How to Read and Write Bits to a File in C++
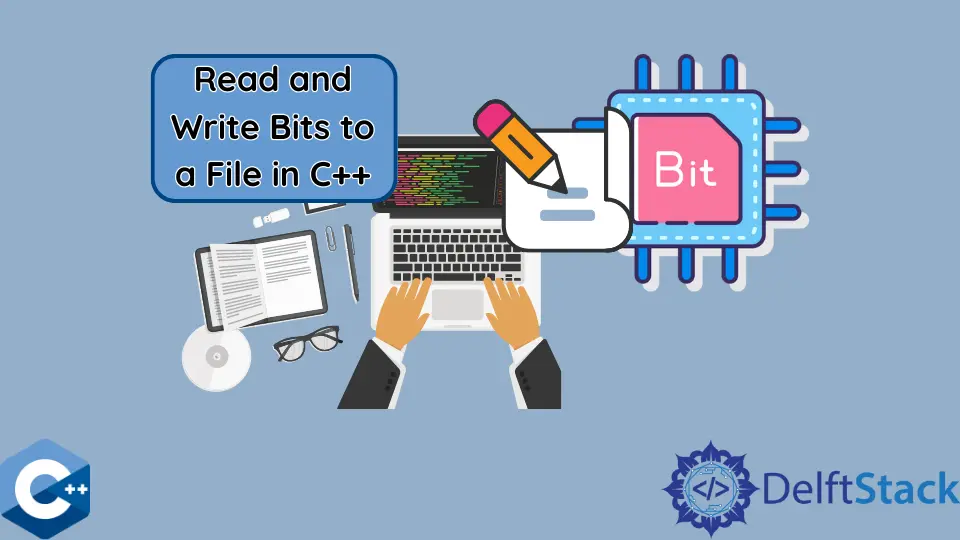
In C++, processes like the compression of a text file require reading and writing the bits to a file. In this tutorial, you will learn how to read or write bits to a file without any errors in C++.
The istream
and ostream
, as a destructor, play a crucial part when reading and writing bits to a file is required.
However, it may require writing bits into a binary output file while encoding through the Huffman
tree in Huffman
coding, and instead of using the streams, you can pack the Booleans into chunks of 8 bits and then write the bytes.
Use iStream
and oStream
to Read and Write Bits to a File in C++
The BitInputStream
class contains the iStream
and oStream
features to read and write bits. As the ifstream
and ofstream
can open binary files by attaching streams to a physical file name, they are also crucial to read or write bits to a file.
Additionally, open member functions of a target BitInputStream
class can provide arguments (optional arguments) that are not described in common.
The ostream
is one of the open member functions that causes n
bytes to be written from the memory location and transfers the pointer ahead of the n
bytes.
The fstream
stream class is highly functional than any other stream class because of its ability to both read and write from/to files.
It’s not important for file streams to read and write operations in binary only because they are opened in binary mode; instead, they can perform read/write operations of any form of consideration.
#include <fstream>
#include <iostream> // reflects `istream` and `ostream`
// optional
using namespace std;
// struct declaration
struct army_per {
// an entity that reflects three attributes
int ref_no;
string rank, name;
};
// primary class
int main() {
// declaration of `ofstream` for read/write `army_per` file
ofstream wf("army_per.dat", ios::out | ios::binary);
if (!wf) {
cout << "Unable to open the file!" << endl;
return 1;
}
// entity declaration
army_per soldier[2];
// first entry
soldier[0].ref_no = 1;
soldier[0].name = "Rob";
soldier[0].rank = "Captain";
// second entry
soldier[1].ref_no = 2;
soldier[1].name = "Stannis";
soldier[1].rank = "Major";
for (int i = 0; i < 2; i++) wf.write((char *)&soldier[i], sizeof(army_per));
wf.close();
if (!wf.good()) {
cout << "Error: bits writing time error!" << endl;
return 1;
}
ifstream rf("army_per.dat", ios::out | ios::binary);
if (!rf) {
cout << "File is not found!" << endl;
return 1;
}
army_per rstu[2];
for (int i = 0; i < 2; i++) rf.read((char *)&rstu[i], sizeof(army_per));
rf.close();
if (!rf.good()) {
cout << "Error: bits reading time error occured!" << endl;
return 1;
}
cout << "Army Div-32 details:" << endl;
for (int i = 0; i < 2; i++) {
// access the elements of the object and output the result of each
// individual
cout << "Reference No: " << soldier[i].ref_no << endl;
cout << "Name: " << soldier[i].name << endl;
cout << "Rank: " << soldier[i].rank << endl;
cout << endl;
}
return 0;
}
Output:
Army Div-32 details:
Reference No: 1
Name: Rob
Rank: Captain
Reference No: 2
Name: Stannis
Rank: Major
Generally, it’s believed that cin
and cout
belong to ostream
in C++, but the cin
object (as a global object) belongs to the istream
class.
Furthermore, the file stream, which includes; ifstream
and ofstream
, are inherited from istream
and ostream
, respectively.
Programmers should be aware that the buffer is always greater than the data it is supposed to hold. In C++, the chances of decreasing your program’s reliability can increase in case of un-checked or solved errors, and make sure that no file operations cause the program to stop.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub