Breaks in C++ Switch Statement
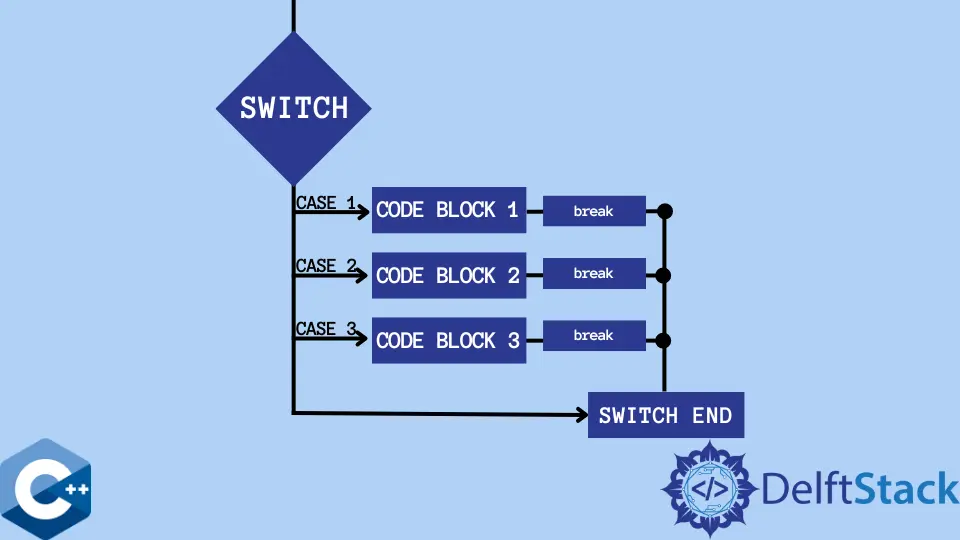
The break
statement in C and C++ is used to stop a loop from iterating if the required condition is met within the code blocks of the switch
statement.
If the break
statement is not used, the program will continue to execute until the end of the switch
statement is reached.
First, we must understand how a switch
statement works.
At the beginning of the switch
statement, we have to provide an expression, then the program will go through each case
in the switch
statement, and if it finds the case that matches the expression that we provided, it will execute that case
.
If the above process cannot match the expression, the program will jump out of the switch
statement and execute the default
statement.
the switch
Statement With break
in C++
#include <iostream>
using namespace std;
int main() {
int rating = 2;
switch (rating) {
case 1:
cout << "Rated as 1. ";
break;
case 2:
cout << "Rated as 2. ";
break;
case 3:
cout << "Rated as 3. ";
break;
case 4:
cout << "Rated as 4. ";
break;
case 5:
cout << "Rated as 5. ";
break;
}
return 0;
}
The expression here is rating = 2
. What the program does here is that it goes through each case
one by one and checks for a potential match for the expression provided to stop executing the loop any further and terminates it, giving us the output below.
Rated as 2.
the switch
Statement Without break
in C++
Let’s run the same code again, but this time by removing the break
statements after each case.
#include <iostream>
using namespace std;
int main() {
int rating = 2;
switch (rating) {
case 1:
cout << "Rated as 1. ";
case 2:
cout << "Rated as 2. ";
case 3:
cout << "Rated as 3. ";
case 4:
cout << "Rated as 4. ";
case 5:
cout << "Rated as 5. ";
}
return 0;
}
Output:
Rated as 2. Rated as 3. Rated as 4. Rated as 5.
You can see that without the break
statement, the program prints the value of each case
even after the requirement is fulfilled.
The break
statement is used for situations in which the number of times a loop should iterate is unknown or when the loop meets a certain predefined condition.
The switch
statement body will not get executed when there is no default
statement and no case
match in the statement body. There can only be one default
statement anywhere inside the switch
statement body.
Finally, the break
statement is also used with doing, for, and while loops. In those situations, the break
statement forces the program to exit the loop when the particular criteria are met.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.