How to Use the const Keyword With Pointers in C++
-
Use the
const
Keyword to Denote Immutable Objects in C++ -
Using the
const
Keyword With Pointer Types in C++
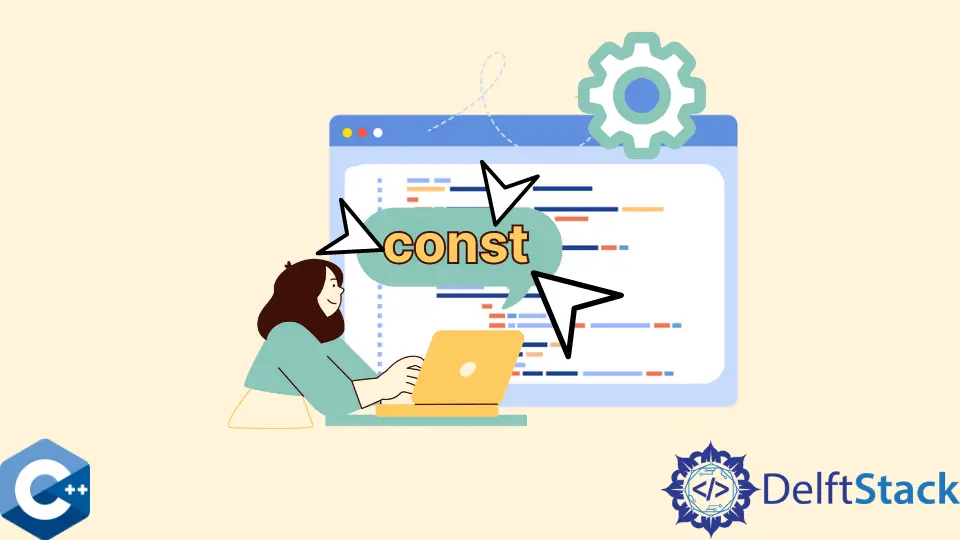
This article will show you methods of how to use the const
keyword with pointers in C++.
Use the const
Keyword to Denote Immutable Objects in C++
Generally, the const
keyword is used to specify that the given object shall be immutable throughout the program execution. The practice of naming the constant values helps keep the codebase more adjustable and easy to maintain compared to directly specifying literal values. Additionally, the const
keyword is highly utilized by the function interfaces to indicate that the given parameters are read-only and help mitigate the run-time errors to the compile-time ones.
We declare a const
object by including the keyword before the type specifier, as shown in the following code snippet. Here, you’ll observe that the s1
variable is created as the read-only string
, and the const
objects can’t be assigned a different value. Thus, they must be initialized during declaration. If we try to modify the const
object, the compiler error is thrown.
#include <iostream>
using std::cout;
using std::endl;
using std::string;
int main() {
const string s1("Hello");
string s2("Hello");
// s1 += "Joe"; // Error
s2 += "Joe";
cout << "s1: " << s1 << endl;
cout << "s2: " << s2 << endl;
return EXIT_SUCCESS;
}
Output:
s1: Hello
s2: HelloJoe
Using the const
Keyword With Pointer Types in C++
Note that when we declare a pointer object, we usually include the type of the pointed object as well. So, it becomes tricky to specify only one const
keyword as a prefix to the declaration statement. Consequently, we need to have separate const
specifiers for the pointer and the pointed object itself.
Generally, we would have three combinations of these specifiers: the first one where both the pointer and object are non-modifiable; the second combination where the pointer can’t be reassigned, but the object itself is mutable; finally, we have the variant where the pointer is mutable, and the object is not.
All three variants are declared in the next code snippet to demonstrate the corresponding syntax notation. Sometimes, it’s suggested to read these declarations from the rightmost specifier to the left, but you can also remember that the *const
declarator is used to indicate the immutable pointers.
#include <iostream>
using std::cout;
using std::endl;
using std::string;
int main() {
string s2("Hello");
const string *const s3 = &s2; // both are non-mutable
s3 = &s2; // Error
*s3 = s2; // Error
const string *s4 = &s2; // only string object is non-mutable
s4 = s3;
*s4 = s2; // Error
string *const s5 = &s2; // only pointer is non-mutable
s5 = s3; // Error
*s5 = s2;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook