How to Plot Data in C
- Using gnuplot to Plot Data in C
- Using PLplot Library for Data Visualization
- Using Cairo Graphics for Custom Plotting
- Conclusion
- FAQ
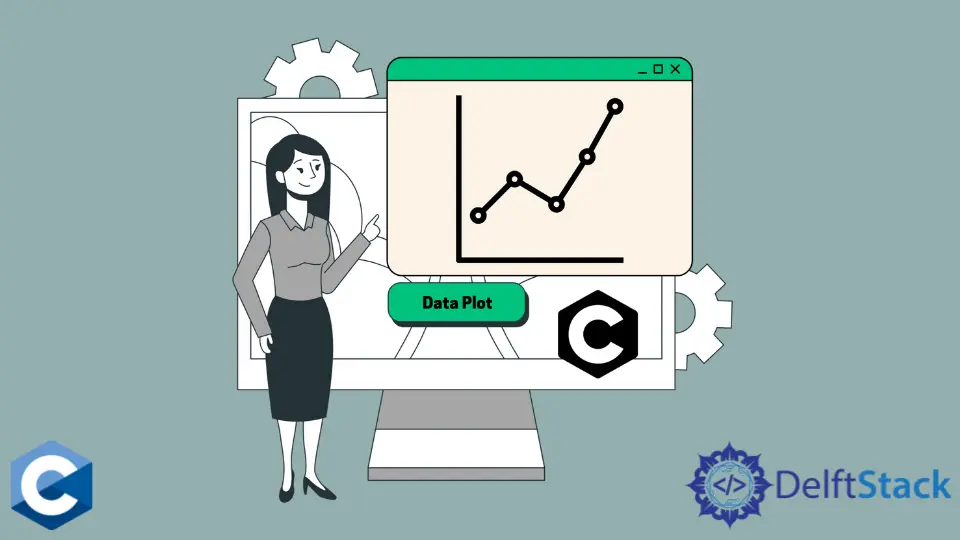
Data visualization is a powerful tool that can enhance your understanding of complex datasets. When it comes to programming languages, C is often overlooked for plotting, but it has its own set of libraries that can help you create effective visualizations.
In this article, we will explore various methods to plot data in C, focusing on libraries and techniques that are both efficient and easy to use. Whether you’re a seasoned programmer or just starting out, this guide will provide you with the knowledge you need to represent your data visually in the C programming language.
Using gnuplot to Plot Data in C
One of the most popular ways to plot data in C is by leveraging the capabilities of gnuplot. Gnuplot is a portable command-line driven graphing utility that allows you to create 2D and 3D plots easily. To use gnuplot, you’ll typically generate data files in your C program and then call gnuplot to visualize them. Below is a simple example of how you can do this.
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *fp;
fp = fopen("data.txt", "w");
for (int i = 0; i < 100; i++) {
fprintf(fp, "%d %f\n", i, sin(i * 0.1));
}
fclose(fp);
system("gnuplot -e \"set terminal png; set output 'plot.png'; plot 'data.txt' with lines\"");
return 0;
}
Output:
A PNG file named plot.png will be created in your working directory.
In this example, we first create a file named data.txt
and write the sine values of integers from 0 to 99 into it. Then, we use the system
function to call gnuplot, which generates a PNG image of the plot. The command passed to gnuplot specifies the terminal type, output file, and the data file to plot. This method is straightforward and allows for a variety of customization options in gnuplot.
Using PLplot Library for Data Visualization
Another effective way to plot data in C is by using the PLplot library. PLplot is a cross-platform library for creating scientific plots and provides a wide range of plotting capabilities. To get started, you need to install the PLplot library and link it to your C project.
Here’s a simple example of how to use PLplot to plot a sine wave:
#include <plplot/plplot.h>
#include <math.h>
int main() {
PLFLT x[100], y[100];
for (int i = 0; i < 100; i++) {
x[i] = i * 0.1;
y[i] = sin(x[i]);
}
plinit();
plenv(0, 10, -1, 1, 0, 0);
plline(100, x, y);
plend();
return 0;
}
In this code, we first initialize two arrays: x
for the x-coordinates and y
for the sine values. The plinit
function initializes the PLplot library, while plenv
sets up the plot environment. The plline
function is then used to draw the line plot based on the x and y data. Finally, plend
concludes the plotting process. This method is particularly useful for creating high-quality scientific plots.
Using Cairo Graphics for Custom Plotting
Cairo is another powerful library that can be used for 2D graphics in C. It provides a rich set of features for drawing shapes, text, and images, making it a versatile choice for custom data visualizations. Below is an example of how to create a simple line plot using Cairo.
#include <cairo.h>
#include <math.h>
int main() {
cairo_surface_t *surface;
cairo_t *cr;
surface = cairo_image_surface_create(CAIRO_FORMAT_ARGB32, 600, 400);
cr = cairo_create(surface);
cairo_set_line_width(cr, 2);
cairo_move_to(cr, 0, 200);
for (int i = 0; i < 600; i++) {
cairo_line_to(cr, i, 200 - (100 * sin(i * 0.01)));
}
cairo_stroke(cr);
cairo_surface_write_to_png(surface, "cairo_plot.png");
cairo_destroy(cr);
cairo_surface_destroy(surface);
return 0;
}
Output:
A PNG file named cairo_plot.png will be created in your working directory.
In this example, we create a PNG surface of size 600 by 400 pixels. We then set the line width and start drawing from the left side of the surface. The cairo_line_to
function is used to create a line plot of the sine function. Finally, we save the surface as a PNG file. Cairo is particularly useful for custom graphics, allowing you to create plots that are tailored to your specific needs.
Conclusion
Plotting data in C may seem daunting at first, but with the right tools and libraries, it can be a straightforward process. Whether you choose to use gnuplot, PLplot, or Cairo, each method offers unique advantages that can help you visualize your data effectively. By understanding these techniques, you can enhance your data analysis and presentation skills, making your insights more accessible and impactful.
FAQ
-
What is the best library for plotting in C?
It depends on your specific needs. Gnuplot is great for quick plots, PLplot is excellent for scientific graphs, and Cairo offers custom graphics capabilities. -
Do I need to install any libraries to plot data in C?
Yes, you may need to install libraries like gnuplot, PLplot, or Cairo, depending on the method you choose. -
Can I plot 3D data in C?
Yes, both gnuplot and PLplot support 3D plotting. -
Is it possible to create interactive plots in C?
While C is not primarily designed for interactive graphics, using libraries like GTK with Cairo can help you create interactive applications. -
How can I improve the appearance of my plots?
Most plotting libraries offer options to customize colors, labels, and styles, allowing you to enhance the visual appeal of your plots.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook