Const Parameters in C++
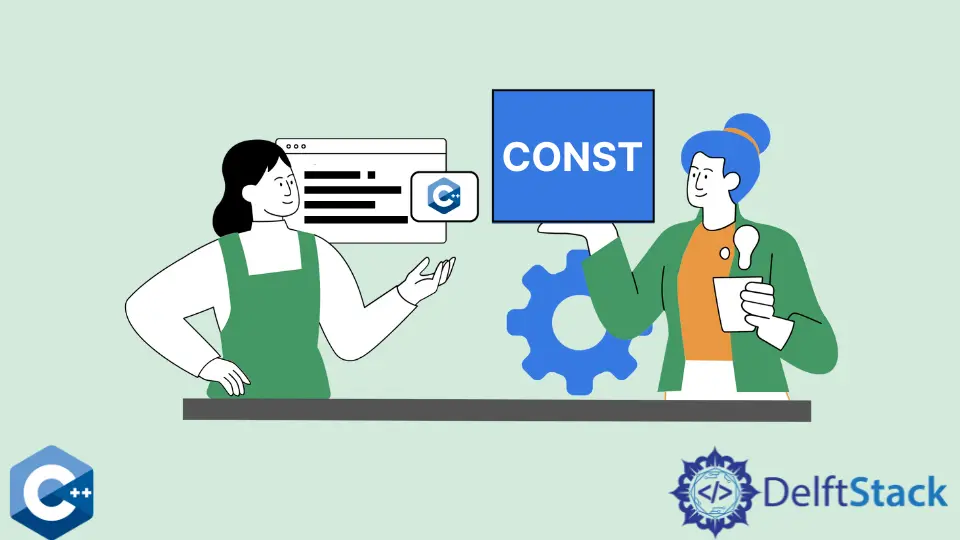
The const
keyword is used in C++ to define a variable as a constant. This keyword can be applied to any data type, but it is most often applied to pointers and references.
A constant parameter is a value that can be set and used by any function within the same scope. The parameter should be declared before any operation that uses it.
The parameter name should describe what it controls, like max_speed
or target_temperature
.
Const
Parameters in C++
We can declare a constant parameter by using the keyword const
. We cannot assign values to constants, but we can assign values to variables declared as constants.
We can also define constants in other scopes if they are not initialized with an integer literal or an expression.
This is different from a variable that has been declared using the volatile
keyword, which means it can change.
The const
keyword can be applied to variables, functions, classes, and objects. Constants cannot be changed by the user or other code with access to the same scope as the constant.
Constants are not just a good programming practice - they also offer some performance benefits for developers.
This section will go over the use of const
parameters in C++ and how they define variables as constants.
Constant Variables
The definition and initialization of constant variables must follow a set of rules.
- At the moment of the allocation, the
const
variable cannot be left uninitialized. - It can’t have a value set elsewhere in the application.
- The constant variable must be given an explicit value when it is declared.
Use Constant Parameters in C++
This section will cover the steps to use constant parameters in C++.
- Create constant parameters
- Declare the variable
- Assign a value to the constant parameter
- Include the parameter in your function call
- Use the constant parameters in your program
Let’s discuss an example.
#include <iostream>
#include <string>
using namespace std;
void myfunction(const string& x) { cout << "Who are you? " << x; }
int main() {
string a = "I'm Muhammad Adil";
myfunction(a);
}
Click here to check the code’s live demonstration.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook