How to Use the strdup Function in C
-
Use the
strdup
Function to Duplicate the Given String in C -
Use the
strndup
Function to Duplicate the Given String in C -
Use the
strdupa
Function to Duplicate the Given String in C
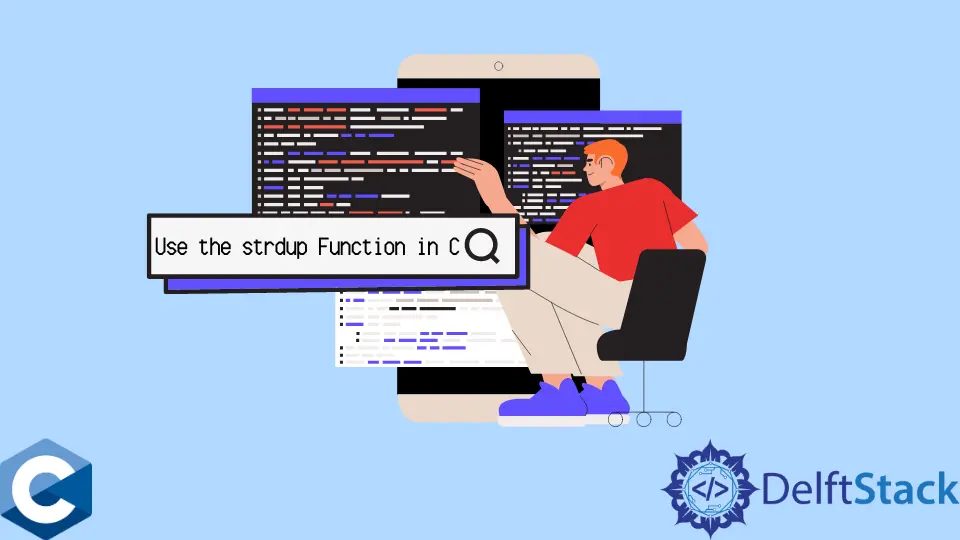
This article will explain several methods of how to use the strdup
function in C.
Use the strdup
Function to Duplicate the Given String in C
strdup
is one of the POSIX compliant functions that’s available on most UNIX based operating systems. It implements string copying functionality but does memory allocation and checking internally. Although a user is responsible for freeing the returned char
pointer since the strdup
allocates the memory with malloc
function call.
strdup
takes a single argument - the source string to be duplicated and returns the pointer to a newly copied string. The function returns NULL
on failure, namely when there’s an insufficient memory to allocate. In this case, we are retrieving the HOME
environment variable using the getenv
function and copy its value with strdup
.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
char *path = NULL;
const char *temp = getenv("HOME");
if (temp != NULL) {
path = strdup(temp);
if (path == NULL) {
perror("strdup");
exit(EXIT_FAILURE);
}
} else {
fprintf(stderr, "$HOME environment variable is not defined\n");
exit(EXIT_FAILURE);
}
printf("%s\n", path);
free(path);
exit(EXIT_SUCCESS);
}
Output:
/home/user
Use the strndup
Function to Duplicate the Given String in C
strndup
is a similar function that takes an additional argument to specify the number of bytes that need to be copied at most. This version is useful to copy only certain parts of the string. Note though, strndup
adds terminating null byte to the copied characters, thus, ensuring that it’s stored as C-style string format and can be manipulated as such.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
char *path = NULL;
const char *temp = getenv("HOME");
if (temp != NULL) {
path = strndup(temp, 5);
if (path == NULL) {
perror("strdup");
exit(EXIT_FAILURE);
}
} else {
fprintf(stderr, "$HOME environment variable is not defined\n");
exit(EXIT_FAILURE);
}
printf("%s\n", path);
free(path);
exit(EXIT_SUCCESS);
}
Output:
/home
Use the strdupa
Function to Duplicate the Given String in C
strdupa
is part of the GNU C library and may not be available in other C compilers. strdupa
is similar to the strdup
function except that it uses alloca
for memory allocation. The alloca
function implements memory allocation on the stack region, and the area is freed automatically when the calling function returns. Thus, the pointer returned from strdupa
should not be freed explicitly with the free
call as it will result in a segmentation fault. Note that _GNU_SOURCE
macro should be defined to compile the code successfully.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
char *path = NULL;
const char *temp = getenv("HOME");
if (temp != NULL) {
path = strdupa(temp);
if (path == NULL) {
perror("strdup");
exit(EXIT_FAILURE);
}
} else {
fprintf(stderr, "$HOME environment variable is not defined\n");
exit(EXIT_FAILURE);
}
printf("%s\n", path);
exit(EXIT_SUCCESS);
}
Output:
/home/user
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook