How to Convert C# Codes to C++
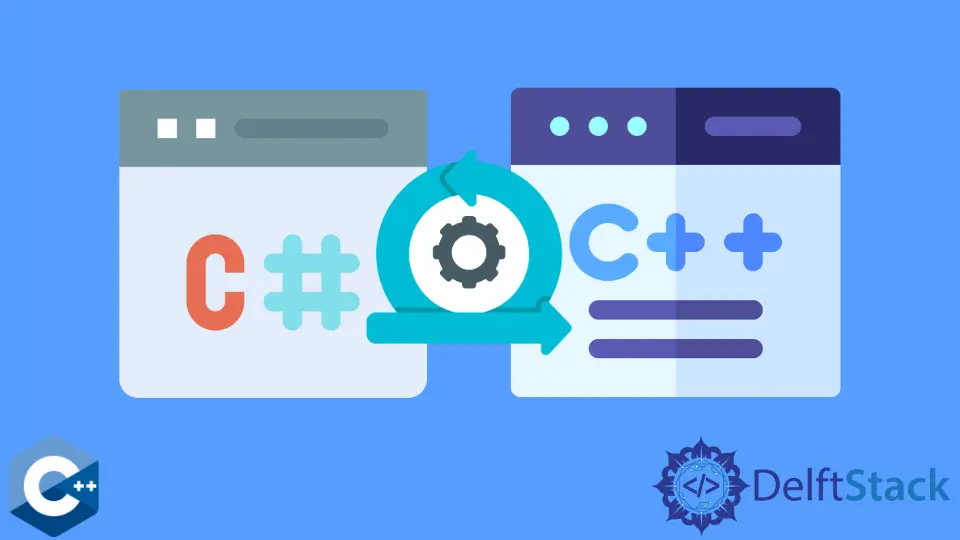
This guide will discuss how you can convert C# codes to C++.
Transforming the whole language into another language is considered nearly impossible. In this case, C# to C++ code applies to Unix, but the .NET
Framework is not available from C++ on Unix.
Convert C# Codes to C++
To convert C# to C++, we can use tools like Mono and PInvoke. These tools are worth checking out.
You can convert your code and run your current .NET
application on Unix.
Mono is also binary compatible, so you won’t need to recompile the current assembly code. Mono will allow you to run as many C# programs, but it still does not support the relative C++ extensions that enable you to work directly with the .NET
framework.
These tools will not help you all the way. You will still need to use your brain and put in your effort.
The question itself about converting C# to C++ is not complete. It needs to be more focused.
There will be some requirements where you could easily use the tools mentioned above to convert C# code to C++. But, this won’t be the case for many other requirements.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn