How to Read a File in C
-
Use
fopen
andfread
Functions to Read Text File in C -
Use
fopen
andgetline
Functions to Read Text File in C
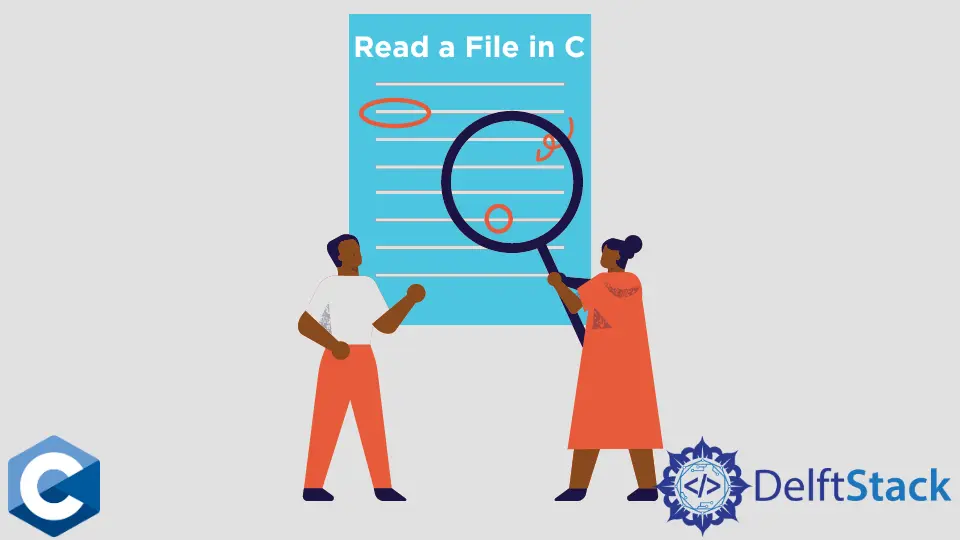
This article will introduce multiple methods about how to read a text file in C.
Use fopen
and fread
Functions to Read Text File in C
fopen
and fread
functions are part of the C standard library’s input/output facilities.
fopen
is used to open the given file as a stream and give the program the handle to manipulate it as needed. It takes two parameters, the name of the file as const char*
string and mode in which to open the file specified with predefined values (r
, w
, a
, r+
, w+
, a+
). When we need to read a file, we pass r
as the second parameter to open the file in read-only mode.
On the other hand, the fread
function is the main actor in conducting the read operation from an already opened file stream. It takes a pointer to buffer where the read bytes should be stored as the first argument. The second and third arguments specify how many items to read from the stream and what size does each of them has. The last argument is the FILE*
pointer to the opened file stream itself.
Notice that we are also using the stat
function to retrieve the file size and read the whole contents in a single fread
call. The file size is passed to the malloc
function as well for allocating enough space to store the whole file contents. Mind though, dynamic memory allocations should be freed by the free
function and open files closed with fclose
.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
int main(void) {
const char* filename = "input.txt";
FILE* input_file = fopen(filename, "r");
if (!input_file) exit(EXIT_FAILURE);
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
char* file_contents = malloc(sb.st_size);
fread(file_contents, sb.st_size, 1, input_file);
printf("%s\n", file_contents);
fclose(input_file);
free(file_contents);
exit(EXIT_SUCCESS);
}
Use fopen
and getline
Functions to Read Text File in C
Alternatively, we can skip retrieving the file size with the stat
function and instead iterate over each line of the file until the end is reached using the getline
function. getline
reads the string input from the given file stream. As the name suggests, the function retrieves all bytes until the newline character is found.
getline
takes three parameters, the first of which stores the read bytes. It can be declared as char*
and set to NULL
. Meanwhile, if the second argument is the address of integer 0
, the getline
automatically allocates dynamic memory for the buffer, which should be freed by the user, hence the call to the free
function at the end of the program.
Note that these parameters can have different values if needed, all of which are explained in this function manual. The third parameter is the FILE*
pointer of the open file stream.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
int main(void) {
const char* filename = "input.txt";
FILE* input_file = fopen(filename, "r");
if (!input_file) exit(EXIT_FAILURE);
char* contents = NULL;
size_t len = 0;
while (getline(&contents, &len, input_file) != -1) {
printf("%s", contents);
}
fclose(input_file);
free(contents);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook