How to Check if Pointer Is NULL in C++
-
Compare With
nullptr
to Check if Pointer IsNULL
in C++ -
Use Comparison With
0
to Check if Pointer IsNULL
in C++ -
Use Pointer Value as Condition to Check if Pointer Is
NULL
in C++
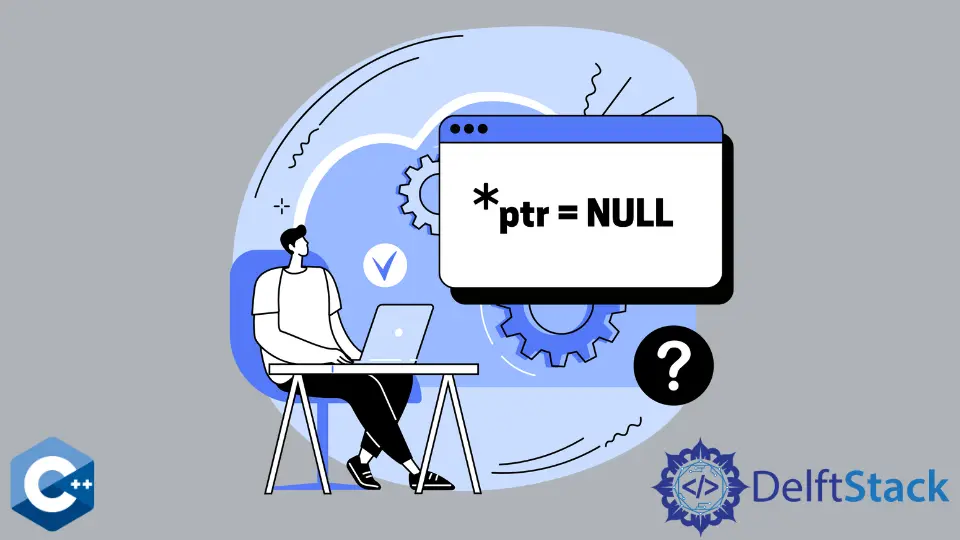
This article will demonstrate multiple methods of how to check if a pointer is null in C++.
Compare With nullptr
to Check if Pointer Is NULL
in C++
The C++ language provides multiple low-level features to manipulate memory directly and specifies the concept of pointer
, which is the object pointing to a memory address. Usually, a pointer should point to some object which is utilized by the executing program. Although, we can also declare a pointer as a null pointer that does not point to any object.
A null pointer is initialized by assigning the literal nullptr
value or with the integer 0
. Note, though, that modern C++ suggests avoiding 0
initialization of pointers because it can lead to undesired results when using overloaded functions. In the following example, we check if the pointer is not equal to a nullptr
, and if the condition is satisfied, we can operate on it.
#include <iostream>
using std::cout;
using std::endl;
#define SIZE 123
int main() {
char *arr = (char *)malloc(SIZE);
if (arr != nullptr) {
cout << "Valid pointer!" << endl;
} else {
cout << "NULL pointer!" << endl;
}
free(arr);
return EXIT_SUCCESS;
}
Output:
Valid pointer!
Use Comparison With 0
to Check if Pointer Is NULL
in C++
There is also a preprocessor variable named NULL
, which has roots in the C standard library and is often used in legacy code. Mind that it is not recommended to use NULL
in contemporary C++ programming because it’s equivalent to initialization by the integer 0
, and the problems may arise as stated in the previous section. Still, we can check if a pointer is null by comparing it to 0
as demonstrated in the next code sample:
#include <iostream>
using std::cout;
using std::endl;
#define SIZE 123
int main() {
char *arr = (char *)malloc(SIZE);
if (arr != 0) {
cout << "Valid pointer!" << endl;
} else {
cout << "NULL pointer!" << endl;
}
free(arr);
return EXIT_SUCCESS;
}
Output:
Valid pointer!
Use Pointer Value as Condition to Check if Pointer Is NULL
in C++
Null pointers are evaluated as false
when they are used in logical expressions. Thus, we can put a given pointer in the if
statement condition to check if it’s null. Note that dereferencing null pointers leads to undefined behavior, which causes a program to terminate abnormally in most cases.
#include <iostream>
using std::cout;
using std::endl;
#define SIZE 123
int main() {
char *arr = (char *)malloc(SIZE);
if (arr) {
cout << "Valid pointer!" << endl;
} else {
cout << "NULL pointer!" << endl;
}
free(arr);
return EXIT_SUCCESS;
}
Output:
Valid pointer!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook