How to Convert String Into Binary Sequence in C++
-
Use the
bitset<N>
Class to Convert String Into Binary Sequence in C++ - Use Custom Function to Convert String Into Binary Sequence in C++
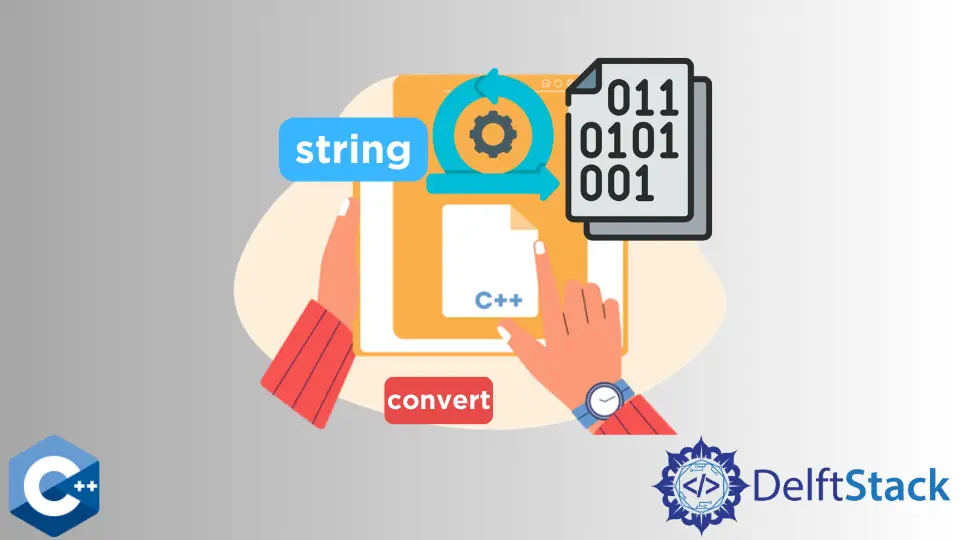
This article will demonstrate multiple methods of how to convert a string into a binary sequence in C++.
Use the bitset<N>
Class to Convert String Into Binary Sequence in C++
Given the arbitrary string sequence, we will convert each character in it to the corresponding binary representation. Since the ASCII characters are associated with the integral numbers and the char
value can be treated as the int
, we will utilize the bitset<N>
class to initialize a fixed binary sequence each character.
Note that one of the constructors of the bitset<N>
provides a way to construct the binary sequence from the character values, but regardless the char
would have been cast to the integral value even if the int
is expected. The above solution requires the traversal of the whole string. Also, notice that, if
statement is only placed inside the loop to control the format of printed output.
#include <bitset>
#include <iostream>
#include <vector>
using std::bitset;
using std::cout;
using std::endl;
using std::string;
int main() {
string str = "Arbitrary string to be converted to binary sequence.";
for (int i = 0; i < str.length(); ++i) {
bitset<8> bs4(str[i]);
cout << bs4 << " ";
if (i % 6 == 0 && i != 0) cout << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
01000001 01110010 01100010 01101001 01110100 01110010 01100001
01110010 01111001 00100000 01110011 01110100 01110010
01101001 01101110 01100111 00100000 01110100 01101111
00100000 01100010 01100101 00100000 01100011 01101111
01101110 01110110 01100101 01110010 01110100 01100101
01100100 00100000 01110100 01101111 00100000 01100010
01101001 01101110 01100001 01110010 01111001 00100000
01110011 01100101 01110001 01110101 01100101 01101110
01100011 01100101 00101110
Use Custom Function to Convert String Into Binary Sequence in C++
Alternatively, we can define a function that will take an int
value and return the binary representation as std::string
object. This version also requires iteration until the given character value is reduced to 0 by dividing it by two. Mind though, the previous solution outputs the big-endian representation as we generally use in written numbers, and the following example outputs the little-endian as the underlying machine stores them.
#include <bitset>
#include <iostream>
#include <vector>
using std::bitset;
using std::cout;
using std::endl;
using std::string;
string toBinary(int n) {
string r;
while (n != 0) {
r += (n % 2 == 0 ? "0" : "1");
n /= 2;
}
return r;
}
int main() {
string str = "Arbitrary string to be converted to binary sequence.";
for (int i = 0; i < str.length(); ++i) {
cout << toBinary(str[i]) << " ";
if (i % 6 == 0 && i != 0) cout << endl;
}
return EXIT_SUCCESS;
}
Output:
1000001 0100111 0100011 1001011 0010111 0100111 1000011
0100111 1001111 000001 1100111 0010111 0100111
1001011 0111011 1110011 000001 0010111 1111011
000001 0100011 1010011 000001 1100011 1111011
0111011 0110111 1010011 0100111 0010111 1010011
0010011 000001 0010111 1111011 000001 0100011
1001011 0111011 1000011 0100111 1001111 000001
1100111 1010011 1000111 1010111 1010011 0111011
1100011 1010011 011101
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++