How to Use a Conditional Operator in C++
- Understanding the Conditional Operator
- Using Conditional Operator for Multiple Conditions
- Advantages of Using the Conditional Operator
- Best Practices When Using the Conditional Operator
- Conclusion
- FAQ
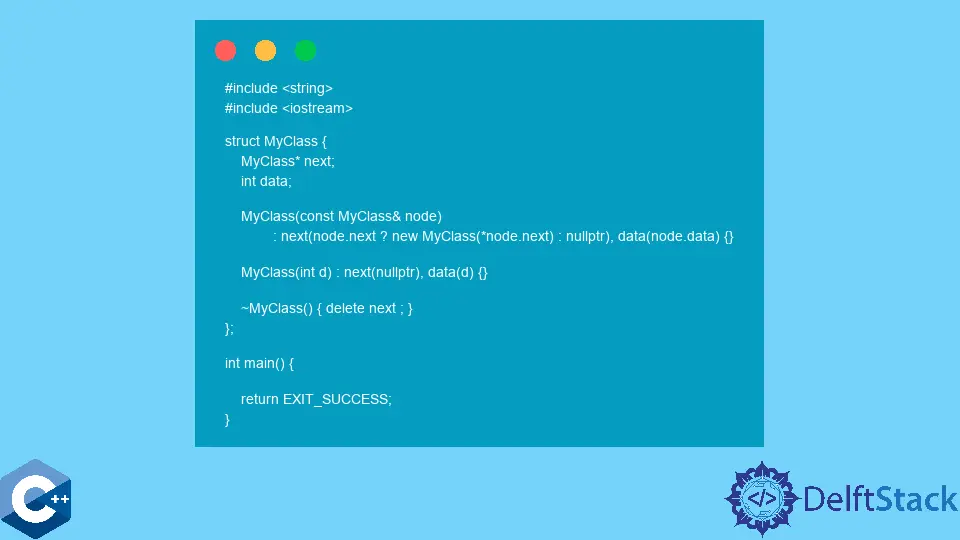
In C++, the conditional operator, also known as the ternary operator, is a powerful tool that allows you to evaluate expressions and return values based on a condition. It provides a more concise way to write simple if-else statements, making your code cleaner and more readable.
In this article, we’ll explore how to effectively use the conditional operator in C++, including examples that illustrate its functionality. Whether you’re a beginner trying to grasp the fundamentals or an experienced programmer looking to refine your skills, this guide will help you understand the nuances of the conditional operator in C++.
Understanding the Conditional Operator
The conditional operator in C++ is represented by the symbols ?
and :
. It takes three operands: a condition to evaluate, the value to return if the condition is true, and the value to return if the condition is false. The syntax looks like this:
condition ? value_if_true : value_if_false;
Here’s a simple example to illustrate its use:
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 20;
int max = (a > b) ? a : b;
cout << "The maximum value is: " << max << endl;
return 0;
}
In this code, we check if a
is greater than b
. If true, max
gets the value of a
, otherwise it gets the value of b
. The output will display the maximum value between the two variables.
Output:
The maximum value is: 20
The conditional operator is particularly useful in situations where you want to assign a value based on a simple condition without the overhead of multiple lines of code. It not only simplifies the code but also enhances readability, especially when used judiciously.
Using Conditional Operator for Multiple Conditions
While the conditional operator is great for simple conditions, it can also be nested to handle multiple conditions. This can be particularly useful when you have more than two possible outcomes. Here’s how you can do it:
#include <iostream>
using namespace std;
int main() {
int score = 85;
string grade = (score >= 90) ? "A" :
(score >= 80) ? "B" :
(score >= 70) ? "C" : "F";
cout << "Your grade is: " << grade << endl;
return 0;
}
In this example, we evaluate the score
variable and assign a grade based on its value. The nested conditional operator checks multiple ranges, allowing us to determine the grade in a compact way.
Output:
Your grade is: B
While nesting can be useful, it’s important to use it judiciously. Excessive nesting can lead to code that is difficult to read and maintain. Therefore, it’s best to keep your conditions simple and clear, ensuring that anyone reading your code can easily understand the logic.
Advantages of Using the Conditional Operator
One of the primary advantages of using the conditional operator is the reduction in code length. This can make your code cleaner and easier to read, especially for straightforward conditions. Additionally, it can improve performance slightly by reducing the number of lines the compiler has to process.
However, it’s important to strike a balance. While the conditional operator can make code more concise, overusing it or using it in complex situations can lead to confusion. Here’s a practical example that demonstrates its advantages:
#include <iostream>
using namespace std;
int main() {
int age = 18;
string eligibility = (age >= 18) ? "eligible" : "not eligible";
cout << "You are " << eligibility << " to vote." << endl;
return 0;
}
In this case, we check if age
is 18 or older to determine voting eligibility. The conditional operator allows us to do this in a single line, making it easy to follow.
Output:
You are eligible to vote.
Using the conditional operator can streamline your code and make your intentions clear. Just remember to keep readability in mind, especially for more complex logic.
Best Practices When Using the Conditional Operator
While the conditional operator can enhance your code, there are best practices to follow to ensure it remains effective. First, use it for simple conditions where the logic is clear. If you find yourself nesting multiple conditions, consider using traditional if-else statements instead. This can help maintain readability.
Here’s an example of a well-structured use of the conditional operator:
#include <iostream>
using namespace std;
int main() {
int temperature = 30;
string weather = (temperature > 25) ? "Hot" : "Cold";
cout << "The weather is: " << weather << endl;
return 0;
}
In this code, we determine if the weather is hot or cold based on the temperature. The logic is straightforward, making it a suitable candidate for the conditional operator.
Output:
The weather is: Hot
Lastly, always prioritize clarity over brevity. If using the conditional operator makes your code harder to understand, it’s better to opt for a more verbose but clearer approach. This will save time for anyone maintaining the code later, including yourself.
Conclusion
In conclusion, the conditional operator in C++ is a valuable tool for simplifying code and enhancing readability. By understanding its syntax and appropriate use cases, you can write cleaner, more efficient programs. Remember to keep conditions simple and prioritize clarity to ensure your code remains maintainable. With practice, you’ll become adept at using the conditional operator effectively, making your C++ programming experience smoother and more enjoyable.
FAQ
-
What is the syntax of the conditional operator in C++?
The syntax is condition ? value_if_true : value_if_false. -
Can I nest conditional operators?
Yes, you can nest them, but it’s best to keep it simple for readability. -
When should I avoid using the conditional operator?
Avoid it for complex conditions where if-else statements are clearer. -
Does using the conditional operator improve performance?
It can slightly improve performance by reducing code length, but readability should be prioritized. -
Is the conditional operator the same as an if-else statement?
They achieve similar outcomes, but the conditional operator is more concise for simple conditions.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook