Const Member Function in C++
- What is a Const Member Function?
- How to Declare a Const Member Function
- Benefits of Using Const Member Functions
- Conclusion
- FAQ
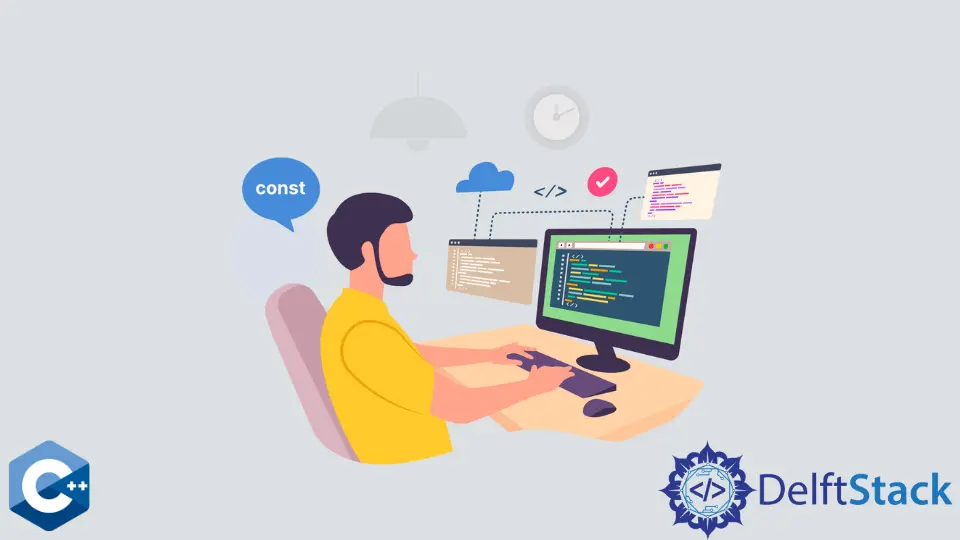
When programming in C++, understanding the concept of const member functions is crucial for writing effective and efficient code. A const member function is a special type of function that guarantees not to modify the object on which it is called. This feature not only enhances code readability but also helps prevent unintended side effects, making your code more robust.
In this article, we will explore what const member functions are, how to declare and use them, and the benefits they offer. Whether you are a beginner or an experienced C++ programmer, grasping this concept can significantly improve your coding practices.
What is a Const Member Function?
A const member function in C++ is defined with the const
keyword at the end of its declaration. This indicates that the function will not modify any member variables of the class. It is essential for functions that are intended to read data rather than change it. By enforcing this rule, you can ensure that certain functions are safe to call on const objects, enhancing the integrity of your code.
Here’s a simple example of a class with a const member function:
#include <iostream>
using namespace std;
class Example {
public:
int value;
Example(int val) : value(val) {}
int getValue() const {
return value;
}
};
int main() {
Example obj(10);
cout << "Value: " << obj.getValue() << endl;
return 0;
}
In this code, the getValue
function is a const member function. It retrieves the value of the value
member variable without modifying it. If you attempt to change any member variable inside getValue
, the compiler will generate an error.
Output:
Value: 10
Using const member functions can lead to safer and more maintainable code. They allow you to define clear interfaces for your classes, indicating which functions are meant to modify the object and which are not.
How to Declare a Const Member Function
Declaring a const member function is straightforward. You simply add the const
keyword after the function signature. Here is a more detailed example demonstrating how to declare and use a const member function:
#include <iostream>
using namespace std;
class Counter {
private:
int count;
public:
Counter() : count(0) {}
void increment() {
count++;
}
int getCount() const {
return count;
}
};
int main() {
Counter myCounter;
myCounter.increment();
cout << "Current Count: " << myCounter.getCount() << endl;
return 0;
}
In this example, getCount
is declared as a const member function. This means it can be called on a const instance of Counter
. The increment
function, on the other hand, modifies the state of the object and is not const.
Output:
Current Count: 1
By using const member functions, you can create a clear separation between modifying and non-modifying operations, which improves the overall design of your classes.
Benefits of Using Const Member Functions
Using const member functions offers several advantages. First, they enhance code safety by preventing unintended modifications to class members. This is particularly important in large codebases where side effects can be hard to track down. Second, const member functions can be called on const instances of a class, which is essential for maintaining the integrity of objects that should not change.
Consider the following example that highlights these benefits:
#include <iostream>
using namespace std;
class Circle {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double getArea() const {
return 3.14159 * radius * radius;
}
void setRadius(double r) {
radius = r;
}
};
int main() {
const Circle myCircle(5.0);
cout << "Area: " << myCircle.getArea() << endl;
// myCircle.setRadius(10.0); // This line would cause a compilation error
return 0;
}
In this example, getArea
is a const member function, allowing it to be called on a const instance of Circle
. Attempting to call setRadius
on myCircle
would result in a compilation error, ensuring that the radius remains unchanged.
Output:
Area: 78.53975
This approach not only improves code reliability but also helps other developers understand your intentions when using your classes.
Conclusion
In conclusion, const member functions are a powerful feature in C++ that contribute to safer and more maintainable code. By clearly distinguishing between functions that modify class members and those that do not, you can create more robust and understandable code. As you continue your journey in C++, integrating const member functions into your coding practices will undoubtedly enhance your programming skills and the quality of your projects.
FAQ
-
What is a const member function in C++?
A const member function is a function that is declared with theconst
keyword at the end of its declaration, indicating that it will not modify the object it is called on. -
Why should I use const member functions?
Using const member functions helps prevent unintended modifications to class members and allows these functions to be called on const instances of a class. -
Can I call a non-const member function on a const object?
No, you cannot call a non-const member function on a const object. Doing so will result in a compilation error. -
How do I declare a const member function?
To declare a const member function, simply add theconst
keyword after the function signature in the class definition. -
What happens if I try to modify a member variable inside a const member function?
If you attempt to modify a member variable inside a const member function, the compiler will generate an error, ensuring that the const contract is upheld.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn