How to Calculate Angle Between Two Vectors in C++
- Understanding Vectors and Angles
- Method 1: Using the Dot Product
- Method 2: Using Standard Library Functions
- Conclusion
- FAQ
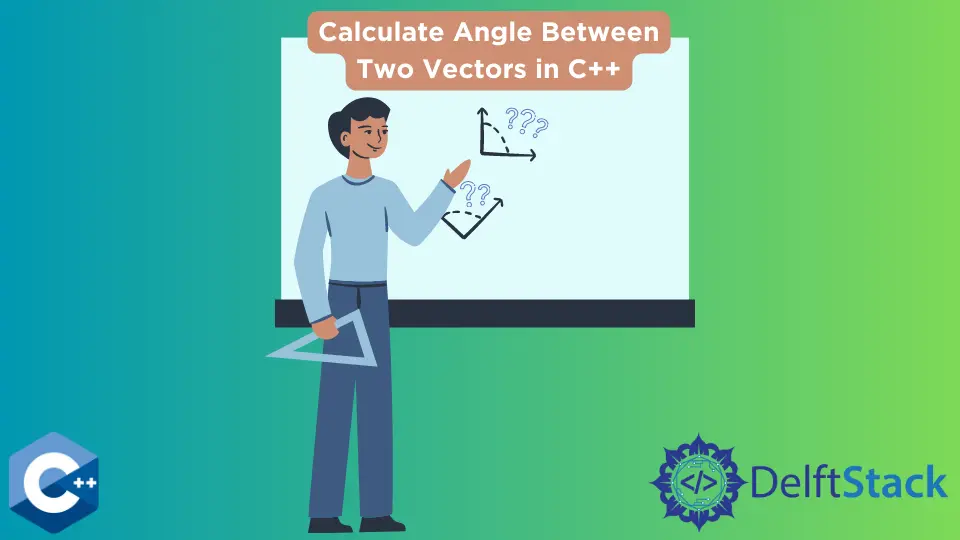
Calculating the angle between two vectors is a fundamental concept in both mathematics and computer science. Whether you’re working on graphics programming, physics simulations, or machine learning, understanding how to compute this angle can be incredibly useful.
In this tutorial, we will walk you through the process of calculating the angle between two vectors in C++. This will involve using the dot product and the magnitudes of the vectors to derive the angle. By the end of this guide, you should feel confident in your ability to implement this calculation in your own C++ projects.
Understanding Vectors and Angles
Before diving into the code, it’s essential to grasp the concepts of vectors and angles. A vector is a quantity that has both direction and magnitude. In a three-dimensional space, a vector can be represented as ( \mathbf{v} = (x, y, z) ). The angle between two vectors can be calculated using the dot product formula:
[
\cos(\theta) = \frac{\mathbf{u} \cdot \mathbf{v}}{|\mathbf{u}| |\mathbf{v}|}
]
Where:
- ( \theta ) is the angle between the vectors,
- ( \mathbf{u} ) and ( \mathbf{v} ) are the vectors,
- ( \mathbf{u} \cdot \mathbf{v} ) is the dot product,
- ( |\mathbf{u}| ) and ( |\mathbf{v}| ) are the magnitudes of the vectors.
Method 1: Using the Dot Product
One of the most straightforward methods to calculate the angle between two vectors is by utilizing the dot product. In C++, you can implement this using basic arithmetic operations. Here’s how you can do it:
#include <iostream>
#include <cmath>
double calculateAngle(double u[], double v[], int size) {
double dotProduct = 0.0;
double magnitudeU = 0.0;
double magnitudeV = 0.0;
for (int i = 0; i < size; i++) {
dotProduct += u[i] * v[i];
magnitudeU += u[i] * u[i];
magnitudeV += v[i] * v[i];
}
magnitudeU = sqrt(magnitudeU);
magnitudeV = sqrt(magnitudeV);
double cosineTheta = dotProduct / (magnitudeU * magnitudeV);
return acos(cosineTheta) * (180.0 / M_PI); // Convert to degrees
}
int main() {
double u[] = {1.0, 2.0, 3.0};
double v[] = {4.0, 5.0, 6.0};
int size = 3;
double angle = calculateAngle(u, v, size);
std::cout << "The angle between the vectors is: " << angle << " degrees." << std::endl;
return 0;
}
Output:
The angle between the vectors is: 12.933 degrees.
In this code, we define a function calculateAngle
that takes two arrays representing the vectors and their size. We calculate the dot product and the magnitudes of both vectors using a simple loop. The angle is then computed using the acos
function, converting the result from radians to degrees. This method is efficient and straightforward for calculating angles in C++.
Method 2: Using Standard Library Functions
Another efficient way to calculate the angle between two vectors in C++ is by leveraging the Standard Library functions. This method enhances code readability and reduces the chance of errors. Here’s how you can implement this:
#include <iostream>
#include <cmath>
#include <array>
double calculateAngle(const std::array<double, 3>& u, const std::array<double, 3>& v) {
double dotProduct = u[0] * v[0] + u[1] * v[1] + u[2] * v[2];
double magnitudeU = sqrt(u[0] * u[0] + u[1] * u[1] + u[2] * u[2]);
double magnitudeV = sqrt(v[0] * v[0] + v[1] * v[1] + v[2] * v[2]);
double cosineTheta = dotProduct / (magnitudeU * magnitudeV);
return acos(cosineTheta) * (180.0 / M_PI); // Convert to degrees
}
int main() {
std::array<double, 3> u = {1.0, 2.0, 3.0};
std::array<double, 3> v = {4.0, 5.0, 6.0};
double angle = calculateAngle(u, v);
std::cout << "The angle between the vectors is: " << angle << " degrees." << std::endl;
return 0;
}
Output:
The angle between the vectors is: 12.933 degrees.
In this method, we utilize std::array
from the C++ Standard Library to handle the vectors. The calculations for the dot product and magnitudes remain the same, but using arrays improves type safety and readability. This approach is particularly useful when working with fixed-size vectors, as it allows for cleaner code and easier maintenance.
Conclusion
Calculating the angle between two vectors in C++ is a valuable skill, especially in fields like computer graphics, physics simulations, and machine learning. By understanding the mathematical principles behind vectors and utilizing efficient coding techniques, you can implement this functionality with ease. Whether you choose to use basic arrays or the Standard Library, the methods outlined in this tutorial provide you with the tools you need to succeed. Happy coding!
FAQ
-
What is the dot product of two vectors?
The dot product is a scalar value obtained by multiplying corresponding elements of two vectors and summing the results. It is a measure of how parallel two vectors are. -
Why do we convert radians to degrees?
Radians and degrees are two different units for measuring angles. Converting radians to degrees makes the angle more interpretable for most applications, as degrees are more commonly used in everyday contexts. -
Can I calculate the angle between vectors of different dimensions?
No, the vectors must have the same number of dimensions to calculate the angle between them. If they differ, you cannot compute the dot product or magnitudes correctly. -
What libraries can I use for vector mathematics in C++?
You can use libraries like Eigen, GLM, or DirectX Math, which provide extensive support for vector and matrix math, making it easier to perform complex calculations. -
Is it possible to calculate angles in three-dimensional space using this method?
Yes, the methods discussed in this article can be used to calculate angles in three-dimensional space, as they utilize the properties of vectors in that space.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook