How to Calculate the Average in C++
- Calculate the Average of Numbers
- Calculate the Average in C++
- Calculate the Average of Arrays in C++
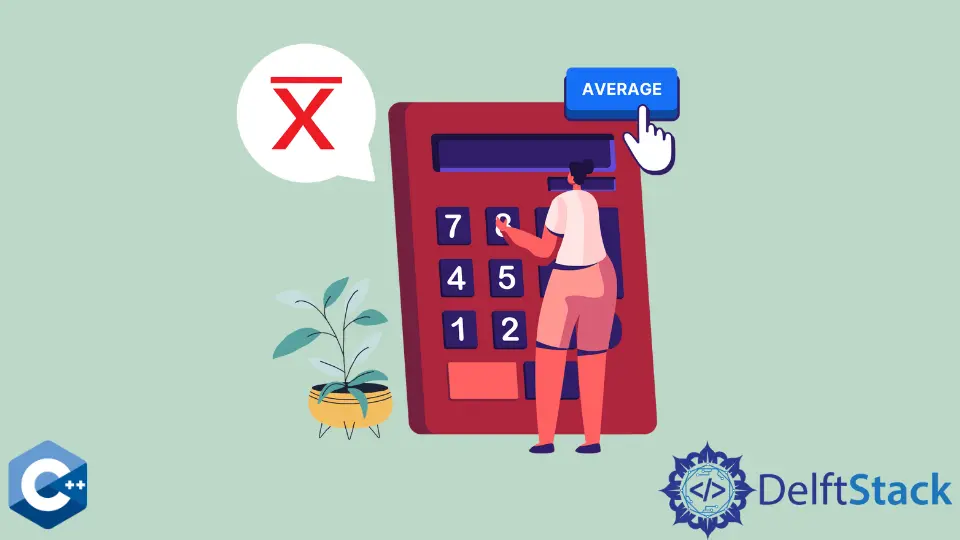
In this tutorial, we will discuss different methods to calculate the average of a series of numbers using C++.
Calculate the Average of Numbers
The average of numbers is calculated by adding all numbers and then dividing the sum by the count of the given numbers.
Assume we have the following series of numbers and want to calculate the average for this series.
10,30,40,50,60
The sum of the series is:
190
To find the average, we divide the sum by the count of numbers in the series, i.e., 5.
Formula:
Average = Sum / Count of numbers
Therefore, the average is:
$190/5 = 38$
Calculate the Average in C++
Assume that we have three numbers, 10, 20, and 30, stored in variables a
, b
, and c
, respectively. The average can be calculated as:
#include <iostream>
using namespace std;
int main() {
int a, b, c, sum;
float avg;
a = 10;
b = 20;
c = 30;
sum = a + b + c;
avg = sum / 3;
cout << "Average of the numbers is: " << avg;
return 0;
}
Output:
Average of the numbers is: 20
In the above code, four variables of integer type a
, b
, c
, sum
, and one float variable avg
(because an average can be in decimal point) are initialized. The sum and average are calculated on lines 11 and 12, respectively.
Calculate the Average of Arrays in C++
In the following example, we will look into calculating the average of elements of an array.
#include <iostream>
using namespace std;
int main() {
float arr[] = {91, 87, 36, 17, 10};
int size, i;
float average, sum = 0.0;
size = sizeof(arr) / sizeof(arr[0]);
for (i = 0; i < size - 1; i++) sum = sum + arr[i];
average = sum / size;
cout << "Average of the elements of the array is: " << average << endl;
return 0;
}
Output:
Average of the elements of the array is: 46.2
The array of type float is initialized in the following line:
float arr[] = {91, 87, 36, 17, 10};
And the size of the array is calculated by the line:
size = sizeof(arr) / sizeof(arr[0]);
In the above line of code, size
is calculated by the sizeof(arr)
function and divided by sizeof(arr[0])
. This is because sizeof(arr)
returns the size of the complete array in Bytes (which is 5*4=20
Bytes in our case), so we divided it by the size of one index (i.e., 4 bytes) to get the total counts of indexes.
The for
loop starts from 0 to size-1
to sum all the array elements and is done in lines 8-9. We divide the sum by total indices in the array to calculate the average, as demonstrated in line 10.