How to Clear Stringstream in C++
-
Use the
str("")
and theclear()
Method to Clearstringstream
in C++ -
Use Scoping to Clear
stringstream
in C++
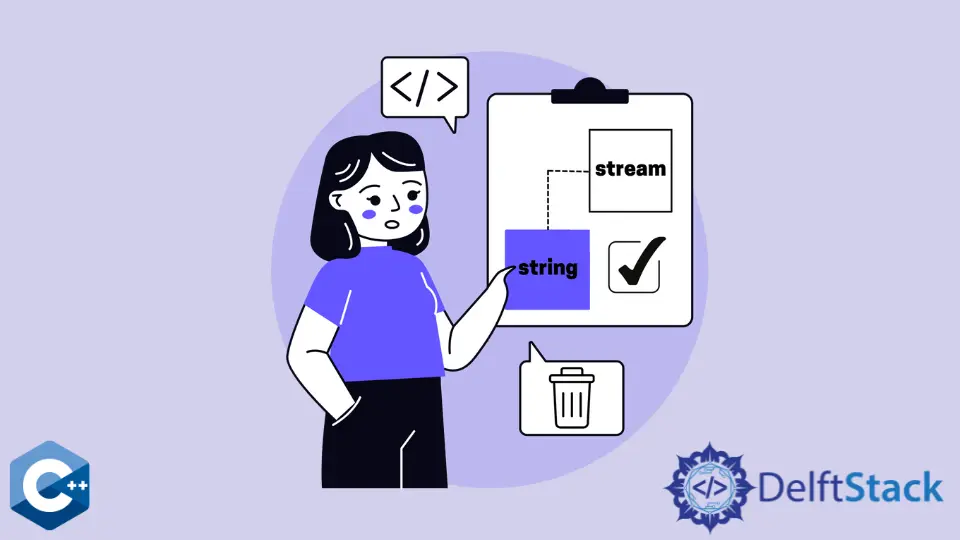
This article will teach different ways to clear or empty stringstreams
in C++.
Use the str("")
and the clear()
Method to Clear stringstream
in C++
To clear out or empty stringstream
, we can use the str("")
and clear()
method, but we have to use both the methods; else the program might not work properly, i.e., it won’t give the correct output.
Example code (Using str("")
only):
#include <bits/stdc++.h>
using namespace std;
int main() {
string temp;
string temp2;
stringstream ss;
for (int i = 0; i < 5; i++)
{
ss.str("");
ss << i;
temp = ss.str();
ss >> temp2;
cout << temp << " " << ss.str() << " " << temp2 << endl;
}
}
Output:
0 0 0
0
0
0
0
The above output is not correct because using ss.str("")
does clear the string, but the issue is that we can’t add new values to the stream.
That’s why in the first line, we get the correct output 0 0 0
, but in the next iteration, both the strings, temp
and temp2
, become empty as new values don’t get added to the stream. So it’s like assigning an empty string to both of them.
Example code (Using str("")
and clear()
together):
clear()
function resets the error state of the stream. Whenever there is an error in the stream, the error state is set to eofbit
(end of file), and by using clear()
, we can reset it back to goodbit
, saying there is no error.
#include <bits/stdc++.h>
using namespace std;
int main() {
string temp;
string temp2;
stringstream ss;
for (int i = 0; i < 5; i++)
{
ss.clear();
ss.str("");
ss << i;
temp = ss.str();
ss >> temp2;
cout << temp << " " << ss.str() << " " << temp2 << endl;
}
}
Output:
0 0 0
1 1 1
2 2 2
3 3 3
4 4 4
Explanation: Here, we get the correct output as using clear()
along with str("")
allows us to add new values to the stream after clearing it.
Use Scoping to Clear stringstream
in C++
Though ineffective as the first method, this is a workaround to clear the stringstream
. We can use the concept of scoping.
Example code:
#include <bits/stdc++.h>
using namespace std;
int main() {
{
stringstream ss;
ss << "our code part ";
cout << ss.str() << endl;
}
{
stringstream ss;
ss << "Tony ";
cout << ss.str() << endl;
}
{
stringstream ss;
ss << "stark ";
cout << ss.str() << endl;
}
}
Output:
our code part
Tony
stark
Explanation: Here, we have to use curly brackets { }
to define the scope of that stringstream
variable. Once out of the scope, it gets cleared.