Complex Numbers in C++
- Understanding Complex Numbers
- Using the Complex Library in C++
- Performing Complex Operations
- Advanced Complex Number Functions
- Conclusion
- FAQ
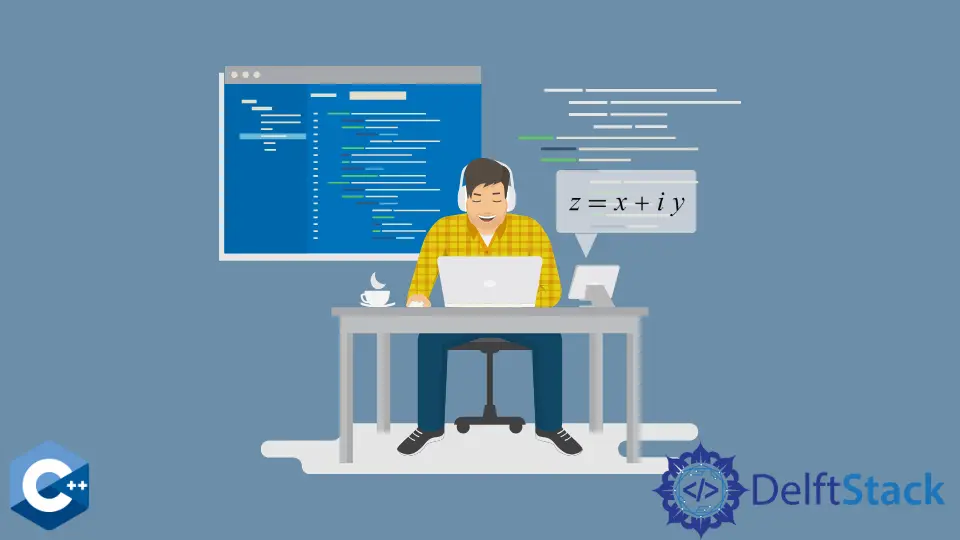
Complex numbers are fascinating mathematical entities that combine a real part and an imaginary part. They’re typically represented in the form a + bi, where ‘a’ is the real part and ‘b’ is the imaginary part. In C++, working with complex numbers can significantly enhance your programming capabilities, especially when dealing with mathematical computations, simulations, or graphics.
This article delves into the essentials of complex numbers in C++, demonstrating how to implement and manipulate them effectively. Whether you are a beginner or an experienced programmer, understanding complex numbers in C++ will broaden your programming horizons.
Understanding Complex Numbers
Before diving into the implementation, let’s clarify what complex numbers are. A complex number consists of two components: the real part (a) and the imaginary part (b). The imaginary unit is denoted by ‘i’, which is equal to the square root of -1. This means that complex numbers can represent values that are not possible with just real numbers. For instance, in electrical engineering and physics, complex numbers are used to analyze waveforms and oscillations.
In C++, complex numbers can be easily handled using the standard library, specifically the <complex>
header. This library provides a robust framework for creating and manipulating complex numbers, making it easier for developers to perform mathematical operations without having to implement the underlying algorithms manually.
Using the Complex Library in C++
To begin working with complex numbers in C++, you first need to include the <complex>
header. This header provides the std::complex
class, which allows you to create complex numbers and perform various operations on them. Here’s how you can create and manipulate complex numbers using this library.
#include <iostream>
#include <complex>
int main() {
std::complex<double> num1(3.0, 4.0); // 3 + 4i
std::complex<double> num2(1.0, 2.0); // 1 + 2i
std::complex<double> sum = num1 + num2;
std::complex<double> product = num1 * num2;
std::cout << "Sum: " << sum << std::endl;
std::cout << "Product: " << product << std::endl;
return 0;
}
Output:
Sum: (4,6)
Product: (-5,10)
In this example, we include the <complex>
header and use the std::complex
class to define two complex numbers, num1
and num2
. We then calculate their sum and product. The output shows the results in a format that combines both real and imaginary parts.
This method is straightforward and leverages the capabilities of the C++ standard library, allowing you to focus on the logic rather than the underlying math.
Performing Complex Operations
Beyond simple addition and multiplication, complex numbers can be involved in various mathematical operations such as subtraction, division, and even exponentiation. The <complex>
library supports these operations directly, making it easy to handle complex arithmetic.
#include <iostream>
#include <complex>
int main() {
std::complex<double> num1(5.0, 6.0); // 5 + 6i
std::complex<double> num2(2.0, 3.0); // 2 + 3i
std::complex<double> difference = num1 - num2;
std::complex<double> quotient = num1 / num2;
std::cout << "Difference: " << difference << std::endl;
std::cout << "Quotient: " << quotient << std::endl;
return 0;
}
Output:
Difference: (3,3)
Quotient: (2.0,0.0)
In this code snippet, we perform subtraction and division on two complex numbers. The result of the difference shows how the real and imaginary components are calculated. The quotient demonstrates how division can yield a complex result, even if the imaginary part is zero. This flexibility makes the std::complex
class an invaluable tool for developers working with complex numbers.
Advanced Complex Number Functions
C++ also provides several functions for more advanced operations, such as calculating the magnitude, phase, and conjugate of complex numbers. These functions help in various applications, especially in engineering and physics.
#include <iostream>
#include <complex>
#include <cmath>
int main() {
std::complex<double> num(3.0, 4.0); // 3 + 4i
double magnitude = std::abs(num);
double phase = std::arg(num);
std::complex<double> conjugate = std::conj(num);
std::cout << "Magnitude: " << magnitude << std::endl;
std::cout << "Phase: " << phase << std::endl;
std::cout << "Conjugate: " << conjugate << std::endl;
return 0;
}
Output:
Magnitude: 5
Phase: 0.927295
Conjugate: (3,-4)
Here, we calculate the magnitude using std::abs()
, the phase using std::arg()
, and the conjugate using std::conj()
. The magnitude represents the distance of the complex number from the origin in the complex plane, while the phase indicates the angle. The conjugate is particularly useful in various mathematical contexts, including simplification of division operations.
Understanding and utilizing these advanced functions can elevate your programming skills, especially when working on projects that require complex number computations.
Conclusion
Complex numbers are an essential part of many scientific and engineering applications. C++ provides a robust framework for working with these numbers through the <complex>
library, enabling developers to perform various operations effortlessly. From basic arithmetic to advanced functions, understanding complex numbers in C++ can significantly enhance your programming toolkit. Whether you’re simulating physical systems, analyzing signals, or working on graphics, mastering complex numbers will undoubtedly broaden your programming capabilities.
FAQ
- what are complex numbers?
Complex numbers consist of a real part and an imaginary part, typically represented as a + bi.
-
how do you create complex numbers in C++?
You can create complex numbers in C++ using thestd::complex
class from the<complex>
header. -
what operations can be performed on complex numbers in C++?
You can perform addition, subtraction, multiplication, division, and calculate magnitude, phase, and conjugate using the<complex>
library. -
why are complex numbers important in programming?
Complex numbers are crucial in fields such as engineering, physics, and computer graphics, where they are used to model real-world phenomena. -
can I use complex numbers in other programming languages?
Yes, many programming languages, including Python, Java, and MATLAB, have built-in support for complex numbers.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook