Array of Arrays in C++
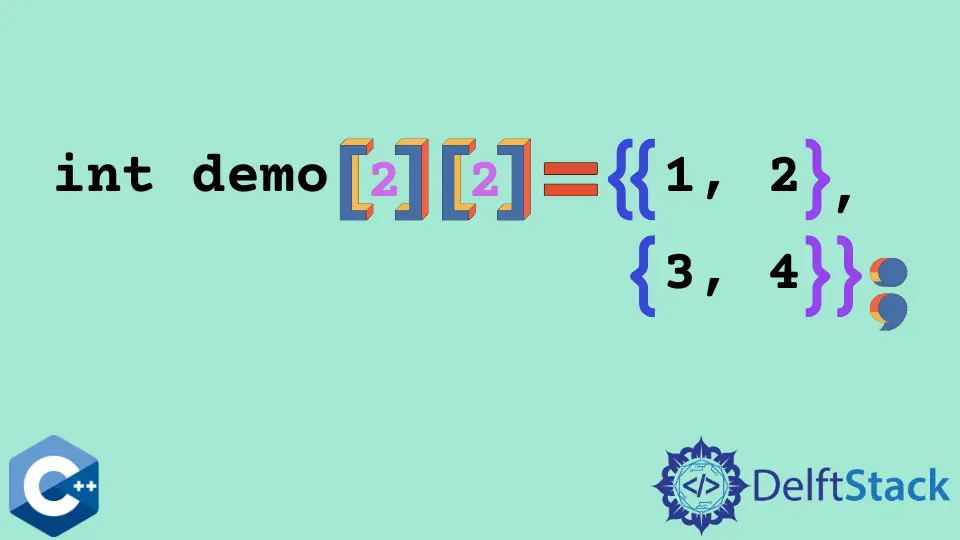
The multi-dimensional array is a data structure that stores arrays of multiple dimensions. It is an extension of the single-dimensional array, which can store only one dimension.
In C++, a multi-dimensional array is, by definition, an array of arrays that stores homogeneous data in a single block of contiguous memory.
A multi-dimensional array has the same number of rows and columns, but it can have different numbers of columns for each row. The dimensionality refers to the number of axes required to specify the position of any given element within the matrix.
The benefits of using a multi-dimensional array in C++ are that it is easier and more efficient. With this data structure, you will not have to worry about the size of your memory or the number of indices you need for each dimension.
C++ Two-Dimensional Arrays
The two-dimensional array is the most basic type of multi-dimensional array. A list of one-dimensional arrays makes up a two-dimensional array in its basic form.
You would type something like the following to declare a two-dimensional integer array with dimensions x
and y
:
type arrayName [x] [y];
Where type
can be any legal data type recognized by C++, and arrayName
is a legal C++ identifier.
Steps to Declare an N-dimensional Array
Declaring an n-dimensional array in C++ is a process of specifying the size of each dimension and the number of elements in it. It is done by following these steps:
-
Define the data type for each dimension.
-
Specify the size of each dimension using a comma-separated list of integers after the data type.
-
Specify the number of elements in that dimension using a comma-separated list of integers after the data type and before the attribute name, e.g.,
int[3][4]={3,5,7}
.
Example code:
#include <iostream>
using namespace std;
int main() {
int demo[3][3] = {{1, 3, 8}, {9, 3, 6}, {2, 4, 7}};
for (int x = 0; x < 3; ++x) {
for (int y = 0; y < 3; ++y) {
cout << "demo[" << x << "][" << y << "] = " << demo[x][y] << endl;
}
}
return 0;
}
Output:
demo[1][2] = 6
demo[2][0] = 2
demo[2][1] = 4
demo[2][2] = 7
Click here to check the working of the code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook