How to Use the strsep Function in C
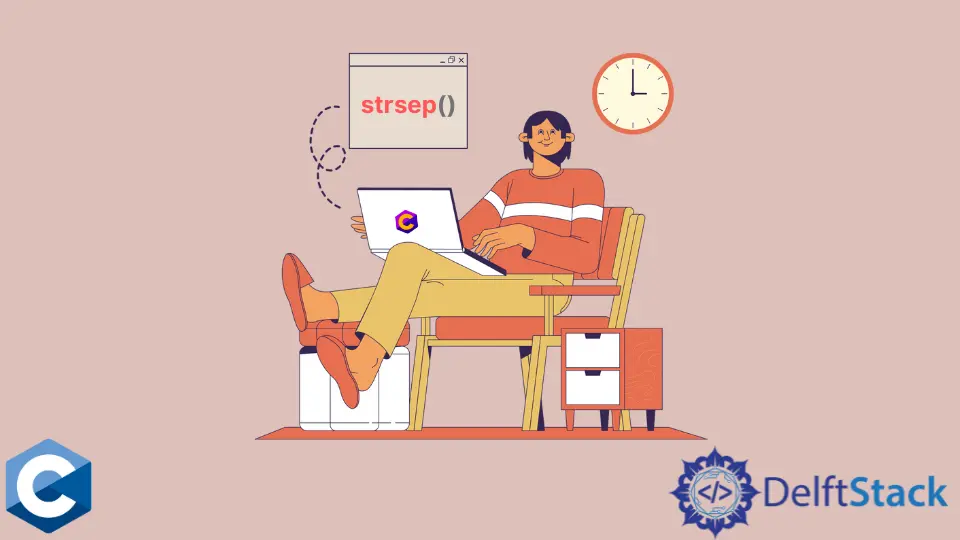
This article will demonstrate multiple methods about how to use the strsep
function in C.
Use the strsep
Function to Find the Given Token in the String
strsep
is part of the C standard library string utilities defined in the <string.h>
header file. It can be utilized to extract tokens surrounded by the given delimiter characters from the string object.
strsep
takes two arguments - pointer to char*
and pointer to char
. The first argument is used to pass the address of the character string that needs to be searched. The second parameter specifies a set of delimiter characters, which mark the beginning and end of the extracted tokens. Note that delimiter characters are discarded in the extracted token strings. When the first token is found, the first argument is modified to store the pointer to the next delimiter found.
In the following example, we demonstrate how to extract two tokens delimited with the given character using the single call to strsep
. Notice that the program takes the string and delimiter set from the 2 command line arguments and exits with failure if not supplied as needed. Next, we duplicate the string with the strdupa
function call as the strsep
modifies the passed pointer, and we don’t want to lose the original value. strdupa
allocates dynamic memory on stack, and the caller should not free the pointer returned from it.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
char *str1, *token;
if (argc != 3) {
fprintf(stderr, "Usage: %s string delim\n", argv[0]);
exit(EXIT_FAILURE);
}
str1 = strdupa(argv[1]);
if (!str1) exit(EXIT_FAILURE);
token = strsep(&str1, argv[2]);
if (token == NULL) exit(EXIT_FAILURE);
printf("extracted: '%s'\n", token);
printf("left: '%s'\n", str1);
exit(EXIT_SUCCESS);
}
Sample Command:
./program "hello there" t
Output:
extracted: 'hello '
left: 'here'
Alternatively, we can implement the for
loop that calls the strsep
function consecutively and extracts every token with the given delimiters rather than only the one encountered first - as seen in the previous example code. Note though, that strsep
returns an empty string when two delimiter characters are in a row; thus, the caller is responsible for checking this before processing the result tokens. Similar functionality with slight differences is also provided using strtok
and strtok_r
library functions described in detail on this page.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
char *str1, *token;
if (argc != 3) {
fprintf(stderr, "Usage: %s string delim\n", argv[0]);
exit(EXIT_FAILURE);
}
str1 = strdupa(argv[1]);
if (!str1) exit(EXIT_FAILURE);
for (int j = 1;; j++) {
token = strsep(&str1, argv[2]);
if (token == NULL) break;
printf("%d: '%s'\n", j, token);
}
exit(EXIT_SUCCESS);
}
Sample Command:
./program "hello there" tl
Output:
1: 'he'
2: ''
3: 'o '
4: 'here'
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook