How to Check if String Contains Substring in C
-
Use the
strstr
Function to Check if a String Contains a Substring in C -
Use the
strcasestr
Function to Check if a String Contains a Substring -
Use the
strncpy
Function to Copy a Substring
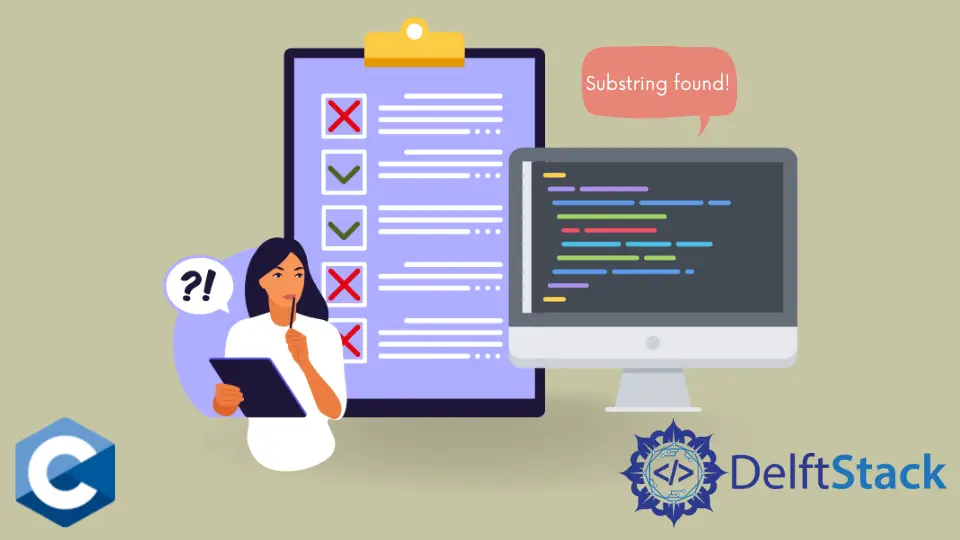
This article will explain several methods of how to check if a string contains a given substring in C.
Use the strstr
Function to Check if a String Contains a Substring in C
The strstr
function is part of the C standard library string facilities, and it’s defined in the <string.h>
header. The function takes two char
pointer arguments, the first denoting the string to search in and the other denoting the string to search for.
It finds the first starting address of the given substring and returns the corresponding pointer to char
. If the substring is not found in the first argument string, the NULL pointer is returned.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
const char *tmp = "This string literal is arbitrary";
int main(int argc, char *argv[]) {
char *ret;
ret = strstr(tmp, "literal");
if (ret)
printf("found substring at address %p\n", ret);
else
printf("no substring found!\n");
exit(EXIT_SUCCESS);
}
Output:
found substring at address 0x55edd2ecc014
Use the strcasestr
Function to Check if a String Contains a Substring
strcasestr
is not part of the standard library features, but it’s implemented as an extension in the GNU C library, which can be indicated with _GNU_SOURCE
macro definition.
Once defined, we can call the strcasestr
function to find the given substring’s first occurrence. Mind though, that this function ignores the case of both strings.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
const char *tmp = "This string literal is arbitrary";
int main(int argc, char *argv[]) {
char *ret;
ret = strcasestr(tmp, "LITERAL");
if (ret)
printf("found substring at address %p\n", ret);
else
printf("no substring found!\n");
exit(EXIT_SUCCESS);
}
Output:
found substring at address 0x55edd2ecc014
Use the strncpy
Function to Copy a Substring
Alternatively, one can copy the given substring to a new buffer using the strncpy
function. It takes three arguments, the first of which is the destination char
pointer where the copied substring will be stored.
The second argument is the source string, and the last argument denotes the first number of bytes to copy at most. Notice that if the null byte is not found in the source string’s first bytes, the destination string won’t be null-terminated.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
const char *tmp = "This string literal is arbitrary";
int main(int argc, char *argv[]) {
char *str = malloc(strlen(tmp));
printf("%s\n", strncpy(str, tmp, 4));
printf("%s\n", strncpy(str, tmp + 5, 10));
free(str);
exit(EXIT_SUCCESS);
}
Output:
This
string lit
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook