C++ Anonymous Struct
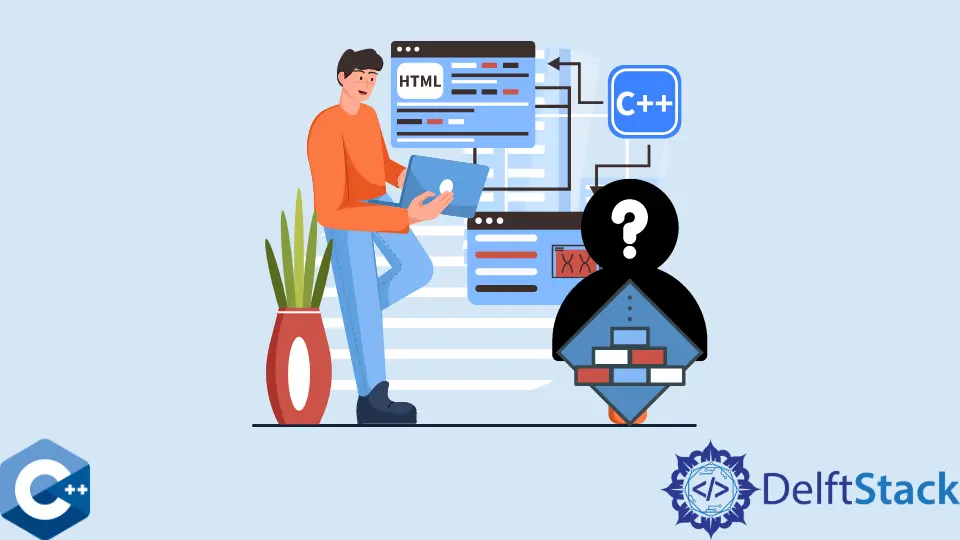
This tutorial demonstrates the use of anonymous structs in C++.
Anonymous Struct in C++
Anonymous structs are not supported in C++ as it was also not supported in C language, but C++ does support anonymous unions, unlike C. According to ANSI C and C++ standards on structs and unions:
- ANSI C++ anonymous unions: Supported
- ANSI C++ anonymous structs: Not Supported
- ANSI C anonymous unions: Supported
- ANSI C anonymous structs: Not Supported
C++ doesn’t support anonymous structs because C doesn’t support it, which is necessary for compatibility. There is no use of anonymous structs in C++, but it provides some alternatives.
For example, see the code below.
struct DemoVector {
float v[3];
float &operator[](int x) { return v[x]; }
float &a() { return v[0]; }
float &b() { return v[1]; }
float &c() { return v[2]; }
};
The code above provides facilities for user-defined types similar to anonymous structs. A similar thing can be done using the unions in C++.
See the code below.
union DemoVector {
struct {
float a, b, c;
};
float v[3];
};
The anonymous structs are now permitted in ISO C11
, gcc
, and Apple’s llvm
but not supported with ISO C++11
.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook