How to Compile C++ Codes in macOS
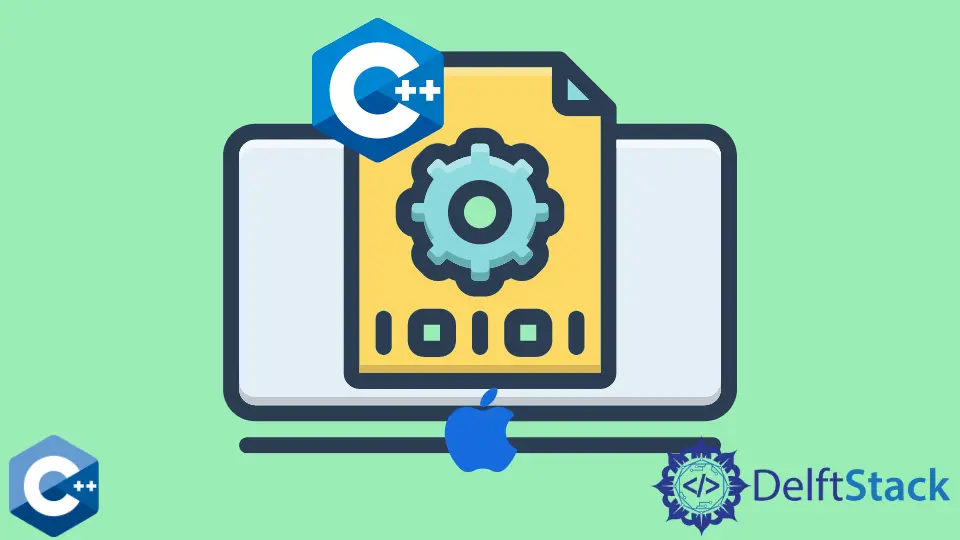
This article contains information about the C++ compiler for macOS X. We will discuss how we compile and run code using the g++ compiler using the command line interface (i.e., terminal).
GNU has two compilers, GCC and g++, for compiling C and C++ codes. GCC is mainly used for compiling C language codes, and g++ is used to compile C++ codes.
If we are using the g++ compiler to link object files, it links them automatically in the standard (STD) C++ libraries.
Install the G++ Compiler to Compile C++ Codes in macOS
To install the G++ compiler on macOS, you need to open the terminal window and type in the following command:
onworks@onworks:/$ g++
If your package is not already installed, then the following window will appear:
Now, to install, we use the following command:
onworks@onworks:/$ sudo apt-get install g++
This command will start the installation of the g++ compiler after you permit by pressing the Y
key, as shown in the image below:
Installation of the g++ compiler is now complete. Now, you can compile your C++ programs on your macOS.
Compile and Run C++ Codes in macOS
You can now create a program named firstProgram.cpp
in any text editor like this code:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World" << endl;
return 0;
}
To compile this code, open the file location in the terminal and type in the following command:
onworks@onworks:/$ g++ firstProgram.cpp -o firstProgram
This command will create an executable .exe
file named firstProgram.exe
.
The -o
flag is used to name the executable file by your choice. If you don’t use -o
, it will create an executable by the name a.out
.
To run that executable, use the following command:
onworks@onworks:/$ ./firstProgram
Output:
Hello World
So now, we have compiled and run a C++ code on macOS.
We can also use different macOS compilers to compile and run C++ codes.
- XCode
- Netbeans
- cLion
- Qt Creator
- Visual Studio for OS X
These compilers provide a good code editor with IntelliSense and code indentation options. They provide very efficient debugging tools to trace down the errors in your code.
These compilers provide you with a manageable project management feature. You can save all the source files of your project and the other files related to your project, for example, database files or image files, etc.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn