How to Use cin.fail Method in C++
-
Understanding the
cin.fail
Method - Handling Input Validation with cin.fail
- Looping Until Valid Input is Received
- Conclusion
- FAQ
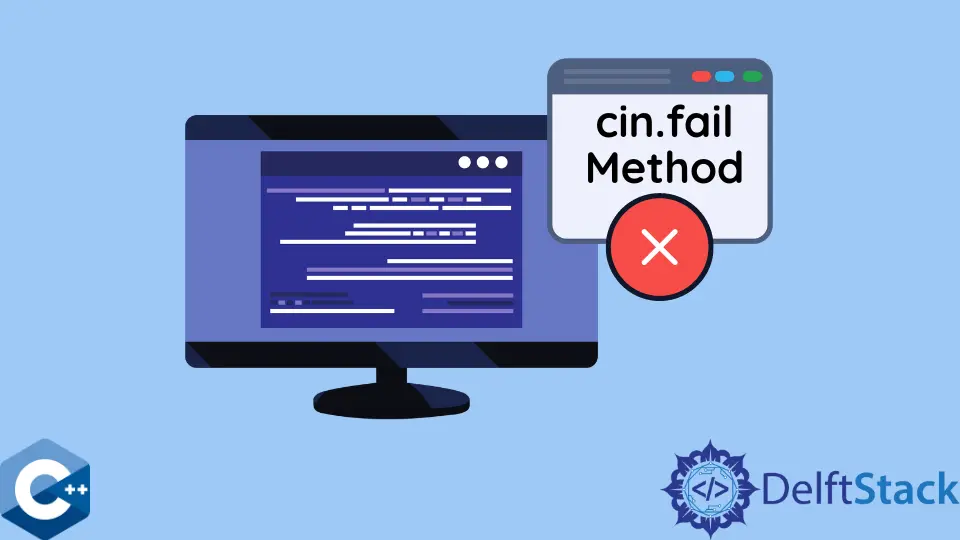
The cin.fail
method is an essential aspect of input handling in C++. It plays a crucial role in determining whether the last input operation failed due to an invalid type or format. Understanding how to use this method correctly can significantly enhance your program’s robustness and user experience.
In this article, we will delve into the intricacies of the cin.fail
method, exploring its functionality and providing practical examples to illustrate its use. Whether you’re a beginner looking to grasp the basics or an experienced programmer aiming to refine your skills, this guide will equip you with the knowledge to effectively utilize cin.fail
in your C++ applications.
Understanding the cin.fail
Method
The cin.fail
method is a member function of the istream
class in C++, which represents input streams. When you attempt to read data using cin
, several things can go wrong, such as entering a character when a number is expected. In such cases, the input stream enters a fail state, which is where cin.fail
becomes useful.
When you call cin.fail()
, it returns a boolean value: true
if the last input operation failed, and false
otherwise. This allows you to check the state of the input stream and handle errors gracefully, ensuring that your program can respond to user mistakes effectively.
Here’s a simple example of using cin.fail
to validate user input:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Please enter a number: ";
cin >> number;
if (cin.fail()) {
cout << "Invalid input! Please enter a valid number." << endl;
cin.clear(); // Clear the fail state
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Ignore the invalid input
} else {
cout << "You entered: " << number << endl;
}
return 0;
}
Output:
Please enter a number: abc
Invalid input! Please enter a valid number.
In this example, if the user inputs a non-numeric value like “abc”, the cin.fail()
method detects the failure. The program then clears the error state using cin.clear()
and ignores the invalid input to allow for a fresh attempt. This way, the user is prompted to enter a valid number again, enhancing the user experience.
Handling Input Validation with cin.fail
Input validation is critical in any application that requires user input. By leveraging the cin.fail
method, you can ensure that your program behaves predictably even when faced with unexpected input.
Imagine you are developing a simple calculator that takes two numbers from the user. You can use cin.fail
to validate each input and ensure that only valid numbers are processed. Here’s how you can implement this:
#include <iostream>
using namespace std;
int main() {
double num1, num2;
cout << "Enter first number: ";
cin >> num1;
if (cin.fail()) {
cout << "Invalid input for first number." << endl;
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
return 1; // Exit the program
}
cout << "Enter second number: ";
cin >> num2;
if (cin.fail()) {
cout << "Invalid input for second number." << endl;
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
return 1; // Exit the program
}
cout << "The sum is: " << num1 + num2 << endl;
return 0;
}
Output:
Enter first number: 5.5
Enter second number: xyz
Invalid input for second number.
In this code, we first prompt the user to enter two numbers. If the user inputs an invalid value for either number, the program immediately informs them of the invalid input, clears the error state, and exits gracefully. This approach prevents further calculations with invalid data, ensuring that your application remains robust and user-friendly.
Looping Until Valid Input is Received
Sometimes, you may want your program to keep prompting the user until valid input is received. This can be easily achieved using a loop in conjunction with cin.fail
. Let’s modify our previous example to implement this functionality:
#include <iostream>
using namespace std;
int main() {
double number;
while (true) {
cout << "Please enter a number: ";
cin >> number;
if (cin.fail()) {
cout << "Invalid input! Please enter a valid number." << endl;
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
} else {
cout << "You entered: " << number << endl;
break; // Exit the loop
}
}
return 0;
}
Output:
Please enter a number: abc
Invalid input! Please enter a valid number.
Please enter a number: 10
You entered: 10
In this example, we use a while
loop to continuously prompt the user for input until they provide a valid number. If the input is invalid, the error state is cleared, and the program prompts the user again. This approach enhances user experience by allowing multiple attempts without terminating the program.
Conclusion
The cin.fail
method is a powerful tool for input validation in C++. By understanding how to use it effectively, you can create robust applications that handle user input gracefully. Whether you’re checking for invalid types, ensuring proper data formats, or looping until valid input is received, mastering cin.fail
is essential for any C++ programmer. With the examples provided, you should now have a solid foundation to implement input validation in your own projects.
FAQ
-
What does cin.fail() do?
cin.fail() checks if the last input operation on the input stream failed, returning true if it did. -
How can I clear the fail state of cin?
You can clear the fail state by using cin.clear(). -
Can I use cin.fail with other data types?
Yes, cin.fail works with any data type that can be read from the input stream, including integers, doubles, and strings. -
How do I ignore invalid input after cin.fail()?
You can ignore invalid input by using cin.ignore(numeric_limits::max(), ‘\n’). -
Is it necessary to check for cin.fail every time I read input?
While it’s not strictly necessary, checking for cin.fail helps ensure that your program handles user input errors gracefully.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook