The Difference Between Function Arguments and Parameters in C++
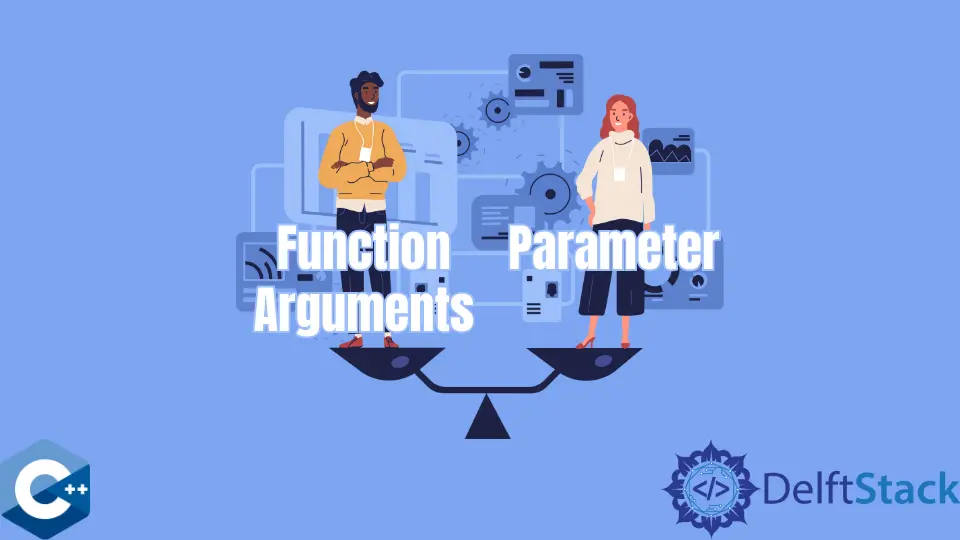
This article will explain the differences between function arguments and parameters in C++.
The Anatomy of Functions in C++
Functions are the common building block of any relatively sophisticated program. They make it possible to define the recurrent routines in the program as separate blocks and call them when the corresponding code needs to be executed. Splitting up a program into multiple functions makes the program more modular and easy to maintain and understand. Consequently, these programs are generally easier to test and debug.
The functions usually have names that uniquely identify them; however, some concepts like function overloading allow the programmer to define multiple functions with the same name; we will explore this feature in the later paragraphs. Lambda expressions are also included in the mix, which provides a way to define unnamed function objects. The sum of different statements in the function block is called a function body. Functions also have a return type that represents the type of the value passed back to the caller.
Another critical part of the function is a list of parameters that denote the variables declared as a part of the function’s signature. The caller usually passes some concrete values to initialize these variables in the function scope. The latter values are called arguments, and they are said to be initializers for the function’s parameters. Still, the terms - parameters and arguments may be used interchangeably in some contexts.
In the example program below, we show you a function named - printVector
, which has only one parameter (named vec
) defined in its signature. When the process is invoked in the main
function, the vec1
argument is passed to it. Once the execution moves to the printVector
function body, the vec
parameter is initialized with the corresponding value.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(const vector<T> &vec) {
for (auto &i : vec) {
cout << i << "; ";
}
cout << endl;
}
int main() {
vector<int> vec1{11, 82, 39, 72, 51, 32, 91};
printVector(vec1);
return EXIT_SUCCESS;
}
Output:
11; 82; 39; 72; 51; 32; 91;
As mentioned in the previous chapter, C++ supports the concept of function overloading, which allows the programmer to define multiple functions with the same name. However, these functions must differ by the number of arguments they accept or the types of the arguments.
The following program below demonstrates the basic usage for overloaded functions that multiplies two numbers of the type int
or double
, respectively.
#include <iostream>
using std::cout;
using std::endl;
int multiply(int x, int y) { return x * y; }
double multiply(double x, double y) { return x * y; }
int main() {
int i1 = 10;
double d1 = 0.1;
cout << multiply(i1, i1 + 1) << endl;
cout << multiply(d1, d1 + 1) << endl;
return EXIT_SUCCESS;
}
Output:
110
0.11
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook