How to Create a Table in C
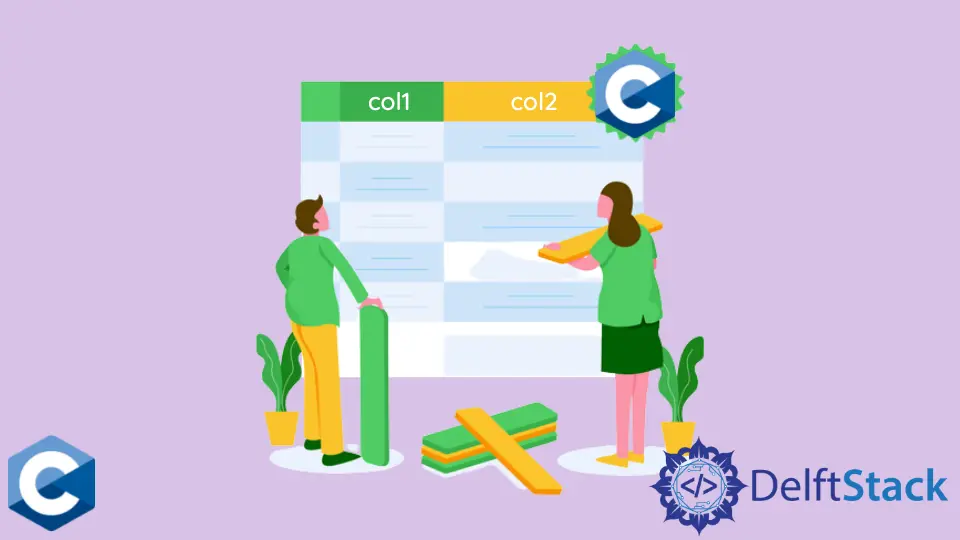
This tutorial will discuss using arrays to create tables in C.
Create a Table in C
Tables are used to store data in the form of rows and columns and are used in data analysis and research. We can use arrays to create tables in the C language.
A table has two dimensions, row and column, and to create a table, we have to use a 2-dimensional array. In a 2-dimensional array, the first dimension represents rows and the second dimension represents columns.
To create an array in C, we can use the syntax below:
dataType array_name[size] = {data};
The above syntax can be used to create a one-dimensional array or vector. The data type of the array can be integer, string, or any other data type, and we can give any name to the array.
The array name should not be the same as any predefined functions of C like printf()
, which will create problems. The size parameter is optional, and it should be equal to the total number of array elements.
We can also create a one-dimensional array or vector without passing the size in the array. For example, let’s create an integer array in C.
See the code below.
#include <stdio.h>
int main() {
int MyArray[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
printf("%d", MyArray[i]);
}
return 0;
}
Output:
12345
In the above code, we created an array of five elements and used the for
loop and the printf()
function to print the elements of the array. We used %d
inside the printf()
function because the array elements are integers.
To create a table, we have to create a 2-dimensional array using the below syntax:
dataType array_name[row][col] = {{row1}, {row2}, {rowN}};
In the above syntax, we must pass the number of rows and columns to create a 2-dimensional array or table. For multidimensional arrays, we must pass the bounds for each dimension except for the first one.
We can pass each table row inside the array separated by a comma. For example, let’s create a 2-dimensional array or table in C.
See the code below.
#include <stdio.h>
int main() {
int My_table[3][5] = {{0, 1, 2, 3, 4}, {1, 1, 2, 31, 41}, {0, 1, 2, 2, 4}};
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 5; ++j) {
printf("%d ", My_table[i][j]);
}
printf("\n");
}
return 0;
}
Output:
0 1 2 3 4
1 1 2 31 41
0 1 2 2 4
In the above code, we created a table with 3 rows and 5 columns, and we used two for
loops, one for rows and one for columns, to print the table. We can also create a table of other data types like char, float, or other data types.