Const at the End of Function in C++
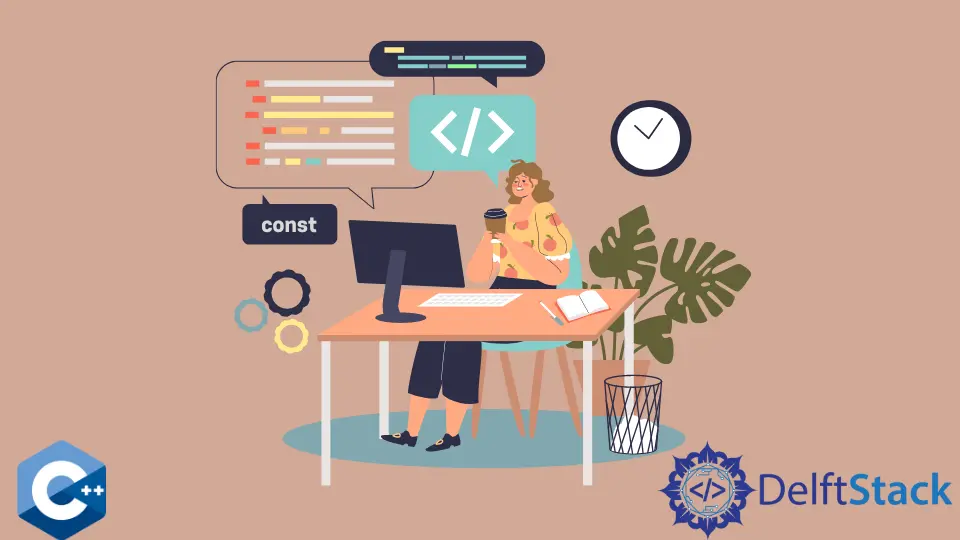
This tutorial demonstrates using the const
keyword at the end of a function in C++.
the const
Keyword at the End of a Function in C++
The const
member function are functions that are once declared and never changed or modified.
The const
at the end of the function means that the function will assume the object of which it is a member. It is constant.
By using the const
keyword at the end of the function, we force the compiler to ensure that object data is not changed or modified by the function. This concept is part of const correctness
, which is if things are working right now without changing, they will never break.
The const
functions and objects are easy and more reliable to work with in C++. The const
at the end of a function will save our code from breaking, which means we should use it repeatedly in our code.
Here is the C++ syntax for declaring the const
at the end of functions in C++.
datatype function_name const();
Now let’s try an example using the const
keyword at the end of the function.
#include <iostream>
using namespace std;
class Delftstack {
int DemoValue;
public:
Delftstack(int a = 0) { DemoValue = a; }
int PrintValue() const { return DemoValue; }
};
int main() {
const Delftstack Demo1(100);
Delftstack Demo2(76);
cout << "The Output using object Demo1 : " << Demo1.PrintValue();
cout << "The Output using object Demo2 : " << Demo2.PrintValue();
return 0;
}
As we can see, we used to const
keyword at the end of the PrintValue
function; now, whenever we create an object, it will be constant.
Output:
The Output using object Demo1 : 100
The Output using object Demo2 : 76
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook