Cotangent Function in C++
- What is the Cotangent Function?
- Implementing Cotangent Function in C++
- Handling Edge Cases
- Conclusion
- FAQ
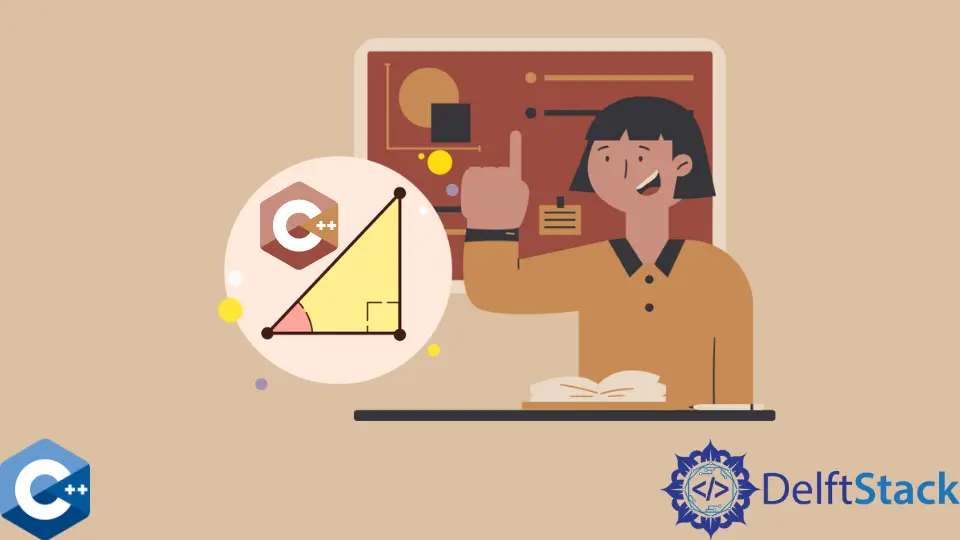
Understanding trigonometric functions is essential for various applications in mathematics, physics, and engineering. Among these functions, the cotangent function is particularly interesting. In C++, the cotangent function can be computed using the tangent function, as cot(x) is the reciprocal of tan(x).
This brief guide will walk you through the implementation of the cotangent function in C++, highlighting the necessary steps to solve trigonometric functions effectively. Whether you’re a beginner or looking to refine your skills, this article will provide you with clear examples and explanations to enhance your understanding of trigonometric functions in C++. Let’s dive in!
What is the Cotangent Function?
The cotangent function, denoted as cot(x), is one of the basic trigonometric functions. It is defined as the ratio of the adjacent side to the opposite side in a right triangle. In terms of sine and cosine, cotangent can be expressed as:
cot(x) = cos(x) / sin(x)
Alternatively, since cotangent is the reciprocal of tangent, we can also write:
cot(x) = 1 / tan(x)
In C++, we can leverage the <cmath>
library, which provides built-in functions for sine, cosine, and tangent calculations. This makes it easy to compute the cotangent function.
Implementing Cotangent Function in C++
To implement the cotangent function in C++, we will create a simple program that takes an angle in radians and returns its cotangent. Below is the code for this implementation:
#include <iostream>
#include <cmath>
double cotangent(double angle) {
return 1.0 / tan(angle);
}
int main() {
double angle;
std::cout << "Enter angle in radians: ";
std::cin >> angle;
double result = cotangent(angle);
std::cout << "Cotangent of " << angle << " is: " << result << std::endl;
return 0;
}
Output:
Enter angle in radians: 1
Cotangent of 1 is: 0.642092
In this code, we start by including the necessary libraries. The cotangent
function takes an angle as input and returns its cotangent by calculating the reciprocal of the tangent. In the main
function, we prompt the user to enter an angle in radians, compute its cotangent, and display the result. This approach effectively demonstrates how to implement the cotangent function in C++.
Handling Edge Cases
When working with trigonometric functions, it’s crucial to handle edge cases, particularly when the angle approaches values that make the function undefined. For cotangent, this occurs at angles where sine equals zero (e.g., 0, π, 2π, etc.). Below is an enhanced version of our previous code that checks for these edge cases:
#include <iostream>
#include <cmath>
double cotangent(double angle) {
if (sin(angle) == 0) {
throw std::invalid_argument("Cotangent is undefined for this angle.");
}
return 1.0 / tan(angle);
}
int main() {
double angle;
std::cout << "Enter angle in radians: ";
std::cin >> angle;
try {
double result = cotangent(angle);
std::cout << "Cotangent of " << angle << " is: " << result << std::endl;
} catch (const std::invalid_argument& e) {
std::cout << e.what() << std::endl;
}
return 0;
}
Output:
Enter angle in radians: 0
Cotangent is undefined for this angle.
In this version, we added a check in the cotangent
function to see if the sine of the angle is zero. If it is, we throw an exception indicating that the cotangent is undefined. This makes our program more robust and user-friendly, as it informs users of invalid inputs.
Conclusion
The cotangent function is an essential part of trigonometry, and implementing it in C++ is straightforward with the help of the <cmath>
library. By understanding how to compute cotangent and handle edge cases, you can effectively work with trigonometric functions in your C++ programs. Whether you’re building applications in physics, engineering, or graphics, knowing how to implement these functions will enhance your programming toolkit. Keep practicing, and soon you’ll master not just cotangent, but all trigonometric functions in C++!
FAQ
-
What is the cotangent function?
The cotangent function is the reciprocal of the tangent function, defined as cot(x) = cos(x) / sin(x). -
How do I implement the cotangent function in C++?
You can implement the cotangent function in C++ using thetan
function from the<cmath>
library and return the reciprocal. -
What happens if I input an angle where cotangent is undefined?
The program should handle this by checking if the sine of the angle is zero and informing the user that cotangent is undefined for that input. -
Is the cotangent function periodic?
Yes, the cotangent function is periodic with a period of π, meaning cot(x) = cot(x + π). -
Can I use degrees instead of radians in my implementation?
Yes, but you’ll need to convert degrees to radians before using trigonometric functions in C++.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn