How to Use const Qualifier With Pointers in C++
- Understanding Pointers and const Qualifiers
- Best Practices When Using const with Pointers
- Conclusion
- FAQ
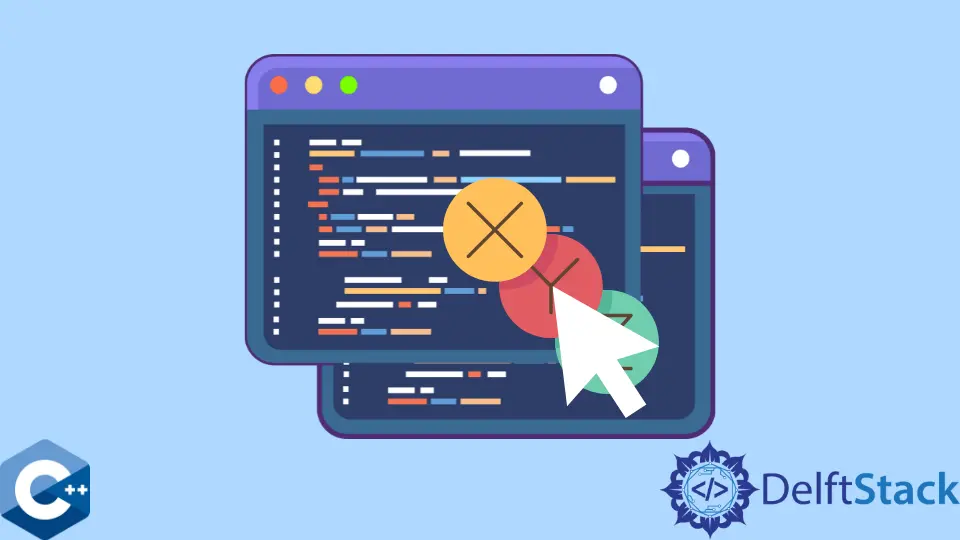
In the world of C++, understanding the const
qualifier and how it interacts with pointers is essential for writing robust and maintainable code. When you declare a pointer as const
, you are essentially telling the compiler and other developers that the data being pointed to should not be modified through that pointer. This can help prevent accidental changes to data and improve code safety.
In this article, we will delve into how to effectively use the const
qualifier with pointers in C++, exploring various scenarios and providing clear examples to illustrate these concepts. Whether you’re a beginner or looking to refine your skills, this guide will provide the insights you need.
Understanding Pointers and const Qualifiers
Before diving into the specifics of using const
with pointers, it’s important to understand what pointers are in C++. A pointer is a variable that stores the memory address of another variable. This capability allows for powerful manipulation of data in memory. The const
qualifier, when applied to pointers, can be used in two main ways: to make the pointer itself constant or to make the data being pointed to constant.
Pointer to Constant Data
When you declare a pointer to constant data, you indicate that the data being pointed to should not be modified through that pointer. This is done using the syntax:
const int* ptr;
Here, ptr
is a pointer to an integer that is constant. This means you cannot change the value of the integer being pointed to via ptr
.
#include <iostream>
using namespace std;
void display(const int* ptr) {
cout << "Value: " << *ptr << endl;
}
int main() {
int num = 10;
const int* ptr = #
display(ptr);
// The following line will cause a compilation error
// *ptr = 20;
return 0;
}
Output:
Value: 10
In this example, we create a constant pointer to an integer and pass it to the display
function. The function can read the value but cannot modify it. Attempting to change the value through ptr
would result in a compilation error, ensuring that the integrity of the data is maintained.
Constant Pointer to Data
In contrast, if you want to ensure that the pointer itself cannot be changed to point to another memory location, you would declare a constant pointer. This is done using the syntax:
int* const ptr;
Here, ptr
is a constant pointer to an integer, meaning you cannot change where ptr
points, but you can modify the integer value at that address.
#include <iostream>
using namespace std;
void modify(int* const ptr) {
*ptr = 30;
}
int main() {
int num = 20;
int* const ptr = #
modify(ptr);
cout << "Modified Value: " << *ptr << endl;
// The following line will cause a compilation error
// ptr = nullptr;
return 0;
}
Output:
Modified Value: 30
In this example, we have a constant pointer that allows modification of the integer value it points to, but we cannot change the pointer itself to point to a different integer. This provides a level of safety in managing memory addresses while still allowing modifications to the data.
Constant Pointer to Constant Data
Combining both concepts, you can create a pointer that is both constant and points to constant data. The syntax for this is:
const int* const ptr;
This means neither the pointer can be changed, nor can the data it points to be modified.
#include <iostream>
using namespace std;
void show(const int* const ptr) {
cout << "Value: " << *ptr << endl;
}
int main() {
int num = 40;
const int* const ptr = #
show(ptr);
// The following lines will cause compilation errors
// *ptr = 50;
// ptr = nullptr;
return 0;
}
Output:
Value: 40
In this case, we have both a constant pointer and constant data. The function can display the value but cannot modify it or change the pointer to point elsewhere. This is useful in scenarios where you want to ensure both the data and the pointer remain unchanged throughout the program.
Best Practices When Using const with Pointers
Using const
with pointers can significantly enhance the safety and clarity of your code. Here are some best practices to consider:
-
Use const for Function Parameters: When passing pointers to functions, use
const
to prevent accidental modifications. This makes your intentions clear and protects the data. -
Prefer const Over Non-const: If you do not need to modify the data, always prefer using
const
pointers. This helps in avoiding unintended side effects. -
Document Your Code: Make sure to document your code effectively. Indicate when a pointer is meant to be constant or when the data it points to should remain unchanged.
-
Combine const Qualifiers: Use combinations of
const
qualifiers to express your intent clearly. This can help in maintaining the integrity of your data structures. -
Be Aware of Pointer Arithmetic: When using pointer arithmetic, be cautious about
const
qualifiers. Ensure that you are not inadvertently modifying data through a pointer that should remain constant.
By following these best practices, you can leverage the power of const
qualifiers in your code, leading to safer and more maintainable C++ programs.
Conclusion
Understanding how to use the const
qualifier with pointers in C++ is crucial for creating safe and efficient code. By distinguishing between constant data and constant pointers, developers can prevent unintended modifications and enhance code clarity. Utilizing const
not only improves safety but also communicates intent clearly to anyone reading your code. Remember to apply these concepts in your programming practices to reap the benefits of robust and maintainable C++ applications.
FAQ
-
What is a pointer in C++?
A pointer is a variable that stores the memory address of another variable, allowing for direct manipulation of memory. -
What does the const qualifier do?
Theconst
qualifier indicates that a variable’s value cannot be changed after initialization, providing a safeguard against unintended modifications. -
Can I modify the value of a constant pointer?
Yes, you can modify the value that a constant pointer points to, but you cannot change the pointer itself to point to another address. -
Why should I use const with pointers?
Usingconst
with pointers enhances code safety, prevents accidental data modification, and clarifies the intent of your code. -
How do I declare a constant pointer to constant data?
You can declare a constant pointer to constant data using the syntax:const int* const ptr;
This prevents both the pointer and the data it points to from being modified.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ Const
- Const at the End of Function in C++
- The const Keyword in C++
- How to Use the const Keyword With Pointers in C++