How to Create a New Directory in C
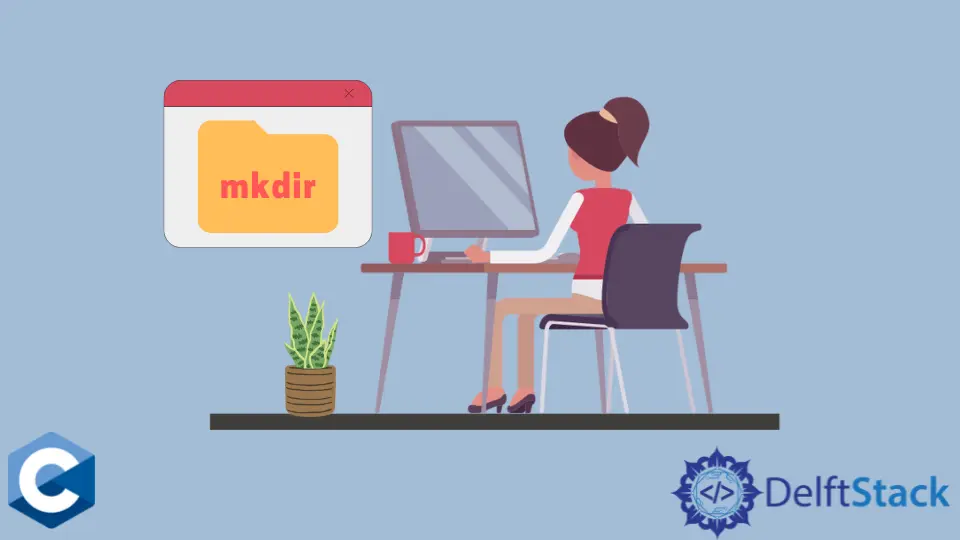
Creating a new directory in C can be a straightforward task, yet it’s essential for anyone looking to manage files effectively in their applications. Whether you’re developing a simple program or a complex system, knowing how to create directories can help organize your files and improve your software’s functionality.
In this article, we’ll explore the methods to create a new directory using C programming. We’ll provide clear examples and explanations to ensure you understand each step of the process. By the end of this guide, you’ll be equipped with the knowledge you need to create directories seamlessly in your C applications.
Using the mkdir Function
One of the most common ways to create a new directory in C is by using the mkdir
function, which is included in the <sys/stat.h>
header file. This function allows you to specify the name of the directory you want to create and set the permissions for that directory. Here’s how you can use it.
#include <stdio.h>
#include <sys/stat.h>
int main() {
const char *dir_name = "new_directory";
int status = mkdir(dir_name, 0755);
if (status == 0) {
printf("Directory created successfully.\n");
} else {
perror("Error creating directory");
}
return 0;
}
Output:
Directory created successfully.
In this code, we first include the necessary headers. The mkdir
function takes two arguments: the name of the directory and the permissions. The permissions are set using octal notation, where 0755
allows the owner to read, write, and execute, while others can only read and execute. If the directory creation is successful, a success message is printed; otherwise, an error message will display the reason for the failure. This method is straightforward and effective for creating directories in C.
Using the system Function
Another way to create a directory in C is by using the system
function, which allows you to execute shell commands directly from your C program. This can be particularly useful if you want to leverage existing shell commands for directory creation.
#include <stdio.h>
#include <stdlib.h>
int main() {
const char *dir_name = "new_directory";
char command[50];
snprintf(command, sizeof(command), "mkdir %s", dir_name);
int status = system(command);
if (status == 0) {
printf("Directory created successfully.\n");
} else {
printf("Error creating directory.\n");
}
return 0;
}
Output:
Directory created successfully.
In this example, we construct a shell command using snprintf
to safely format the string. The system
function is then called with this command. If the command executes successfully, a success message is printed; otherwise, an error message is displayed. This method is flexible, allowing you to use any shell command, but it may not be as portable as using the mkdir
function directly.
Checking Directory Existence
Before creating a new directory, it’s often a good idea to check if it already exists. This can prevent errors and ensure that your program runs smoothly. Here’s how you can do this in C.
#include <stdio.h>
#include <sys/stat.h>
#include <string.h>
int main() {
const char *dir_name = "new_directory";
struct stat statbuf;
if (stat(dir_name, &statbuf) == 0) {
printf("Directory already exists.\n");
} else {
if (mkdir(dir_name, 0755) == 0) {
printf("Directory created successfully.\n");
} else {
perror("Error creating directory");
}
}
return 0;
}
Output:
Directory created successfully.
In this code, we use the stat
function to check if the directory already exists. If it does, a message is printed. If it doesn’t, we proceed to create the directory using mkdir
. This approach enhances the robustness of your application by handling potential errors gracefully. It’s a good practice to check for existing directories, especially in larger applications where file management is crucial.
Conclusion
Creating a new directory in C is essential for effective file management in your applications. Whether you choose to use the mkdir
function, the system
function, or check for existing directories beforehand, each method has its advantages. By following the examples and explanations provided in this article, you can confidently implement directory creation in your C programs. This knowledge will not only enhance your coding skills but also improve the overall functionality of your applications.
FAQ
-
What header files are required to create a directory in C?
You need to include<stdio.h>
and<sys/stat.h>
for using themkdir
function. -
Can I specify permissions when creating a directory in C?
Yes, themkdir
function allows you to specify permissions using octal notation. -
What happens if I try to create a directory that already exists?
If the directory already exists, themkdir
function will return an error, which you can handle in your code. -
Is using the system function for creating directories portable?
While it works, using thesystem
function may not be as portable as directly usingmkdir
, as it depends on the underlying shell. -
How can I check if a directory exists before creating it?
You can use thestat
function to check if a directory exists by examining its status.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook