C++ STL Binary Search
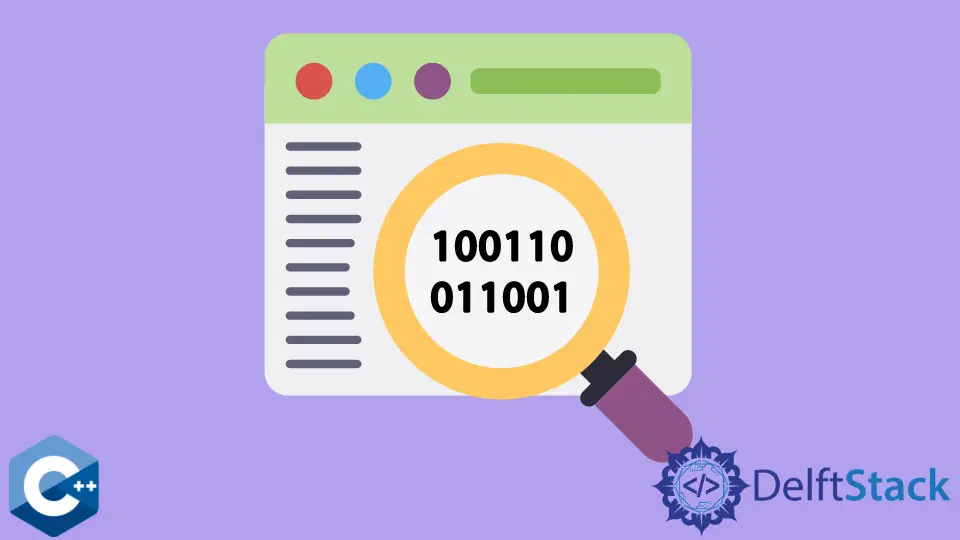
C++ provides us with a ready-to-use function binary_search()
so that we don’t have to implement the function ourselves. It is a very simple to use and efficiently implemented method and not prone to errors.
C++ STL binary_search()
Overview
Syntax
DEFAULT
: template <class ForwardIterator, class T>
bool
binary_search(ForwardIterator first, ForwardIterator last, const T& val);
CUSTOM COMPARISON FUNCTION
: template <class ForwardIterator, class T, class Compare>
bool
binary_search(ForwardIterator first, ForwardIterator last, const T& val,
Compare comp);
Here, T
can be any of the following: int
, float
, short
, long
, byte
, char
, double
, and even a user-defined Object
as well.
It checks whether an element inside [first, last)
matches the target element X
using the binary search algorithm. By default, it uses the less-than operator to compare elements, but we can also provide our own custom comp
as described in the second template given above.
Parameters
first |
A forward iterator pointing towards the first element in the given array range. |
last |
A forward iterator pointing towards the last element in the given array range. |
comp |
A user-defined binary predicate function that accepts two forward iterators as arguments and returns true if the two arguments are present in the correct order. It does not modify any arguments and follows the strict weak ordering to order the elements. |
val |
The target element we are searching inside the given array range. |
Return
If it finds the target element, then it returns true; else it returns false.
C++ Program for Binary Search
#include <bits/stdc++.h>
using namespace std;
int main() {
vector<int> v = {1, 2, 3, 4, 5, 6};
if (binary_search(v.begin(), v.end(), 5)) {
cout << "Element found" << endl;
} else {
cout << "Element not found" << endl;
}
return 0;
}
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn