Call by Reference vs Call by Value in C++
-
Use
&
Symbol to Call Function by Reference in C++ - Use Plain Function Definition to Call Functions by Value in C++
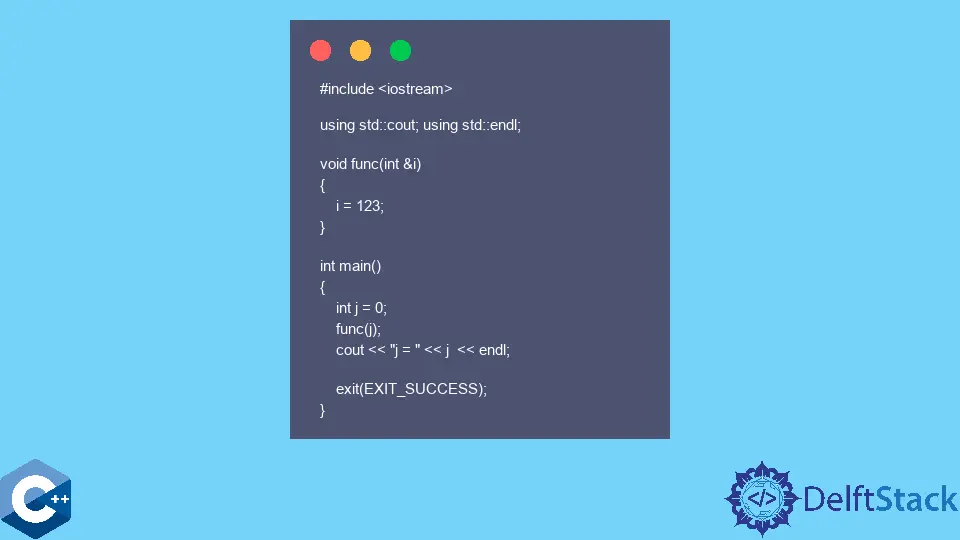
This article will explain several methods of how to call by reference vs call by value in C++.
Use &
Symbol to Call Function by Reference in C++
Function arguments are the core part of the function definition, as they are the values that initialize the function’s parameters. When the parameter is a reference, it’s bound to the argument and the value of the argument is not copied. The corresponding behavior is called passing by the reference or that the function is called by reference. Note that this method is more commonly used when relatively larger objects need to be passed between the functions as it saves extra resources held up by the copying process.
The operations that get conducted on the object inside the function will modify the variable’s original memory location, which is a similar method to passing the pointer to the object. In the following example, we demonstrate the func
function that takes a single int
type parameter and assigns the 123
value. The i
symbol is merely the alias name for the original j
object from the main
program. Mind though that, &
symbol is quite overloaded in C++ and should not be confused with address-of operator functionality.
#include <iostream>
using std::cout;
using std::endl;
void func(int &i) { i = 123; }
int main() {
int j = 0;
func(j);
cout << "j = " << j << endl;
exit(EXIT_SUCCESS);
}
Output:
j = 123
Use Plain Function Definition to Call Functions by Value in C++
Calling the function by value is often said for the behavior when the given function does copy the parameter and stores it as a separate object in the respective scope. The corresponding notation does not require any distinct symbols, but the arguments are specified as plain variables. Consequently, the function block scope has a separate object, and modifications to it do not affect the original variable from the main
function. The most common practice would be to employ calling by value
method when the passed arguments are built-in types or small objects easily copied between functions.
#include <iostream>
using std::cout;
using std::endl;
void func2(int i) { i = 123; }
void func(int &i) { i = 123; }
void func(int *ip) {
*ip = 123;
ip = nullptr;
}
int main() {
int j = 0;
func(j);
cout << "j = " << j << endl;
j = 0;
func2(j);
cout << "j = " << j << endl;
j = 0;
func(&j);
cout << "j = " << j << endl;
exit(EXIT_SUCCESS);
}
Output:
j = 123
j = 0
j = 123
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook