How to Solve Control Reaches End of Non-Void Function Error in C++
-
Use the
return
Statement at the End of the Function Body -
Use the
return
Statements at the End of Each Code Path of the Function Body
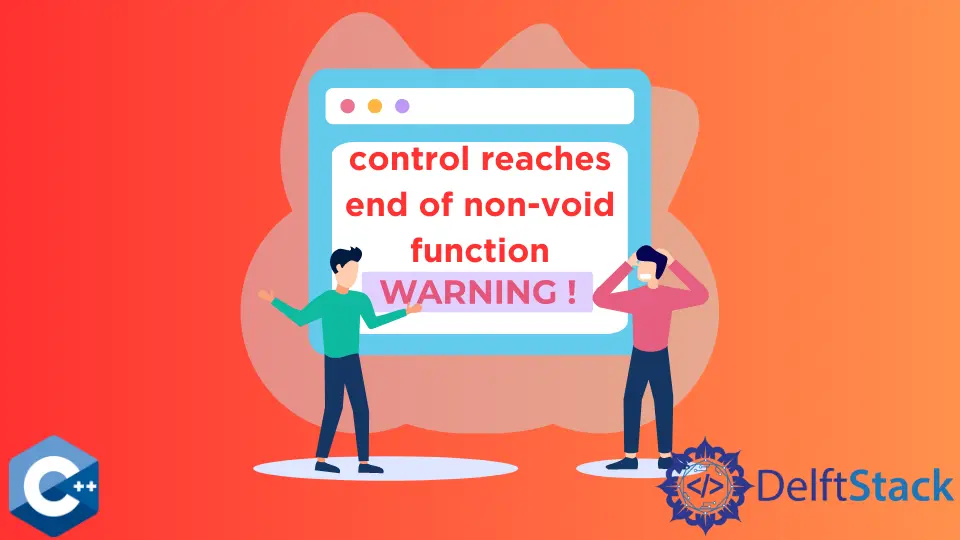
This article will explain several methods of solving control reaches end of non-void function error C++.
Use the return
Statement at the End of the Function Body
Non-void functions are required to have a return type. Consequently, the function needs to have a statement that returns the object of the corresponding type. If certain compiler flags are passed, this type of error or warning might get suppressed entirely, which will lead to run-time faults if the given function gets called in the program.
The following example code has the reverseString
function defined, which takes a reference to a string and returns the string value. If we look into a function body, there is no return
statement. Even though the reverseString
does not pass any arguments to the caller function, the compiler only displays the warning message, and the executable program is built anyway. If the function is called, then the control flow would most likely lead to a segmentation fault.
#include <algorithm>
#include <iostream>
#include <iterator>
using std::cout;
using std::endl;
using std::reverse;
using std::string;
string reverseString(string &s) { string rev(s.rbegin(), s.rend()); }
int main() {
string str = "This string is arbitrary";
int cond = -1;
cout << str << endl;
cout << reverseString(str, cond) << endl;
return EXIT_SUCCESS;
}
Use the return
Statements at the End of Each Code Path of the Function Body
Another scenario where the control reaches the end of a non-void function is when the conditional blocks don’t have the return
statements in every path. Thus, if the execution in the non-void function is branched and if
statements don’t cover every possible path, then there needs to be an explicit return
call at the end of the function body.
The next example demonstrates the string manipulation function with two conditional paths that pass the return value to the caller function. However, some cases are left not evaluated for the given condition, which implies that control flow might reach the end of the function block and cause run-time errors.
#include <algorithm>
#include <iostream>
#include <iterator>
using std::cout;
using std::endl;
using std::reverse;
using std::string;
string reverseString(string &s, int condition) {
if (condition == -1) {
string rev(s.rbegin(), s.rend());
return s;
} else if (condition == 0) {
return s;
}
}
int main() {
string str = "This string is arbitrary";
int cond = -1;
cout << str << endl;
cout << reverseString(str, cond) << endl;
return EXIT_SUCCESS;
}
You will possibly see the warning below.
Main.cpp:15:1: warning: non-void function does not return a value in all control paths [-Wreturn-type]
}
^
1 warning generated.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook