Static Variable in C
-
Use
static
Variable to Preserve Value Between Function Calls in C -
Use the
static
Keyword to Declare Variable Within the File Scope in C
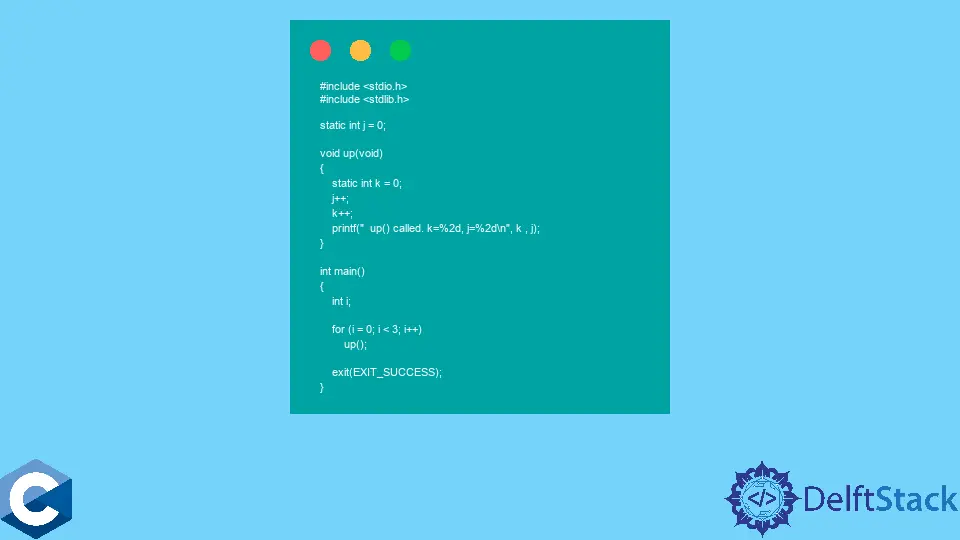
This article will demonstrate multiple methods about how to use static variables in C.
Use static
Variable to Preserve Value Between Function Calls in C
The static
keyword is used in multiple contexts, one of them is to declare the variable that keeps its value in memory between the function calls. This type of variable has a static storage duration.
In the following example, we declare a variable k
in a function block scope. When the control flow leaves the up
function, the value of k
persists in memory until the program exits. This behavior can be utilized to store data objects persistently during the program execution.
Note that static variables are automatically initialized to 0 if we do not initialize them explicitly.
#include <stdio.h>
#include <stdlib.h>
static int j = 0;
void up(void) {
static int k = 0;
j++;
k++;
printf(" up() called. k=%2d, j=%2d\n", k, j);
}
int main() {
int i;
for (i = 0; i < 3; i++) up();
exit(EXIT_SUCCESS);
}
Output:
up() called. k= 1, j= 1
up() called. k= 2, j= 2
up() called. k= 3, j= 3
Use the static
Keyword to Declare Variable Within the File Scope in C
The static
qualified variables can be declared outside any function, making them visible inside the single source file scope. Such variables are called static variables with internal linkage, meaning that their values can be used only by the function in the same file.
Notice that both functions have the local variable named k
initialized to zero, but when the down
function gets called, the variable k
(declared in up
) is not in the same scope, and a new one is initialized at the different memory location. Thus, the negative value is displayed after two iterations. Note that function parameters can’t have a static
qualifier.
#include <stdio.h>
#include <stdlib.h>
static int j = 0;
void up(void) {
static int k = 0;
j++;
k++;
printf(" up() called. k=%2d, j=%2d\n", k, j);
}
void down(void) {
static int k = 0;
j--;
k--;
printf("down() called. k=%2d, j=%2d\n", k, j);
}
int main() {
int i;
for (i = 0; i < 3; i++) up();
for (i = 0; i < 2; i++) down();
exit(EXIT_SUCCESS);
}
Output:
up() called. k= 1, j= 1
up() called. k= 2, j= 2
up() called. k= 3, j= 3
down() called. k=-1, j= 2
down() called. k=-2, j= 1
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook