How to wait Function in C
-
Use the
wait
Function to Wait for State Change in Child Processes in C -
Use the
waitpid
Function to Wait for State Change in Specific Child Process in C
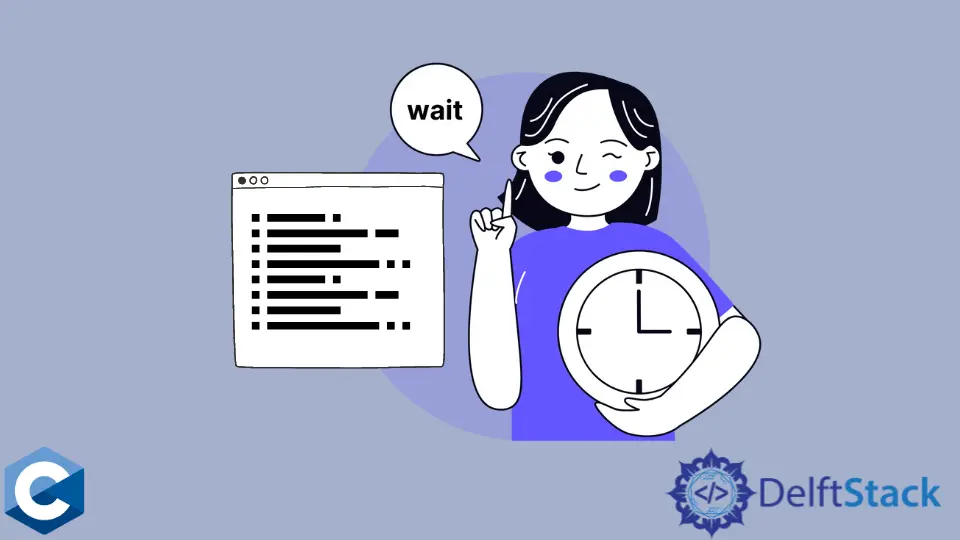
This article will demonstrate multiple methods about how to use the wait
function in C.
Use the wait
Function to Wait for State Change in Child Processes in C
The wait
function is a wrapper for POSIX compliant system call, defined in <sys/wait.h>
header file. The function is used to wait for program state changes in children processes and retrieve the corresponding information. wait
is usually called after the fork
system call that creates a new child process. wait
call suspends the calling program until one of its children processes terminate.
The user should structure the code so that there are two different paths for a calling process and the child process. It is usually accomplished with the if...else
statement that evaluates the fork
function call’s return value. Note that fork
returns child process ID, a positive integer, in the parent process and returns 0 in the child process. fork
will return -1 if the call fails.
#include <stdio.h>
#include <stdlib.h>
#include <sys/wait.h>
#include <unistd.h>
int main() {
pid_t c_pid = fork();
if (c_pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (c_pid == 0) {
printf("printed from child process %d", getpid());
exit(EXIT_SUCCESS);
} else {
printf("printed from parent process %d\n", getpid());
wait(NULL);
}
exit(EXIT_SUCCESS);
}
Use the waitpid
Function to Wait for State Change in Specific Child Process in C
waitpid
is a slightly enhanced version of the wait
function that provides the feature to wait for the specific child process and modify return triggering behavior. waitpid
can return if the child process has been stopped or continued additionally to the case when the child is terminated.
In the following example, we call the pause
function from the child process, which goes to sleep until the signal is received. On the other hand, the parent process calls the waitpid
function and suspends the execution until the child returns. It also uses macros WIFEXITED
and WIFSIGNALED
to check if the child is terminated normally or terminated by the signal, respectively, and then print the corresponding status message to the console.
#include <stdio.h>
#include <stdlib.h>
#include <sys/wait.h>
#include <unistd.h>
int main() {
pid_t child_pid, wtr;
int wstatus;
child_pid = fork();
if (child_pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (child_pid == 0) {
printf("Child PID is %d\n", getpid());
pause();
_exit(EXIT_FAILURE);
} else {
wtr = waitpid(child_pid, &wstatus, WUNTRACED | WCONTINUED);
if (wtr == -1) {
perror("waitpid");
exit(EXIT_FAILURE);
}
if (WIFEXITED(wstatus)) {
printf("exited, status=%d\n", WEXITSTATUS(wstatus));
} else if (WIFSIGNALED(wstatus)) {
printf("killed by signal %d\n", WTERMSIG(wstatus));
}
}
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook