i++ vs ++i in C
-
Main Differences Between
++i
andi++
Notations in C -
Use
++i
Notation as Commonly Accepted Style for Loop Statements in C
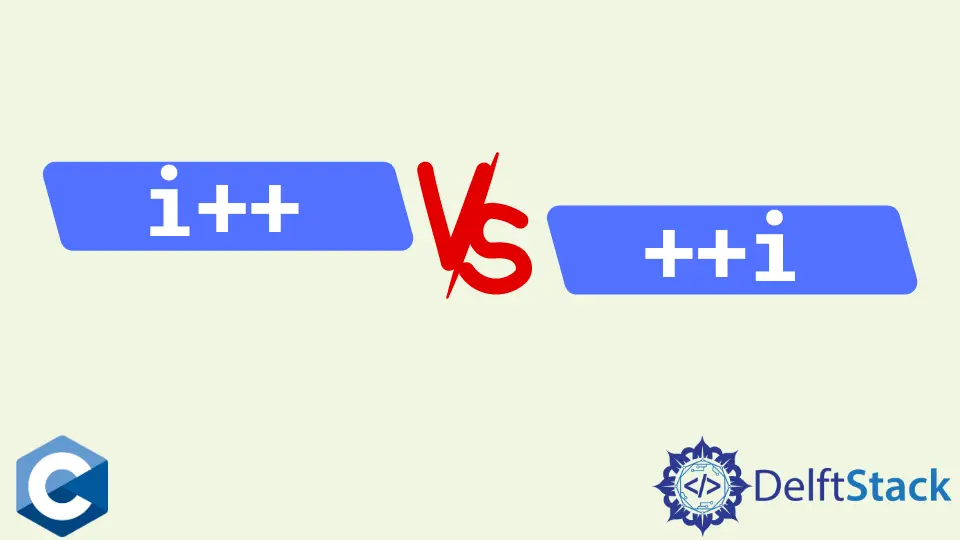
This article will explain several methods of using prefix increment vs postfix increment operators, aka, i++
vs ++i
, in C.
Main Differences Between ++i
and i++
Notations in C
The fundamental part of these two notations is the increment unary operator ++
that increases its operand, e.g. i
, by 1. The increment operator may come before the operand as prefix ++i
or after the operand as postfix operator - i++
.
Slightly unusual behavior arises when the variable value incremented with the ++
operator is used in an expression. In this case, prefix and postfix increments behave differently.
Namely, the prefix operator increments its operand before its value is used, whereas the postfix operator increments the operand after the value has been used.
As a result, the printf
function that uses the i++
expression as its argument will print the value of i
before it gets incremented by one.
On the other hand, printf
with prefix increment operator ++i
will print the incremented value, which will be 1 on the first iteration as demonstrated in the following example code.
#include <stdio.h>
#include <stdlib.h>
int main() {
int i = 0, j = 0;
while ((i < 5) && (j < 5)) {
/*
postfix increment, i++
the value of i is read and then incremented
*/
printf("i: %d\t", i++);
/*
prefix increment, ++j
the value of j is incremented and then read
*/
printf("j: %d\n", ++j);
}
printf("At the end they have both equal values:\ni: %d\tj: %d\n\n", i, j);
exit(EXIT_SUCCESS);
}
Output:
i: 0 j: 1
i: 1 j: 2
i: 2 j: 3
i: 3 j: 4
i: 4 j: 5
At the end they have both equal values:
i: 5 j: 5
Use ++i
Notation as Commonly Accepted Style for Loop Statements in C
Postfix and prefix operators have the same functional behavior when used in the for
loop statement. As the two for
iterations are executed in the sample code below, they print the values of k
identically.
Note that there are several claims about the better performance efficiency of using prefix increment over postfix in the for
loops, but effective time difference in most applications will be negligible. We can get into the habit of using the prefix operator as the preferred method as a commonly accepted style.
But if you are already using postfix notation, then consider that using modern compilers with corresponding optimization flags will automatically eliminate inefficient loop iterations.
#include <stdio.h>
#include <stdlib.h>
int main() {
for (int k = 0; k < 5; ++k) {
printf("%d ", k);
}
printf("\n");
for (int k = 0; k < 5; k++) {
printf("%d ", k);
}
printf("\n\n");
exit(EXIT_SUCCESS);
}
Output:
0 1 2 3 4
0 1 2 3 4
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook