The syntax takes in the path
and creates the directory.
The create_directory
function is used to create a new directory in the current working directory. The function takes one mandatory argument of type std::filesystem::path
, which can also be passed as a string object, and the proper initialization is done automatically.
Let’s take a look at an example:
#include <filesystem>
#include <iostream>
using std::cout;
using std::endl;
using std::string;
using std::system;
namespace fs = std::filesystem;
int main() {
string directory_name("tmp_delftstack");
fs::create_directory(directory_name)
? cout << "created directory - " << directory_name << endl
: cout << "create_directory() failed" << endl;
return EXIT_SUCCESS;
}
Here, we use the create_directory()
function of the std::filesystem
namespace to create a directory with the name "tmp_delftstack"
.
Output:
As we see in the output above, the function is successfully executed, and a directory is created. The name of the directory is "tmp_delftstack"
.
The successful function call can be checked by the return value of the create_directory
, which should be evaluated as true
.
Note that the created directory can be removed with the std::filesystem::remove
call, which takes the same directory path. The create_directory
optionally can take two-path arguments, the second of which must be an existing directory, and a newly created directory will copy the attributes of the former one.
#include <filesystem>
#include <iostream>
using std::cout;
using std::endl;
using std::string;
using std::system;
namespace fs = std::filesystem;
int main() {
string directory_name("temp_delftstack");
fs::create_directory(directory_name)
? cout << "created directory - " << directory_name << endl
: cout << "create_directory() failed" << endl;
fs::remove(directory_name);
cout << "removed directory - " << directory_name << endl;
return EXIT_SUCCESS;
}
Output:
created directory - tmp_delftstack
removed directory - tmp_delftstack
Use the std::filesystem::create_directories
Function to Create a Directory in C++
Another function we can use is std::filesystem::create_directories
, which can create multiple nested directories, all specified with a single path argument.
Syntax:
bool create_directories(const std::filesystem::path& p);
Similar to the method mentioned above, we specify the path
, and we are able to create multiple directories through this method.
In the following example code, we demonstrate this function to create four-level nested directories and then another one that shares the root directory with the first one.
Note, though, if the last level directory names already exist, the function returns false
.
#include <filesystem>
#include <iostream>
using std::cout;
using std::endl;
using std::string;
using std::system;
namespace fs = std::filesystem;
int main() {
auto ret = fs::create_directories("x_tmp/level1/level2/level3");
if (ret) {
cout << "created directory tree " << endl;
} else {
cout << "create_directories() failed" << endl;
}
auto ret2 = fs::create_directories("x_tmp/level1-2/level2-1");
if (ret2) {
cout << "created directory tree " << endl;
} else {
cout << "create_directories() failed" << endl;
}
return EXIT_SUCCESS;
}
The above code creates a directory tree with the help of the create_directories()
function taken from the std::filesystem
namespace.
Output:
The above output displays the message of the successful creation of a directory tree.
Similar to the create_directory
, this function also returns a Boolean value to denote a successful call. In case the removal is required std::filesystem::remove_all
function can be invoked with the root directory name; it will delete the whole tree.
#include <filesystem>
#include <iostream>
using std::cout;
using std::endl;
using std::string;
using std::system;
namespace fs = std::filesystem;
int main() {
bool ret = fs::create_directories("y_tmp/level1/level2/level3");
if (ret) {
cout << "created directory tree " << endl;
} else {
cout << "create_directories() failed" << endl;
}
bool ret2 = fs::create_directories("y_tmp/level1-2/level2-1");
if (ret2) {
cout << "created directory tree " << endl;
} else {
cout << "create_directories() failed" << endl;
}
fs::remove_all("y_tmp");
cout << "removed directory tree" << endl;
return EXIT_SUCCESS;
}
Output:
created directory tree
created directory tree
removed directory tree
After attempting to create directories, we remove the entire directory tree with the path "y_tmp"
using the remove_all
function from the filesystem
library and print a message indicating that the directory tree has been removed.
Use mkdir
System Calls in Linux/Unix on the GCC/G++ Compiler
In Linux/Unix-based systems, we use the GCC/G++ compiler along with the mkdir
system calls to create directories.
The mkdir
function, in this case, works with the same syntax as a generic C++ code due to the presence of the G++ compiler. It may have a different interpretation when implemented in a stock Linux programming environment.
Syntax:
#include <sys/stat.h>
int mkdir(const char *path, mode_t mode)
As specified in the first method of this article, this function takes in two arguments, path
and mode
.
We should also note that there is wide use of Linux/Unix systems for programming these days, and the process of carrying out this particular task on these systems is not unknown.
Consider the following code:
#include <bits/stdc++.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <iostream>
using namespace std;
int main()
{
// Creating a directory
if (mkdir("delftstack_file", 0777) == -1)
cerr << "Error : " << strerror(errno) << endl;
else
cout << "Directory created";
}
Similar to the first method explained in this article, we use the mkdir()
function, but this time in a GCC compiler on a Linux-based setup.
Output:
The output of this code displays the message "Directory created."
post the success of creating a directory.
Conclusion
Creating directories in C++ can be accomplished using various methods, each with its advantages and drawbacks. The best method for you depends on your specific project requirements:
- Standard Library (C++ 17 and later): This is the recommended approach for modern C++ code. It’s portable, safe, and easy to use. If your project uses C++17 or later, this method is the way to go.
- System Calls (Platform-Specific): While not recommended for cross-platform applications, using system calls can be suitable for platform-specific projects where portability is not a concern.
In general, it’s advisable to use the standard library when possible, as it provides a reliable and platform-independent solution for creating directories in C++. However, the choice ultimately depends on your project’s specific needs and constraints.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook