How to Print Formatted Text in C
-
Use the
printf
Function With%s
Specifier to Print Strings -
Use the
printf
Function With%i
Specifier to Print Integers
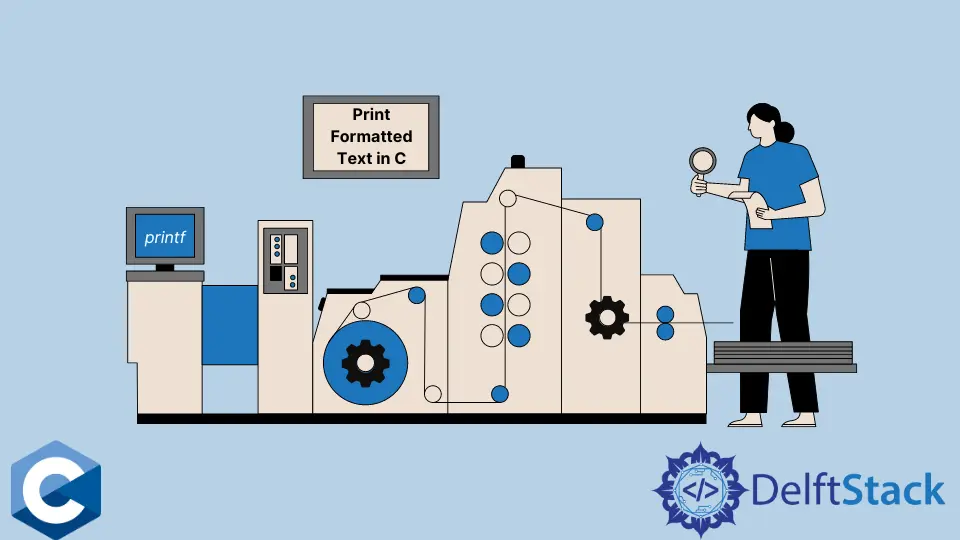
This article will introduce several methods of how to print formatted text to console in C.
Use the printf
Function With %s
Specifier to Print Strings
The printf
function is one of the most utilized parts of the standard input/output library. Actually, there is a whole family of printf
functions specialized for multiple scenarios, all of which are documented in great detail on this page. In this article, we only demonstrate the formatted output using the printf
function.
printf
is unique in that it can take a variable number of arguments. Namely, the function parameters can be divided into two parts, the format string and other arguments.
The format string specifies the formatting part of the function, and it includes ordinary characters and specifiers starting with the %
symbol.
The simplest forms are demonstrated in the following example, where printf
takes the string itself as the only argument in the first call, and the second call declares a single %s
specifier in the format string followed by the string variable argument.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
const char* str1 = "fabulae mirabiles";
printf("String literal\n");
printf("%s\n", str1);
exit(EXIT_SUCCESS);
}
Output:
String literal
fabulae mirabiles
Another useful feature of the format string is to specify how many characters to display from the string argument that is passed to function. The next example code demonstrates two solutions to this problem.
The first puts the integer denoting the number of characters between the %
and s
symbols; thus, only 6 characters are printed from the given string argument.
The second printf
call puts *
character instead and lets the user pass the integral value from one of the arguments. The plus side of the latter method is that value can be calculated at run-time, while the former method requires the value to be hard-coded. Notice that, in both cases, the .
after the %
symbol is necessary.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
const char* str1 = "fabulae mirabiles";
printf("%.6s\n", str1);
printf("%.*s \n", 6, str1);
exit(EXIT_SUCCESS);
}
Output:
fabula
fabula
Use the printf
Function With %i
Specifier to Print Integers
printf
can print integers with different representations. Common methods include modifying the base in which the integer numbers are displayed.
Integer arguments can be denoted with %i
or %d
specifier. The positive and negative numbers are automatically formatted, except that positive numbers are not displayed with the plus sign, and it can be included with explicit specifier %+i
.
Hexadecimal and octal numbers can be outputted with %x
and %o
specifiers correspondingly. %X
specifier displays hexadecimal numbers with capital letter formatting.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
printf("%i %d %.6i %+i %i\n", 11, 22, 12, 41, -31);
printf("%.4x %x %X %#x\n", 126, 125, 125, 100);
printf("%.4o %o\n", 8, 11);
exit(EXIT_SUCCESS);
}
Output:
11 22 000012 +41 -31
007e 7d 7D 0x64
0010 13
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook