The Round Function in C
-
Use the
round
Function to Round Floating-Point Number to the Nearest Integer and Return Floating-Point Number -
Use the
lround
Function to Round Floating-Point Number to the Nearest Integer and Return Integral Type -
Use the
ceil
Function to Round Floating-Point Number to the Smallest Integral Value Not Less Than Argument
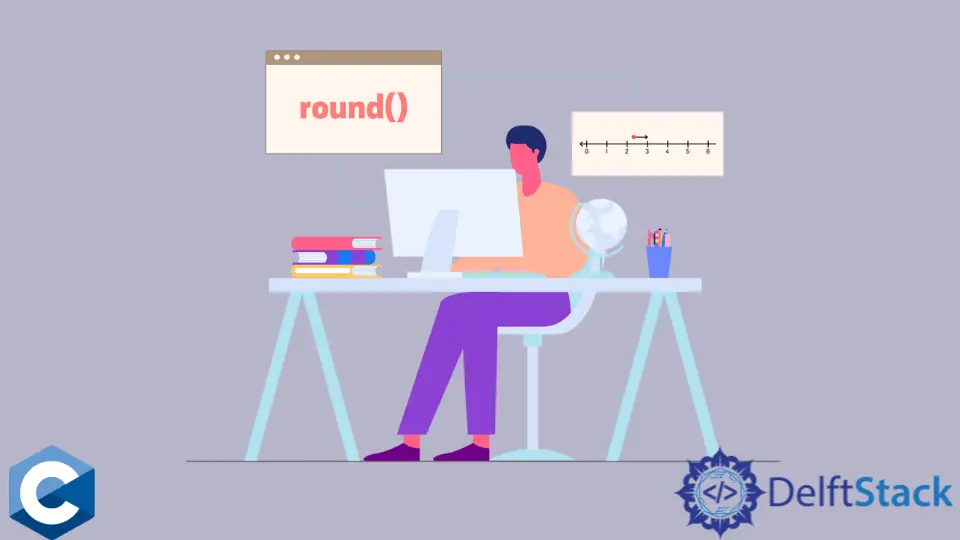
This article will explain several methods of how to use the round
function in C.
Use the round
Function to Round Floating-Point Number to the Nearest Integer and Return Floating-Point Number
The round
function is part of the C standard library math utilities defined in the <math.h>
header file. There are three functions in this family - round
, roundf
and roundl
. These functions are for different types of floating-point numbers, and each returns the corresponding type value. Note that source files, including the math
header, need to be compiled using the -lm
flag to link the library code. In the following example code, we demonstrate the conversions for multiple float
literal values and output the results to the console. Notice that round
essentially rounds away from the zero. If the integral value, -0
, +0
, NaN
or INFINITY
is passed as an argument, the same value is returned.
#include <fenv.h>
#include <math.h>
#include <stdio.h>
#include <stdlib.h>
int main(void) {
printf("round(+2.3) = %+.1f\n", round(2.3));
printf("round(+2.5) = %+.1f\n", round(2.5));
printf("round(+2.7) = %+.1f\n", round(2.7));
printf("round(-2.3) = %+.1f\n", round(-2.3));
printf("round(-2.5) = %+.1f\n", round(-2.5));
printf("round(-2.7) = %+.1f\n", round(-2.7));
exit(EXIT_SUCCESS);
}
Output:
round(+2.3) = +2.0
round(+2.5) = +3.0
round(+2.7) = +3.0
round(-2.3) = -2.0
round(-2.5) = -3.0
round(-2.7) = -3.0
Use the lround
Function to Round Floating-Point Number to the Nearest Integer and Return Integral Type
The lround
function, on the other hand, round to the nearest in integer and return the integral value. There are six functions in this family, the half of which returns long int
as rounded value and others - long long int
. lround
similar to the round
family rounds the halfway real numbers away from zero.
#include <fenv.h>
#include <math.h>
#include <stdio.h>
#include <stdlib.h>
int main(void) {
printf("lround(+2.3) = %ld\n", lround(2.3));
printf("lround(+2.5) = %ld\n", lround(2.5));
printf("lround(+2.7) = %ld\n", lround(2.7));
printf("lround(-2.3) = %ld\n", lround(-2.3));
printf("lround(-2.5) = %ld\n", lround(-2.5));
printf("lround(-2.7) = %ld\n", lround(-2.7));
exit(EXIT_SUCCESS);
}
Output:
lround(+2.3) = 2
lround(+2.5) = 3
lround(+2.7) = 3
lround(-2.3) = -2
lround(-2.5) = -3
lround(-2.7) = -3
Use the ceil
Function to Round Floating-Point Number to the Smallest Integral Value Not Less Than Argument
Alternatively, the ceil
function can be utilized to round the given floating-point number to the smallest integer value that is not less than the argument itself. Similar to the round
function, there are three functions in this family too - ceil
, ceilf
and ceill
to be used for float
, double
and long double
types, respectively. Note that we include the +
sign in the prinf
specifier string, and it automatically displays the corresponding signs for the rounded arguments.
#include <fenv.h>
#include <math.h>
#include <stdio.h>
#include <stdlib.h>
int main(void) {
printf("ceil(+2.3) = %+.1f\n", ceil(2.3));
printf("ceil(+2.5) = %+.1f\n", ceil(2.5));
printf("ceil(+2.7) = %+.1f\n", ceil(2.7));
printf("ceil(-2.3) = %+.1f\n", ceil(-2.3));
printf("ceil(-2.5) = %+.1f\n", ceil(-2.5));
printf("ceil(-2.7) = %+.1f\n", ceil(-2.7));
exit(EXIT_SUCCESS);
}
Output:
ceil(+2.3) = 3.000000
ceil(+2.5) = 3.000000
ceil(+2.7) = 3.000000
ceil(-2.3) = -2.000000
ceil(-2.5) = -2.000000
ceil(-2.7) = -2.000000
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook