break vs continue in C++
- Understanding the Break Statement
- Exploring the Continue Statement
- When to Use Break and Continue
- Conclusion
- FAQ
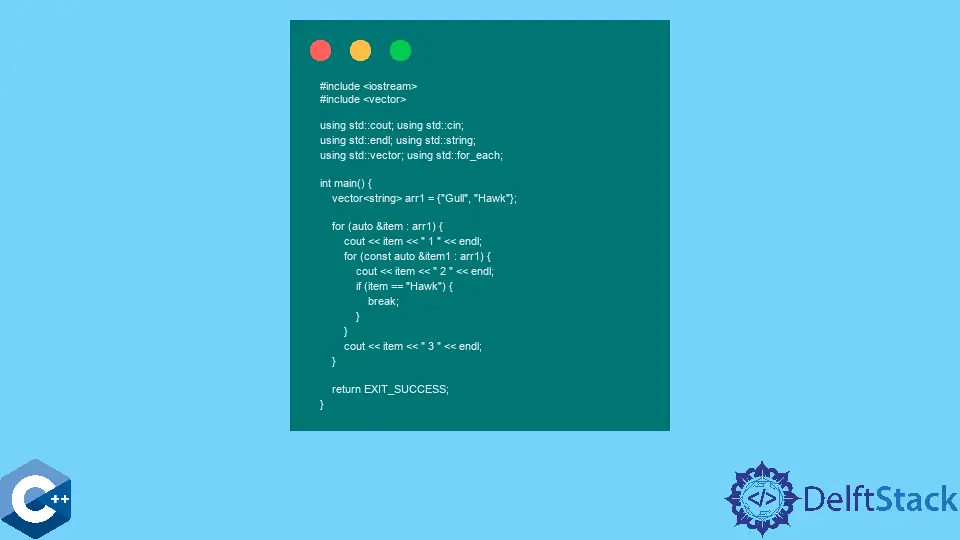
In C++, controlling the flow of your program is essential for creating efficient and logical code. Two key statements that help manage this flow are break
and continue
. While both are used to alter the execution of loops, they serve distinct purposes that can significantly impact how your program operates.
In this article, we will explore the differences between break
and continue
, how to use them effectively, and provide clear examples to illustrate their functionality. Whether you’re a beginner or looking to refine your C++ skills, understanding these control statements is crucial for writing cleaner, more efficient code.
Understanding the Break Statement
The break
statement is used to terminate a loop prematurely. When a break
is encountered, the control exits the loop immediately, and the program continues with the next statement following the loop. This can be particularly useful when you want to stop the execution based on a certain condition.
Here’s a simple example of using break
in a C++ program:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break;
}
cout << i << " ";
}
return 0;
}
Output:
1 2 3 4
In this example, the loop is set to run from 1 to 10. However, when i
reaches 5, the break
statement is executed. This causes the loop to terminate immediately, and the program outputs the numbers 1 through 4. Understanding how to effectively use the break
statement can help you manage complex loops and improve your program’s efficiency.
Exploring the Continue Statement
In contrast to break
, the continue
statement is used to skip the current iteration of a loop and move directly to the next iteration. When continue
is executed, the remaining code within the loop for that iteration is skipped, but the loop itself continues to run. This can be useful when you want to ignore certain values without terminating the entire loop.
Here’s an example demonstrating the use of continue
:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
continue;
}
cout << i << " ";
}
return 0;
}
Output:
1 2 3 4 6 7 8 9 10
In this code, the loop iterates from 1 to 10. When i
equals 5, the continue
statement is executed, skipping the output of 5. As a result, the program prints all numbers from 1 to 10, except for 5. This demonstrates how continue
allows for more granular control over loop execution, enabling you to bypass specific conditions without breaking out of the loop entirely.
When to Use Break and Continue
Choosing between break
and continue
depends on the specific requirements of your program. Use break
when you need to exit a loop based on a condition that makes further iterations unnecessary. On the other hand, use continue
when you want to skip certain iterations while still processing the rest of the loop.
For instance, if you’re searching for a specific value in a list and want to stop searching as soon as you find it, break
is the appropriate choice. Conversely, if you’re processing a list and need to skip over invalid entries while still checking the rest, continue
is the better option.
Conclusion
In summary, both break
and continue
are essential control flow statements in C++ that serve different purposes. The break
statement allows you to exit loops prematurely, while continue
enables you to skip specific iterations without terminating the loop. Understanding the nuances of these statements will help you write cleaner and more efficient code. As you continue to practice and implement these concepts, you’ll find that mastering control flow is key to becoming a proficient C++ programmer.
FAQ
-
What is the main difference between break and continue in C++?
The main difference is thatbreak
terminates the loop entirely, whilecontinue
skips the current iteration and continues with the next one. -
Can I use break and continue in nested loops?
Yes, you can use bothbreak
andcontinue
in nested loops. However,break
will only exit the innermost loop unless you use labeled breaks.
-
Is it possible to use break and continue in switch statements?
Yes, you can usebreak
in switch statements to exit the switch case. However,continue
is not applicable in switch statements. -
Are there any alternatives to break and continue in C++?
Alternatives include using flags or conditions to control loop execution, butbreak
andcontinue
are the most straightforward methods for managing loop flow. -
Can I use break and continue in
while
loops?
Absolutely! Bothbreak
andcontinue
can be used inwhile
loops just as effectively as infor
loops.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook