Error: Cannot Call Member Function Without Object in C++
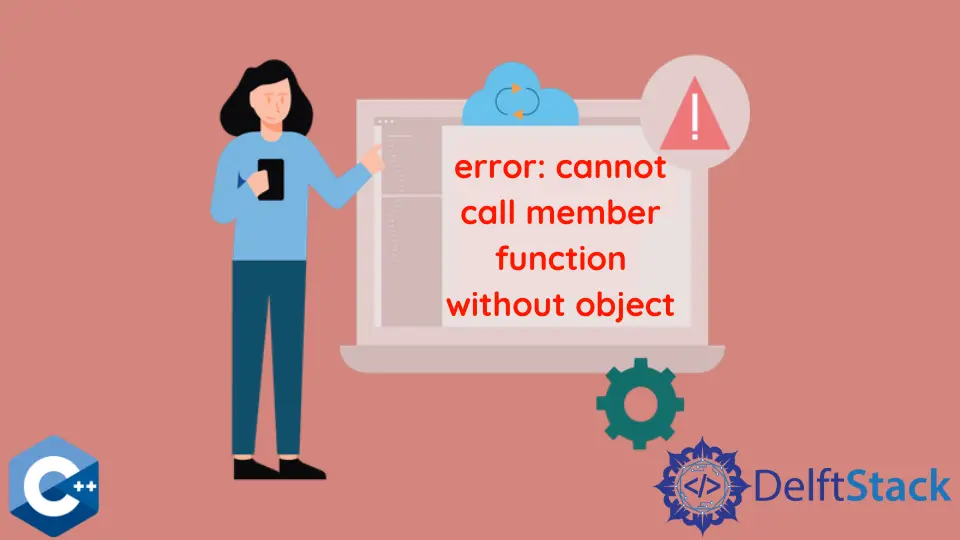
In C++, the error message “Cannot call member function without object” can be quite perplexing for both novice and experienced programmers. This error typically arises when you attempt to invoke a member function of a class without a valid instance of that class. This can lead to confusion, especially when you’re deep into coding and expect everything to run smoothly.
In this article, we will explore the causes of this error and provide effective solutions to resolve it. We’ll break down the error, discuss its implications, and offer practical examples to enhance your understanding. So, let’s dive into the world of C++ and unravel this common coding conundrum.
Understanding the Error
The Cannot call member function without object
error occurs when you try to call a member function on a class without creating an instance of that class. In C++, member functions are designed to operate on specific objects, and attempting to call them without an object leads to this error. This situation often arises when you mistakenly forget to create an object or when you try to call a non-static member function from a static context.
For example, if you have a class named Car
with a member function drive()
, you cannot just call Car::drive()
without creating an instance of Car
. Instead, you need to create an object of Car
first and then call the drive()
function on that object. Understanding how object-oriented programming works in C++ is crucial to avoid this error.
Solution Methods
Method 1: Create an Object
The most straightforward solution to this error is to ensure that you create an object of the class before calling its member function. This approach is essential because member functions are tied to specific instances of a class. Here’s how you can do it:
cppCopy#include <iostream>
using namespace std;
class Car {
public:
void drive() {
cout << "Driving the car!" << endl;
}
};
int main() {
Car myCar; // Creating an object of Car
myCar.drive(); // Calling the member function on the object
return 0;
}
Output:
textCopyDriving the car!
In this example, we define a class Car
with a member function drive()
. In the main()
function, we create an object of Car
named myCar
. Then, we call the drive()
function on the myCar
object. This is the correct way to call a member function, and it resolves the error. Always remember that member functions require an object to operate on, so creating an instance is a critical step.
Method 2: Use Static Member Functions
If you find yourself needing to call a member function without an object, consider defining the function as a static member function. Static member functions belong to the class itself rather than any particular object. This way, you can call them without creating an instance. Here’s an example:
cppCopy#include <iostream>
using namespace std;
class Car {
public:
static void drive() {
cout << "Driving the car!" << endl;
}
};
int main() {
Car::drive(); // Calling the static member function directly
return 0;
}
Output:
textCopyDriving the car!
In this code snippet, we declare the drive()
function as a static member of the Car
class. This allows us to call Car::drive()
directly without needing to create an instance of Car
. Static member functions are particularly useful for utility functions or when you don’t need to maintain any state related to a specific object. However, remember that static functions cannot access non-static member variables or functions.
Method 3: Check for Null Pointers
Another common scenario that can lead to the “Cannot call member function without object” error is when you attempt to call a member function on a null pointer. Always ensure that your object is properly initialized before calling its member functions. Here’s how you can implement this check:
cppCopy#include <iostream>
using namespace std;
class Car {
public:
void drive() {
cout << "Driving the car!" << endl;
}
};
int main() {
Car* myCar = nullptr; // Pointer initialized to null
if (myCar) {
myCar->drive(); // Call only if myCar is not null
} else {
cout << "myCar is null. Cannot call member function." << endl;
}
return 0;
}
Output:
textCopymyCar is null. Cannot call member function.
In this example, we declare a pointer to Car
named myCar
and initialize it to nullptr
. Before calling drive()
, we check if myCar
is not null. If it is null, we print a message indicating that we cannot call the member function. This approach helps prevent runtime errors and ensures that your code runs smoothly.
Conclusion
The “Cannot call member function without object” error in C++ is a common pitfall that can be easily avoided with a solid understanding of object-oriented programming principles. By creating an object of the class, using static member functions, or checking for null pointers, you can effectively resolve this error. Remember, member functions need an instance to operate on, so always ensure you have a valid object before making a call. With these strategies in your toolkit, you’ll be better equipped to tackle similar issues in your coding journey.
FAQ Section
- what causes the cannot call member function without object error?
This error occurs when you attempt to call a non-static member function without an instance of the class.
-
how can I resolve this error in C++?
You can resolve this error by creating an object of the class before calling the member function or by defining the function as static. -
can I call a member function from a static context?
No, you cannot call a non-static member function from a static context without an object. -
what should I do if I have a null pointer?
Always check if the pointer is not null before calling a member function to avoid runtime errors. -
are there any best practices to avoid this error?
Yes, always ensure that you properly instantiate your objects and utilize static functions when appropriate.