How to Truncate String in C
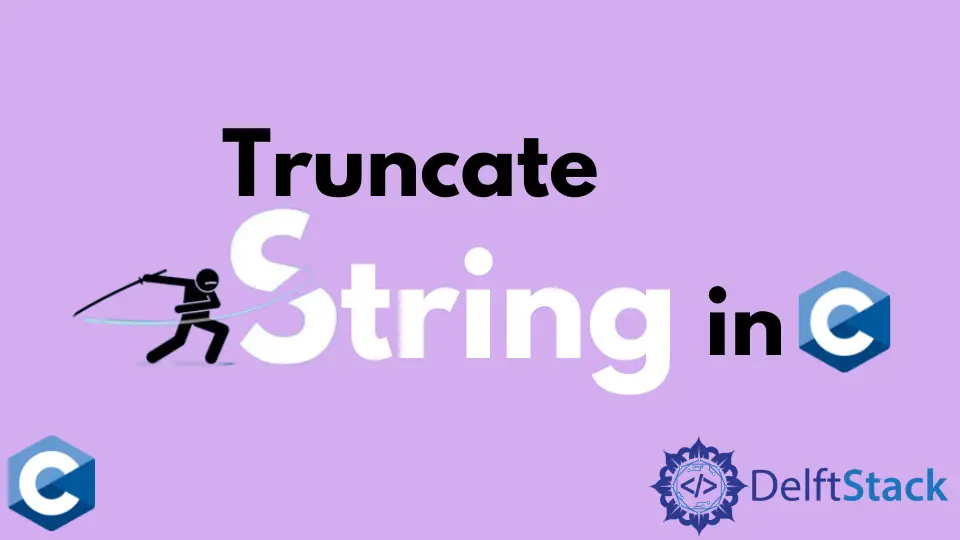
This article will introduce multiple methods about how to truncate string in C.
Use Custom Function with Pointer Arithmetic to Truncate String
Since the strings in C are just the character arrays terminated with null byte - \0
, we can implement a custom function that moves the current pointer to the beginning of the string by the given number of places and return a new pointer value.
Note though, there are two issues; the first is we need to have the option to truncate the given string from left or right and the second is that moving the pointer is not enough from the string’s right side as the null byte needs to be inserted to denote the ending. Thus, we define truncString
function that takes the string and several characters to truncate from the string. The number can be negative, indicating the side from which to remove the given number of chars
. Next, we retrieve the string length using the strlen
function, which implies that the user is responsible for passing the valid string. Then, we compare the length with the number of characters to be truncated and then move on to conduct pointer manipulation.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *truncString(char *str, int pos) {
size_t len = strlen(str);
if (len > abs(pos)) {
if (pos > 0)
str = str + pos;
else
str[len + pos] = 0;
}
return str;
}
int main(void) {
char *str1 = "the string to be truncated";
printf("%s\n", str1);
printf("%s \n", truncString(strdupa(str1), 4));
printf("%s \n", truncString(strdupa(str1), -4));
exit(EXIT_SUCCESS);
}
Output:
the string to be truncated
string to be truncated
the string to be trunc
We just assign the 0
value to the character position calculated by subtracting the length of the string and the passed number. Thus, we moved the ending of the string, and printing its value can be done with the old pointer.
Alternatively, we can implement the similar function truncString2
with the same prototype but truncates the string to the number of characters passed as the second argument. The sign of the number indicates the side from which to form the new string, namely, the positive integer denotes the left side and the negative - the opposite.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *truncString2(char *str, int pos) {
size_t len = strlen(str);
if (len > abs(pos)) {
if (pos > 0)
str[pos] = 0;
else
str = &str[len] + pos;
} else {
return (char *)NULL;
}
return str;
}
int main(void) {
char *str2 = "temporary string variable";
printf("%s\n", str2);
printf("%s \n", truncString2(strdupa(str2), 6));
printf("%s \n", truncString2(strdupa(str2), -6));
exit(EXIT_SUCCESS);
}
Output:
the string to be truncated
string to be truncated
the string to be trunc
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook